‘0’ in C++ with operators ‘- ‘ and ‘+ ‘ facilitate character-to-integer and integer-to-character conversion, respectively. Leveraging ASCII encoding, they convert character digits to integers and vice versa for efficient numeric manipulation in C++ programming.
‘0’ in C++ is very essential to learn as a C++ programmer. You’ll understand the subtle details and advanced techniques of the language are critical for mastery. One example that often confuses beginners is the use of -‘0′ and +’0’ when manipulating digit characters and integers. Since C++ stores characters and integers differently, conversions are necessary.
Our Physics Wallah’s C++ with DSA Course offers an in-depth look at concepts like this to take your skills to an expert level. From basic to advanced, we’ll explain that character digits have ASCII values from 48 for ‘0’ to 57 for ‘9’. By subtracting ‘0’, you can convert a digit character to its integer value. Adding back ‘0’ reverses this to convert integers to digit characters. Mastering numeric manipulation at this lower level allows you to become a proficient C++ programmer.
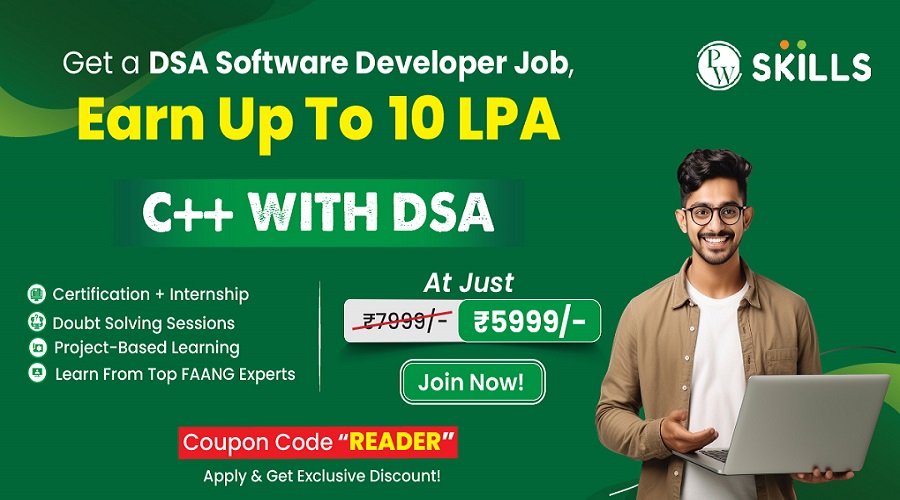
With expert instructors and a comprehensive curriculum, our C++ course gives you the tools needed to leverage the full power of this versatile language in your development career. Moreover, the ice on the cake for our regular readers is you can apply READER coupon code to get an exclusive discount and get certified as a ‘0’ in C++ programming.Â
0 in C++ Definition
In C++, the symbol ‘0’ plays a crucial role in character-to-integer and integer-to-character conversions using the ‘-‘ and ‘+’ operators. These operators, when applied to ‘0’, leverage ASCII encoding to manipulate digit characters and integers. Understanding this concept is fundamental for C++ programmers, as characters and integers are stored differently. For instance, subtracting ‘0’ converts a digit character to its integer value, and adding ‘0’ reverses the process, of converting integers to digit characters.Â
This lower-level numeric manipulation is essential for mastery of C++ programming. The ASCII values for character digits range from 48 for ‘0’ to 57 for ‘9’. Gaining proficiency in these conversions empowers programmers to navigate the intricacies of C++ development effectively.
0 in C++ example
In C++, the integer literal 0 represents the integer zero. It’s a fundamental concept in programming and has various uses. Below is an example demonstrating the usage of 0 in C++, along with explanations:
#include <iostream>
int main() {
    int number = 0; // Assigning the value 0 to an integer variable
    std::cout << “The value of number is: ” << number << std::endl;
    // Conditional statements involving 0
    if (number == 0) {
        std::cout << “The number is zero.” << std::endl;
    } else {
        std::cout << “The number is not zero.” << std::endl;
    }
    // Arithmetic operations involving 0
    int result = 10 * number; // Multiplying any number by 0 yields 0
    std::cout << “Multiplying 10 by zero gives: ” << result << std::endl;
    // Division involving 0
    // Note: Division by zero is undefined behavior and should be avoided
    int division_result = 10 / number; // Division by zero would result in a runtime error
    std::cout << “Dividing 10 by zero gives: ” << division_result << std::endl;
    return 0;
}
Explanation
int number = 0;: This line declares an integer variable named number and initializes it with the value 0.
if (number == 0) { … }: This is a conditional statement that checks if the value of the number is equal to 0. If it is, the code inside the if block executes, indicating that the number is zero.
int result = 10 * number;: This line performs multiplication of 10 with the value stored in number. Since the number is 0, the result will be 0.
int division_result = 10 / number;: Here, division of 10 by the value stored in the number is attempted. However, since the number is 0, division by zero is attempted, which results in undefined behavior. In practice, this often leads to a runtime error.
The usage of 0 in C++ is not limited to the examples provided here. It is a fundamental value used in various contexts, such as initializing variables, checking conditions, arithmetic operations, and more.
Also Read: C++ Classes and Objects: Exercises, Examples
0 in C++ array
In C++, the value 0 plays an important role in array initialization, comparison, and termination. Here’s a detailed explanation of how 0 is used with arrays in C++:
- Array Initialization
- In C++, arrays can be initialized with the value 0 to set all elements to zero. This is particularly useful when creating arrays of primitive types like integers, floats, or pointers.
- If an array is initialized with fewer values than its size, the remaining elements are automatically initialized to zero.
Example:
int array[5] = {0}; // Initializes all elements of the array to 0
- Comparing Array Elements with 0
- In C++, you can compare individual elements of an array with 0 to check if they are zero.
- This is commonly used in loop iterations or when processing array elements.
Example:
int array[5] = {1, 0, 3, 0, 5};
for (int i = 0; i < 5; ++i) {
    if (array[i] == 0) {
        std::cout << “Element at index ” << i << ” is zero.” << std::endl;
    }
}
- Terminating Arrays with 0
- In C-style strings (arrays of characters), the null character ‘\0’, which has an ASCII value of 0, is used to denote the end of the string.
- This allows functions like strlen() to determine the length of the string by iterating until it encounters the null character.
Example:
char str[] = “Hello”; // Compiler automatically appends ‘\0’ at the end
std::cout << “Length of str: ” << strlen(str) << std::endl; // Outputs: 5
- Using 0 as a Sentinel Value
- In some scenarios, 0 is used as a sentinel value to indicate the end of data input or processing.
- For example, when reading input until the user enters 0, or when parsing a list of integers terminated by 0.
Example:
int numbers[100]; // Assuming maximum of 100 numbers
int count = 0;
int num;
std::cout << “Enter numbers (terminate with 0): “;
while (std::cin >> num && num != 0 && count < 100) {
    numbers[count++] = num;
}
Overall, 0 in C++ arrays serves multiple purposes, including initialization, comparison, termination, and sentinel value, making it a versatile and commonly used constant in array-related operations.
0 in C
In C programming, the integer literal 0 plays several important roles and has various uses. Below are detailed explanations along with examples:
- Initialization and Assignment
- In C, 0 is frequently used for initializing variables, especially integers, pointers, and arrays.
Example:
int x = 0; // Initializing an integer variable
char *ptr = NULL; // Initializing a pointer to NULL (equivalent to 0)
int array[5] = {0}; // Initializing all elements of an array to 0
- Null Pointer:
- In C, 0 is commonly used to represent a null pointer, which points to no memory location.
- It’s a portable way to initialize pointers or compare them to check for nullness.
Example:
char *ptr = NULL; // Initializing a pointer to NULL
if (ptr == 0) {
    printf(“Pointer is NULL.\n”);
}
- Conditional Statements
- 0 is frequently used in conditional statements to check for zero values or false conditions.
Example:
int num = 5;
if (num == 0) {
    printf(“Number is zero.\n”);
} else {
    printf(“Number is not zero.\n”);
}
- Function Return Values:
- In C, a function returning an integer often uses 0 to indicate success or a non-error condition.
Example:
int add(int a, int b) {
    return a + b;
}
int main() {
    int result = add(3, 4);
    if (result == 0) {
        printf(“Addition successful.\n”);
    } else {
        printf(“Error in addition.\n”);
    }
    return 0;
}
Termination Conditions:
- In loops or functions, 0 is often used as a termination condition, especially when iterating through arrays or processing data.
Example:
int numbers[] = {1, 2, 3, 4, 0}; // Using 0 as a sentinel value
int sum = 0;
for (int i = 0; numbers[i] != 0; i++) {
    sum += numbers[i];
}
String Termination:
- In C-style strings, the null character ‘\0’, which has a value of 0, is used to terminate the string.
Example:
char str[] = “Hello”; // Compiler automatically appends ‘\0’ at the end
These are some of the common uses of 0 in C programming. Understanding its various roles is essential for writing efficient and correct C code.
Also Read: Virtual Function in C++: Everything You Need to Know
Function = 0 in C++
In C++, the concept of a function being equal to 0 typically refers to function pointers or the return value of functions. Let’s explore both cases in detail:
- Function Pointers
In C++, functions can be passed as arguments to other functions or stored in variables known as function pointers. A function pointer can be initialized to 0 (or nullptr in modern C++) to indicate that it does not currently point to any function.
Example:
#include <iostream>
// Function to be assigned to the function pointer
void myFunction() {
    std::cout << “Inside myFunction” << std::endl;
}
int main() {
    void (*funcPtr)() = nullptr; // Function pointer initialized to nullptr
    funcPtr = &myFunction; // Assigning a function to the function pointer
    if (funcPtr == nullptr) {
        std::cout << “Function pointer is currently null.” << std::endl;
    } else {
        std::cout << “Function pointer is not null.” << std::endl;
        // Calling the function through the function pointer
        funcPtr();
    }
    return 0;
}
In the example above, funcPtr is initialized to nullptr, indicating that it currently doesn’t point to any function. Later, it is assigned the address of the myFunction, and then the function is called through the function pointer.
- Return Value of a Function
A function in C++ can also return a value of 0 to indicate a successful execution or a default value. This is particularly common in functions that return integers, where 0 can signify success, or in functions returning pointers, where nullptr (which is equivalent to 0) may denote failure or absence of valid data.
Example:
#include <iostream>
// Function returning 0 to indicate success
int myFunction() {
    // Perform some operations
    return 0; // Success
}
int main() {
    int result = myFunction();
    if (result == 0) {
        std::cout << “Function executed successfully.” << std::endl;
    } else {
        std::cout << “Function encountered an error.” << std::endl;
    }
    return 0;
}
In this example, myFunction() returns 0 to indicate that it executed successfully. The return value is then checked in main() to determine the outcome of the function call.
In short, in C++, a function being equal to 0 can refer to either a function pointer initialized to nullptr or a return value of 0 indicating success or a default value. Understanding these concepts is crucial for writing robust and efficient C++ code.
Also Read: C++ vs Java: 10 Key Difference Between C++ and Java
null vs 0 C++
In C++, nullptr and 0 (or the integer literal 0) are both used to represent null or absence of a value, but they have different meanings and usage contexts. Let’s delve into the details of nullptr and 0 in C++:
nullptr:
- nullptr is a keyword introduced in C++11 to explicitly denote a null pointer.
- It serves as a safer and more type-safe alternative to using integer literals like 0 or macros like NULL for null pointers.
- It can be assigned to any pointer type and is implicitly convertible to any pointer type.
- It has its distinct type std::nullptr_t.
- Using nullptr helps prevent potential bugs caused by mistakenly treating null pointers as integers or vice versa.
Example:
int* ptr = nullptr; // Assigning nullptr to a pointer
0 (or Integer Literal 0)
- In C++, the integer literal 0 is often used to represent null pointers, particularly in older codebases or when nullptr is not available (e.g., in pre-C++11 code).
- It is commonly used to initialize pointers or to compare against pointers to check for nullness.
- While widely used, using 0 for null pointers can sometimes lead to ambiguity or errors, especially in contexts where integer arithmetic is involved.
- In modern C++, it’s generally recommended to use nullptr instead of 0 for null pointers.
Example:
int* ptr = 0; // Assigning 0 to a pointer (older style)
The Key Differences
- Type Safety: nullptr is a specific type (std::nullptr_t), whereas 0 is an integer literal. Using nullptr helps enforce type safety and prevents unintended conversions.
- Ambiguity: Using 0 for null pointers can sometimes lead to ambiguity in overloaded functions or templates where an integer argument might be expected. nullptr helps avoid such ambiguities.
- Modern Best Practices: In modern C++ codebases (post-C++11), nullptr is generally preferred over 0 for null pointers due to its explicitness and type safety.
In short, while both nullptr and 0 can represent null or the absence of a value in C++, nullptr is the preferred choice for null pointers in modern C++ code due to its type safety and explicitness. However, 0 is still commonly used in legacy codebases or in contexts where nullptr is not available.
Also Read: Top 50 C++ Project Ideas For Beginners & Advanced
‘\0’ meaning in c
In C programming, ‘\0’ is a special character constant known as the null character or null terminator. It has a value of 0 in ASCII encoding and is used to terminate strings in C-style strings. Here’s a detailed explanation of ‘\0’ in C:
- Representation and Value:
- ‘\0’ is represented as a single character enclosed within single quotes. It’s important to note that it consists of a backslash followed by the digit 0.
- The ASCII value of ‘\0’ is 0, which is equivalent to an integer value of 0.
- It’s not visible when printed, but it serves as a special marker to denote the end of a string.
- String Termination:
- In C, strings are represented as arrays of characters terminated by ‘\0’. This null character indicates the end of the string.
- When a sequence of characters is enclosed in double quotes ” “, the compiler automatically appends a ‘\0’ at the end to signify the termination of the string.
- Functions that operate on strings, such as strlen(), strcpy(), strcmp(), etc., rely on the presence of ‘\0’ to determine the length or to perform operations on strings.
- Example Usage:
#include <stdio.h>
int main() {
    char str[] = “Hello”; // Compiler appends ‘\0’ automatically
    printf(“Length of str: %d\n”, strlen(str)); // Outputs: 5
    return 0;
}
In this example, the string “Hello” is stored in the str array, and the null character ‘\0’ is automatically added by the compiler to mark the end of the string. The strlen() function calculates the length of the string until it encounters the null character.
- Terminating Input:
- ‘\0’ can also be used to terminate input in certain contexts, such as when reading characters until a null character is encountered.
- This can be useful in scenarios where input is processed character by character until the end of input is reached.
‘\0’ is a fundamental aspect of C-style strings and plays a crucial role in string manipulation and processing. Understanding its usage is essential for effective string handling in C programming.
Understanding the nuances of 0 in C and C++ programming is essential for mastering these languages. From its role in representing null pointers to its significance in character-to-integer and integer-to-character conversions, 0 serves multiple purposes and is deeply ingrained in the fundamental operations of both languages. Additionally, leveraging – ‘0’ and + ‘0’ operators for efficient numeric manipulation underscores the importance of understanding ASCII encoding and character representations.
For those seeking to deepen their understanding of C++ and elevate their programming skills to an expert level, our Physics Wallah’s C++ with DSA Course offers comprehensive coverage of topics like this. Our course not only explores the subtleties of C++ programming but also provides insights into advanced techniques and best practices, including character-digit conversions and low-level numeric manipulation. With expert instructors and a structured curriculum, learners can gain proficiency in C++ programming and develop the skills necessary to excel in their development careers.
For Latest Tech Related Information, Join Our Official Free Telegram Group : PW Skills Telegram Group
0 in C++ FAQs
What is the significance of '0' in C++ programming?
'0' in C++ plays a crucial role in character-to-integer and integer-to-character conversions using the '-' and '+' operators, respectively. These conversions are essential for manipulating digit characters and integers efficiently.
How does '0' facilitate character-to-integer conversion in C++?
By leveraging ASCII encoding, subtracting '0' from a digit character converts it to its corresponding integer value. For example, '5' - '0' results in the integer 5.
In what scenarios is '0' commonly used in C++ arrays?
'0' is frequently used in array initialization, comparison, and termination. It initializes all elements of an array to 0, compares individual elements to check for zero values, and terminates C-style strings with the null character '\0'.
Why is nullptr preferred over '0' for null pointers in modern C++ code?
nullptr is a type-safe alternative introduced in C++11, explicitly denoting a null pointer. Unlike '0', which is an integer literal, nullptr helps prevent unintended conversions and ambiguities in overloaded functions or templates, making code more robust and readable.
Can '0' be used as a sentinel value in C++?
Yes, '0' can be used as a sentinel value to indicate the end of data input or processing in certain scenarios, such as reading input until the user enters '0' or parsing a list of integers terminated by '0'. This usage helps streamline input processing and termination conditions in loops or functions.