Function pointer in C is a variable which holds the address of a function. The function pointers point to executable code inside a program, unlike regular pointers in C, which point to data. The function pointers are used to get the address of the function. Let us consider an example.
Function Pointer in C |
void fun (int a )
{ printf(“ Rahul got %d marks in maths.\n”,mks); } int main () {
void (*ptr1) (int) = fun; ptr1(20); return 0; } |
In the above function, the fun pointer is a pointer pointing to a function name fun, which will return an integer value at the end of execution.
Start your programming career with the C programming foundation. Let us learn some significant information in this article related to the function pointers in C.
Declaration of a Function Pointer in C
The function pointers contain the address of the function, which we can access using pointers.
Function Pointer Syntax in C |
return data_type (*pointer_name) (parameter_type); |
Let us take an example to understand the declaration of function pointers in C.
Function Pointer in C |
int add (int a, int b) {
return a + b; } int main () { int (*functionPtr)(int, int); functionPtr = add; int result = functionPtr (2,3); printf (“Result: %d\n”, result); |
Output:
Function Pointer in C |
Result: 5 |
Here, in the above example, we declare a ‘functionPtr’ that takes two parameters and returns one. We then assign the address of the function ‘add’ to the pointer and then call the add function by using the pointer directly.
Function Pointers in C Important Points
Let us now check some important facts related to function pointers in C.
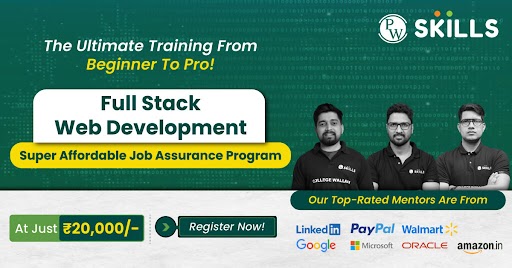
- Function pointers are used to point to code, unlike regular pointers, which point to data.
- With the help of function pointers, we can get the function address using only the function’s name.
Function Pointers in C |
void fun (int a )
{ printf(“ Rahul got %d marks in maths.\n”,mks); } int main () {
void (*ptr1) (int) = fun; ptr1(20); return 0; } |
- We can use function pointers in switch case. Check the examples above where we use a switch case based on the value of operation.
Function Pointer in C using Arrays
We can have an array of function pointers in C. Let us check an example of function pointers using an array. The syntax for the function pointer array is
Function Pointer in C |
return _type (*functionPtr [SIZE]) (parameter_type) |
Also read: Linear Search Algorithm in C
Let us take an example to understand the array of function pointers in C.
Function Pointer in C |
#include <stdio.h>
int add(int a, int b) { return a + b; } int subtract(int a, int b) { return a – b; } int multiply(int a, int b) { return a * b; } int main() { // Declare an array of function pointers int (*functionPtrArray[3])(int, int) = {add, subtract, multiply}; // Call functions using function pointers int result1 = functionPtrArray[0](3, 5); int result2 = functionPtrArray[1](8, 2); int result3 = functionPtrArray[2](4, 6); printf(“Result 1: %d\n”, result1); printf(“Result 2: %d\n”, result2); printf(“Result 3: %d\n”, result3); return 0; } |
Also read: Keywords and Identifiers in C
Output:
Function Pointers in C |
Result1: 8
Result2: 6 Result3: 24 |
Function Pointer in C using Pointer Variable
We can pass pointers as function arguments in C and can return the pointers from the function. We can pass a reference to the value stored instead of passing the value stored inside the variable. This is called pass by reference.
Function Pointers in C |
#include <stdio.h>
int add(int a, int b) { return a + b; } int subtract(int a, int b) { return a – b; } int main() { int (*functionPtr)(int, int); int a = 10, b = 5; char operation = ‘+’; if (operation == ‘+’) { functionPtr = add; }
else if (operation == ‘-‘) { functionPtr = subtract; } else { printf(“Invalid operation\n”); return 1; } int result = functionPtr(a, b); printf(“Result: %d\n”, result); return 0; } |
Here, we use a function pointer variable named ‘functionPtr’, which can point to either addition or subtraction. If the value of the operation is ‘+’, then it performs addition, and if it is ‘-’, it performs subtraction. It assigns the appropriate function to the pointer using conditional statements.
Output:
Function Pointer in C |
Result: 15 |
How to Reference and Derefernce A Pointer in C
Function pointer in C is used to assign the address of a function and can call the function directly with the help of these pointers. If we want to reference a pointer, we need to assign the address of the function. The pointer will store the address of the function.
Referencing a Function pointer in C |
int add(int a, int b) {
return a + b; } int (*functionPtr)(int, int); functionPtr = add; |
But when we want to dereference a function pointer then we only need to call the function pointer, just like we call a function. You need to provide the necessary arguments to the function pointer to deference it.
Dereferencing a Function pointer in C |
int result = functionPtr (2, 3);
printf (“Result: %d\n”, result); // Output: Result: 5 |
After providing the arguments to the function pointer, it will call the function and compute the code. The output result will be 5 in the above case.
Function Pointer Example
Let us understand the function pointer using the example given here in the table. Here, we use function pointers to find the addition, subtraction, multiplication or division of two numbers based on the value of the operation variable.
Function Pointer in C |
#include <stdio.h>
// Function prototypes for operations int add(int a, int b); int subtract(int a, int b); int multiply(int a, int b); int divide(int a, int b); int main() { int (*operation)(int, int); int a, b, result; char operator; printf(“Enter operation (+, -, *, /): “); scanf(” %c”, &operator); switch (operator) { case ‘+’: operation = add; break; case ‘-‘: operation = subtract; break; case ‘*’: operation = multiply; break; case ‘/’: operation = divide; Break; default: printf(“Invalid operation\n”); return 1; } printf(“Enter two numbers: “);
scanf(“%d %d”, &a, &b); result = operation(a, b); printf(“Result: %d\n”, result); return 0; } int add(int a, int b) { return a + b; } int subtract(int a, int b) { return a – b; } int multiply(int a, int b) { return a * b; } int divide(int a, int b) { if (b != 0) { return a / b; } else { printf(“Error: Division by zero\n”); return 0; } } |
PW Skills Recommended Courses
- Decode DSA with C++
- Full Stack Data Science Pro Course
- Java For Cloud Course
- Full Stack Web Development Course
- Data Analytics Course
Advantage of Function Pointer in C
Function Pointer in C has many benefits. Let us check out some of the major advantages of Function pointers.
- Function pointers are used to store the address of the function and point them whenever needed. Hence, they make the callback mechanism easy. You can easily pass a function as an argument in C.
- They make the program simple and short.
- Function pointers are used in a program to enhance the flexibility of a program.
- Function pointers make our code safe.
- We can select functions dynamically at runtime based on various conditions.
Recommended Reads
Top Features of C++ programming language
Function Pointer in C FAQs
Q1. What are function pointers in C?
Ans: The function pointers contain the address of the function, which we can access using pointers.
Q2. Is a function pointer a regular pointer?
Ans: No, function pointers are used to point an executable code, unlike regular pointers, which point data. With the help of function pointers, we can store the address of a function using a pointer variable.
Q3. What is the size of the function pointer in C?
Ans: The size of function pointers in C depends upon various factors such as operating system, system capabilities, version, etc.
Q4. What is the major advantage of using a function pointer in C?
Ans: Function pointer in C has many advantages, such as callback mechanism, dynamic function selection, testing and debugging, etc. Read the article to know more.