Basic Coding Questions: Coding interviews, much like the tech world’s Olympics, are where you prove your skills and problem-solving abilities. From explaining complex concepts to showcasing your interests beyond coding, these interviews demand a well-rounded view of your abilities. If you want to build a successful career as a coder, taking a course is highly recommended.
In this article, we discuss the 50 most asked basic coding questions during interviews. With the right insights and answers, you can face your coding interviews with confidence and secure that dream tech job. Let’s dive in!
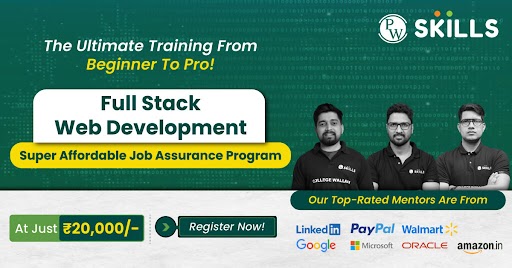
Basic Coding Questions
-
How do you reverse a string?
Reversing a string is a common coding task. Achieve this by looping through the string’s characters and constructing a reversed string.
Python Code
def reverse_string(input_string):
    return input_string[::-1]
-
How can you check whether a string is a palindrome or not?
A palindrome is a word or phrase that reads the same both ways, forward and backward. You can simply compare it to its reverse to determine if a string is a palindrome.
Python Code
def is_palindrome(input_string):
    return input_string == input_string[::-1]
-
How can you compute the number of numericals in a string?
You can inspect each character within the string by utilising the isdigit() method to determine if it’s a numeral.
Python Code
def count_numerical_digits(input_string):
    count = 0
    for char in input_string:
        if char.isdigit():
            count += 1
    return count
-
How do you find the count for the occurrence of a particular character in a string?
You can traverse the string and tally the instances of the specific character.
Python Code
def count_character_occurrences(input_string, char):
    count = 0
    for c in input_string:
        if c == char:
            count += 1
    return count
-
How do you find the non-matching characters in a string?
You can detect different characters in two equally long strings by comparing their corresponding characters and noting discrepancies.
Python Code
def find_non_matching_characters(str1, str2):
    non_matching = []
    for i in range(len(str1)):
        if str1[i] != str2[i]:
            non_matching.append(str1[i])
    return non_matching
-
How do you find out if the two given strings are anagrams?
Two strings are anagrams if they have the same characters in a different order. You can sort the characters in each string and compare them.
Python Code
def are_anagrams(str1, str2):
    return sorted(str1) == sorted(str2)
-
How do you calculate the number of vowels and consonants in a string?
You can iterate through the string and count the vowels and consonants.
Python Code
def count_vowels_and_consonants(input_string):
    vowels = “aeiou”
    num_vowels = 0
    num_consonants = 0
    for char in input_string:
        if char.isalpha():
            if char.lower() in vowels:
                num_vowels += 1
            else:
                num_consonants += 1
    return num_vowels, num_consonants
-
How do you total all of the matching integer elements in an array?
Iterate through the array, adding up matching integer elements to find their sum.
Python Code
def sum_matching_elements(arr, target):
    total = 0
    for num in arr:
        if num == target:
            total += num
    return total
-
How do you reverse an array?
Reversing an array can be done by swapping elements from the start and end until you reach the middle of the array.
Python Code
def reverse_array(arr):
    left = 0
    right = len(arr) – 1
    while left < right:
        arr[left], arr[right] = arr[right], arr[left]
        left += 1
        right -= 1
-
How do you find the maximum element in an array?
To discover the array’s highest value, scan through it and remember the greatest encountered.
Python Code Code
def find_max_element(arr):
    if not arr:
        return None
    max_element = arr[0]
    for num in arr:
        if num > max_element:
            max_element = num
    return max_element
Basic Coding Questions for Freshers
-
How do you sort an array of integers in ascending order?
You can employ sorting methods like bubble sort, selection sort, or the innate sorting features in programming languages.
Python Code
def sort_array_ascending(arr):
    arr.sort()
-
How do you print a Fibonacci sequence using recursion?
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. You can print it using recursion.
Python Code
def print_fibonacci(n, a=0, b=1):
    if n == 0:
        return
    print(a)
    print_fibonacci(n – 1, b, a + b)
-
How do you calculate the sum of two integers?
To calculate the sum of two integers, you can simply add them.
Python Code
def sum_of_integers(a, b):
    return a + b
-
How do you find the average of numbers in a list?
To find the average of numbers in a list, sum all the numbers and divide by the count of numbers.
Python Code
def average_of_numbers(numbers):
    if not numbers:
        return 0
    return sum(numbers) / len(numbers)
-
How do you check if an integer is even or odd?
You can check if an integer is even by dividing it by 2 and checking the remainder.
Python Code
def is_even(number):
    return number % 2 == 0
-
How do you find the middle element of a linked list?
To determine a linked list’s midpoint, employ a method with two pointers. One progresses by one step, while the other advances by two steps.
Python Code
def find_middle_element(head):
    slow = head
    fast = head
    while fast is not None and fast.next is not None:
        slow = slow.next
        fast = fast.next.next
    return slow
-
How do you remove a loop in a linked list?
Detecting and removing a loop in a linked list is a complex algorithmic task and typically involves Floyd’s Tortoise and Hare algorithm.
Python Code
def detect_and_remove_loop(head):
    # Implementation varies, depending on the data structure used.
    # You can use Floyd’s Tortoise and Hare algorithm to detect the loop,
    # and then remove it.
-
How do you merge two sorted linked lists?
Merging two sorted linked lists is a common operation when dealing with linked data structures.
Python Code
class ListNode:
    def __init__(self, val=0, next=None):
        self.val = val
        self.next = next
def merge_sorted_lists(l1, l2):
    dummy = ListNode(0)
    current = dummy
    while l1 is not None and l2 is not None:
        if l1.val < l2.val:
            current.next = l1
            l1 = l1.next
        else:
            current.next = l2
            l2 = l2.next
        current = current.next
    current.next = l1 or l2
    return dummy.next
-
How do you implement binary search to find an element in a sorted array?
Binary search is an efficient algorithm to find an element in a sorted array.
Python Code
def binary_search(arr, target):
    left, right = 0, len(arr) – 1
    while left <= right:
        mid = left + (right – left) // 2
        if arr[mid] == target:
            return mid
        elif arr[mid] < target:
            left = mid + 1
        else:
            right = mid – 1
    return -1 # Element not found
-
How do you print a binary tree in vertical order?
Printing a binary tree in vertical order involves a bit more complex logic. You can use a map or dictionary to store nodes at different vertical levels.
Python Code
class TreeNode:
    def __init__(self, val=0, left=None, right=None):
        self.val = val
        self.left = left
        self.right = right
def print_vertical_order(root):
    def vertical_order_traversal(node, distance, level, result):
        if node is None:
            return
        if distance in result:
            result[distance].append((level, node.val))
        else:
            result[distance] = [(level, node.val)]
        vertical_order_traversal(node.left, distance – 1, level + 1, result)
        vertical_order_traversal(node.right, distance + 1, level + 1, result)
    result = {}
    vertical_order_traversal(root, 0, 0, result)
    for distance in sorted(result.keys()):
        for _, val in sorted(result[distance]):
            print(val, end=’ ‘)
        print()
Also Check: C++ Programming Basics Every Programming Enthusiast Must Know
Basic Coding Questions and Answers
-
What is a data structure?
A data structure organises and stores data in a computer’s memory or storage. It specifies how data elements relate and what operations are possible.
-
What is an array?
An array stores elements, each identified by an index or key. It allows accessing and manipulating a group of items of the same kind.
-
What is a linked list?
A linked list is a linear structure with elements, each having a reference to the next. Linked lists efficiently handle data insertions and deletions.
-
What is the difference between an array and a linked list?
Arrays have a fixed size, but linked lists can grow or shrink as needed. When you access array elements, it’s fast thanks to direct indexing, taking constant time. However, inserting or deleting elements is slower. In contrast, linked lists offer efficient insertion and deletion but slower access time, which takes linear time.
-
What is LIFO (Last-In-First-Out)?
LIFO, a concept in data structures, means the most recently added element is the first to be taken out. It’s often linked with stack data structures.
-
What is FIFO (First-In-First-Out)?
FIFO means the first element added is the first to be removed in data structures, often linked with queue data structures.
-
What is a stack?
A stack is a linear data structure that adheres to the Last In, First Out (LIFO) principle. It enables actions such as pushing to insert an element and popping to eliminate the top element.
-
What are binary trees?
Binary trees are tree structures with nodes having a maximum of two children: the left child and the right child.
-
What are binary search trees?
A binary search tree sorts values. The left side has smaller or equal values. The right side has greater values. This setup makes searching and sorting fast.
-
What is object-oriented programming?
OOP organises code with objects. It uses classes, encapsulation, inheritance, and polymorphism to solve complex problems.
Coding Interview Questions with Solutions
-
What is the purpose of a loop in programming?
Loops in programming are used to repeat a specific block of code multiple times. They are essential for performing repetitive tasks, iterating over data structures, and solving problems that involve multiple iterations.
-
What is a conditional statement?
A conditional statement in programming enables the execution of code blocks depending on the truth or falsehood of a given condition. Common conditional statements encompass “if,” “else,” and “switch” statements.
-
What is debugging?
Debugging means locating and rectifying errors in a program, known as bugs. It entails tracing the code to pinpoint issues and making essential adjustments to guarantee the program’s proper operation.
-
What is recursion?
Recursion, a programming method, involves a function calling itself to solve problems. It’s valuable for addressing issues that can be subdivided into smaller, analogous sub-problems.
-
What are the differences between linear and non-linear data structures?
Arrays and linked lists hold data one after another, while trees and graphs have intricate connections between elements. Linear structures follow a strict sequence, while non-linear ones allow complex relationships.
-
What programming languages do you have experience working with?
During coding interviews, you might face questions about your expertise in certain programming languages. Get ready to talk about the languages you’re skilled in, detailing their advantages and drawbacks.
-
Describe a time you faced a challenge in a project you were working on and how you overcame it.
Use the STAR method (Situation, Task, Action, Result) to structure your response. Explain the challenge, your role, actions taken, and the positive outcomes.
-
Walk me through a project you’re currently or have recently worked on.
Provide an overview, your role, technologies used, challenges faced, and the project’s results.
-
Give an example of a project where you had to learn a new programming language or technology. How did you learn it?
New Skill Acquisition: Detail the necessity, course-based or peer-driven acquisition, challenges faced, and impact on project success.
-
How do you ensure your code is readable by other developers?
Highlight best practices like clear variable names, comments, consistent style, modular code, testing, and code reviews.
-
What are your interests outside of programming?
Share your hobbies or interests to present a well-rounded image. Mention activities like hiking, playing musical instruments, or other passions.
-
How do you keep your skills sharp and up to date?
Explain how you stay current with the tech industry – online courses, workshops, news sources, and practical applications in your work.
-
How do you collaborate on projects with non-technical team members?
Explain your strategy for clear communication, linking technology and non-technical aspects, and the collaboration tools you employ.
-
Tell me about a time when you had to explain a complex technical concept to a non-technical team member.
Use the STAR method to describe the situation, the concept, how you simplified it, and the positive result.
-
How do you get started on a new coding project?
Outline your structured approach: project understanding, planning, design, coding, testing, collaboration, documentation, deployment, and ongoing maintenance.
-
Explain the concept of time complexity in algorithms.
Time complexity measures the efficiency of an algorithm in terms of the time it takes to run as a function of the input size. It’s usually denoted using big O notation (O()). “In computer science, O(1) means constant time, O(n) signifies linear time, and O(n^2) represents quadratic time. When evaluating algorithms, selecting the most efficient one for a specific problem depends on its time complexity.”
-
What is the significance of version control, and which version control systems are you familiar with?
Version control systems are essential for managing changes in code and collaborating with others. Version control systems aid in monitoring and recording alterations, simplifying cooperation, reverting adjustments, and addressing disputes. Well-known systems are Git, SVN, and Mercurial. I am proficient in Git and have used it extensively for tracking changes and collaborating on code projects.
-
Can you explain the differences between SQL and NoSQL databases?
SQL (Structured Query Language) and NoSQL databases are distinct in their data models. SQL databases are relational, use tables, and ensure data consistency and integrity. NoSQL databases, on the other hand, are non-relational, using various data models like key-value, document, column-family, or graph. They offer more flexibility but may sacrifice some ACID (Atomicity, Consistency, Isolation, Durability) properties.
-
What is a design pattern in software development, and can you give an example?
A design pattern is a reusable solution to common problems in software design. It provides a template for structuring code to solve a specific issue efficiently. An example is the Singleton pattern, which ensures that a class has only one instance and provides a global point of access to it. This is useful when you want to limit a resource’s availability.
-
How do you handle exceptions and errors in your code?
I handle exceptions and errors by using try-catch blocks. In the try block, I place code that might raise an exception. In the catch block, I specify how to handle the exception – whether to log it, display a user-friendly error message, or take specific actions to recover from it. Handling exceptions gracefully is crucial to ensure that the application doesn’t crash and provides a better user experience.
Also check: Backend Developer Skills That Employers Look for in 2023
Conclusion
Coding interviews are the gateways to coveted tech positions. They’re all about solving complex problems, showcasing your coding skills, and vying for that dream job. It’s an exhilarating challenge, and we hope that this blog helped you with the skills to excel. Whether you’re a coding enthusiast or a seasoned pro, you need to master the art of nailing coding interviews.
If you want to level up in your coding career, enroll in a course form PW Skills today! Get mentored from experts in the industry and get 100% Job Assurance with every course! So, don’t wait, enrol today!
FAQs
What's the purpose of the STAR method in interview responses?
The STAR method (Situation, Task, Action, Result) is a structured approach to answer behavioural interview questions. It helps you provide a well-organised and comprehensive response, highlighting your skills and achievements.
What's the difference between procedural and object-oriented programming?
Procedural programming focuses on procedures or functions, while object-oriented programming emphasises the use of objects, combining data and the methods that manipulate it to promote code reusability and maintainability.
How does continuous integration benefit software development?
Continuous integration (CI) involves regularly merging code changes into a shared repository and running automated tests. It helps identify and fix integration issues early, resulting in more stable and reliable software.
Why is algorithm analysis important in coding interviews?
Algorithm analysis demonstrates your ability to assess the efficiency and performance of code. It's crucial in solving complex problems with optimal solutions.
Can you provide an example of the Factory Design Pattern in software development?
The Factory Design Pattern involves creating an interface for object creation but allowing subclasses to alter the type of objects that will be created. For instance, a car manufacturing process can be implemented using this pattern.
What's the difference between HTTP and HTTPS?
HTTP (Hypertext Transfer Protocol) is unsecured, while HTTPS (Hypertext Transfer Protocol Secure) encrypts data transmission, ensuring secure communication over the internet.
How do you approach code refactoring in a project?
Code refactoring involves restructuring code to improve its readability, maintainability, and performance without changing its external behaviour. It's essential to prioritise code quality and avoid introducing new bugs during the process.
What are design principles like SOLID in software development?
SOLID is an acronym representing five design principles: Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion. These principles guide the creation of maintainable and extensible software.
What's the role of load balancing in web applications?
Load balancing distributes incoming network traffic across multiple servers to enhance the application's availability, scalability, and reliability.
Why is responsive web design important in front-end development?
Responsive web design ensures that web applications adapt to various screen sizes and devices, providing a seamless user experience.