Learn effective Python programming with OOPS Concepts in Python. Whether you’re a beginner or a seasoned coder, this blog breaks down concepts like abstraction and polymorphism for your understanding!
OOPS Concepts In Python: Java’s Object-Oriented Programming System (OOPS) hinges on four pivotal principles — the core tenets of Object-Oriented Programming (OOPS) in Java. Python, a dynamic and versatile language, effortlessly incorporates OOPS Concepts In Python, providing developers with a potent set of tools.
This article discussed the core ideas of OOPS Concepts In Python. It leads readers through concepts like classes, objects, encapsulation, inheritance, polymorphism, and abstraction. Exploring constructors, destructors, and design principles such as SOLID reveals the core of Pythonic OOP.
Real-world case studies illustrate the practical use of these principles, enabling developers to craft modular, maintainable, and scalable code in the Python ecosystem.
If you want to make a successful and impactful career as a web developer, then PhysicsWallah’s Full Stack Web Development Course is highly beneficial for you. In our course, you’ll be mentored by industry leaders that will teach you everything you need to know for success! So, don’t wait! Enroll now and apply the coupon code “READER” for an discount on the course!
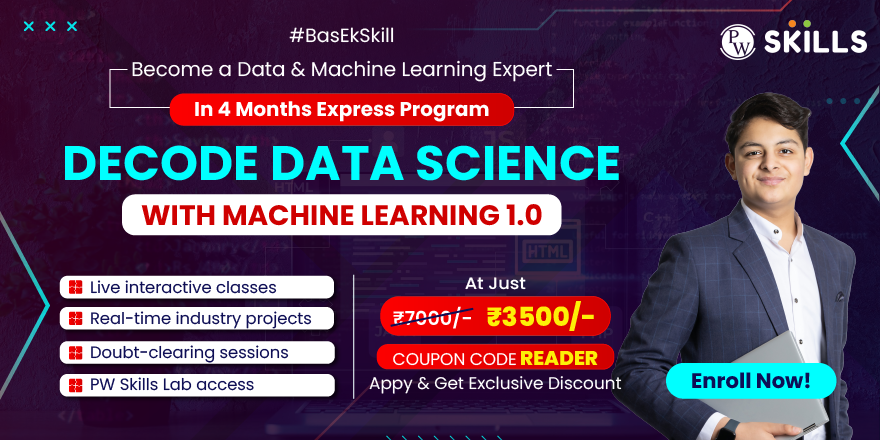
What Are OOPS Concepts in Python?
Object-oriented programming (OOP) in Python is a programming paradigm centred around the concept of “objects,” which encapsulate data and behaviour. Python seamlessly integrates OOP principles, providing a robust framework for software development. In OOP, classes act as blueprints for objects, defining their attributes and methods.
Encapsulation ensures data security, allowing the bundling of data and methods within a class. Inheritance lets you make new classes from ones that already exist, promoting the reuse of code. Polymorphism allows objects to take on multiple forms, enhancing flexibility. Abstraction simplifies complex systems by highlighting essential details.
Constructors and destructors manage object initialization and resource cleanup. Pythonic OOP involves idiomatic practices using properties, decorators, and magic methods. By applying these principles, developers create modular, scalable, and maintainable code, enhancing the efficiency and readability of Python programs.
Also read: Java Or Python: Which Language Should You Learn in 2023?
Features of OOPS Concepts in Python
Python showcases OOP with powerful features for systematic software development.
- Classes and Objects
- OOP starts with classes, which act as blueprints for creating objects. Objects are instances of these classes, encapsulating both data and functionality.
- Encapsulation
- Encapsulation involves bundling data and the methods that operate on the data within a single unit, a class. This ensures that the internal workings of an object are hidden from the outside world, promoting data security and code organisation.
- Inheritance
- Inheritance allows a class to inherit properties and methods from another class. This promotes code reuse and establishes a hierarchy of classes. In Python, a class can inherit from one or more classes, supporting single and multiple inheritance.
- Polymorphism enables objects to take multiple forms. In Python, this can be achieved through method overloading and method overriding. Polymorphism enhances flexibility by allowing different objects to be treated uniformly, promoting code extensibility.
- Abstraction involves simplifying complex systems by modelling classes based on the essential features they provide. Abstract classes and methods in Python allow developers to define blueprints without providing a complete implementation, leaving room for further customization in derived classes.
- Constructors and Destructors
- Constructors initialise objects when they are created, ensuring that they start with a valid state. Python supports both the __init__ method as a constructor and the __del__ method as a destructor for resource cleanup. Constructors contribute to object initialization, while destructors handle cleanup tasks when an object is no longer in use.
- Composition vs. Inheritance
- OOP in Python allows developers to choose between composition and inheritance. Composition involves creating relationships between classes by including instances of other classes, promoting code flexibility. This provides an alternative to traditional inheritance, allowing for better code maintainability in specific scenarios.
- Design Principles (SOLID)
- SOLID principles (Single Responsibility Principle, Open/Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle) guide developers in creating modular, maintainable, and scalable code. These principles enhance the robustness of OOP in Python by promoting good design practices.
OOP in Python provides a comprehensive set of features that facilitate code organisation, reusability, and maintainability. By leveraging classes, encapsulation, inheritance, polymorphism, abstraction, constructors, and design principles, developers can build efficient and scalable software solutions in a structured manner.
Recommended Technical Course
- Full Stack Web Development Course
- Generative AI Course
- DSA C++ Course
- Java+DSA 1.0 Course
- Data Analytics Course
- Data Science with ML 1.0 Course
Composition vs. Inheritance
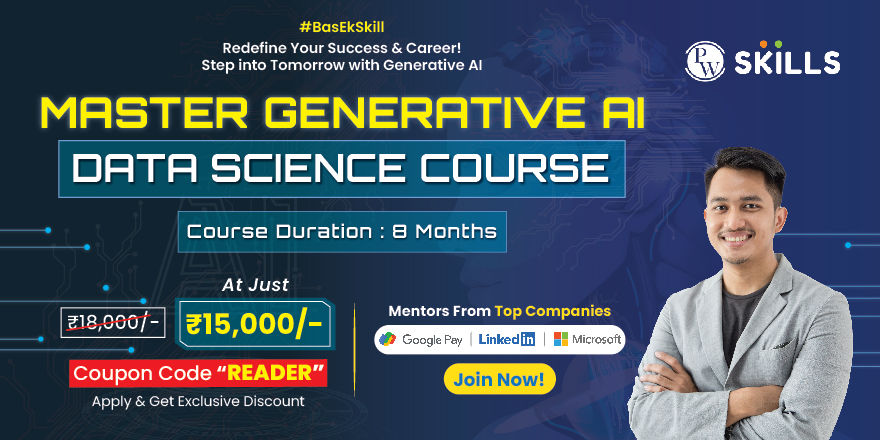
Composition and inheritance are two fundamental concepts in Object-Oriented Programming (OOP) that address code organisation and reuse. Both approaches offer distinct advantages, and the choice between them often depends on the specific requirements of a given situation.
Inheritance
Inheritance is a mechanism that allows a class to inherit properties and behaviours from another class. The derived class, also known as the child class, inherits attributes and methods from a base class, referred to as the parent class.
This promotes code reuse and establishes an “is-a” relationship between the two classes. While inheritance can lead to cleaner code in some instances, it comes with challenges such as the potential for tight coupling, where changes in the base class may affect the derived class.
Composition
Composition, on the other hand, involves creating relationships between classes by including instances of other classes within a class. Instead of relying on an “is-a” relationship, composition fosters a “has-a” relationship.
This approach promotes flexibility and allows for a more modular design. Components can be swapped or modified without affecting the entire structure. Composition is particularly useful in scenarios where there is no clear hierarchical relationship between objects, or where flexibility and maintainability are top priorities.
Choosing Between Composition and Inheritance
- Code Reusability
- Inheritance: Ideal when there is a clear hierarchical relationship, and the derived class shares a significant portion of the functionality of the base class.
- Composition: More flexible for scenarios where code reuse is achieved by combining different components, rather than inheriting from a common ancestor.
- Flexibility and Maintenance
- Inheritance: Changes in the base class may impact derived classes, potentially leading to a cascade of modifications.
- Composition: Components can be modified or replaced independently, promoting a more modular and maintainable design.
- Complexity and Structure
- Inheritance: Provides a clear structure but may lead to a rigid hierarchy, making it challenging to adapt to changing requirements.
- Composition: Offers a more dynamic structure, allowing for greater adaptability and ease of modification.
- Design Goals
- Inheritance: Suited for scenarios where a clear hierarchy and code sharing are essential.
- Composition: Beneficial when flexibility, maintainability, and the ability to adapt to changes are top priorities.
A combination of both composition and inheritance is often employed, with developers leveraging the strengths of each approach to create well-structured, modular, and maintainable code. The choice between composition and inheritance should be guided by the specific needs and goals of the software being developed.
Also read: Full Form of OOPS: Applications, Advantages, Future Trends
Python Objects
In Python, everything is an object. Understanding Python objects is fundamental to grasping the principles of Object-Oriented Programming (OOP) in the language. Here’s a comprehensive look at Python objects:
- Everything is an Object
- In Python, everything, from simple data types like integers and strings to more complex structures like functions and classes, is treated as an object. This adherence to the object-oriented paradigm is a key feature that simplifies code organisation and promotes consistency.
- Objects and Identity
- Each object in Python has a unique identity, which is essentially its memory address. The id() function can be used to retrieve the identity of an object. This identity remains constant throughout the object’s lifetime.
- Attributes and Methods
- Objects in Python have attributes and methods. Attributes are characteristics or properties of the object, while methods are functions associated with the object. They are accessed using the dot notation: object.attribute or object.method().
- Mutable vs. Immutable Objects
- Objects in Python can be classified as mutable or immutable. Mutable objects can be modified after creation (e.g., lists, dictionaries), while immutable objects cannot be changed once created (e.g., strings, tuples).
- Creating Custom Objects – Classes
- Python allows the creation of custom objects through classes. A class serves as a blueprint for creating instances, or objects, which encapsulate data and behaviour. Classes define attributes and methods that instances inherit.
- Instantiation and Initialization
- Creating an object from a class is called instantiation. The __init__ method in a class is used for object initialization, allowing attributes to be set when the object is created.
- Dynamic Typing
- Python is dynamically typed, meaning the type of an object is determined at runtime. This flexibility allows variables to reference different types of objects over their lifetime.
- Garbage Collection:
- Python employs automatic garbage collection to reclaim memory occupied by objects that are no longer in use. The Python memory manager handles the process, and developers don’t need to explicitly free memory.
- Object Comparison:
- The == operator compares the values of objects, while the is operator checks for identity. Understanding the difference between these two is crucial, especially when dealing with mutable objects.
- Magic Methods
- Python objects can define unique methods, often referred to as “magic methods” or “dunder methods” (double underscore methods), such as __str__ and __len__. These methods provide customised behaviour for objects, making them more versatile.
- Python objects adhere to key OOP principles like encapsulation, inheritance, and polymorphism. These principles guide developers in creating organised, reusable, and extensible code.
Python’s object model is at the core of the language’s design philosophy. Embracing objects and understanding their properties, methods, and dynamic nature is essential for effective and idiomatic Python programming. Whether working with built-in types or creating custom classes, the object-oriented paradigm is central to the Python language.
Also read: OOPs Concepts in Java with Examples & Interview Questions
How Do You Instantiate a Class in Python?
In Python, instantiation is the process of creating an object from a class. To instantiate a class, follow these steps:
- Define the Class:
- Begin by defining a class using the class keyword. Inside the class, define attributes and methods that will characterise the objects created from it.
- Create an Instance:
- To create an instance (object) of the class, call the class as if it were a function. This invokes the class’s constructor method, usually named __init__, which initialises the object’s attributes.
- Set Object Attributes:
- If the class has an __init__ method with parameters, provide values for those parameters when creating the object. These values initialise the object’s attributes.
- Once instantiated, you can access the object’s attributes and methods using dot notation (my_car.make, my_car.model). This allows interaction with the object’s properties and behaviours.
In summary, instantiation involves creating an instance of a class by calling the class as a function. The class’s constructor initialises the object’s attributes, providing a blueprint for creating and interacting with objects in Python.
Python’s object-oriented paradigm provides a versatile and consistent approach to programming. With everything treated as an object, developers benefit from dynamic typing, encapsulation, and powerful features like magic methods. The language’s emphasis on objects, whether built-in or custom, fosters modular and maintainable code. Understanding Python objects is not just a fundamental aspect of programming in the language; it’s a gateway to unlocking the full potential of Object-Oriented
Programming, empowering developers to create efficient, scalable, and organised solutions. Embracing Python’s object model is key to harnessing the language’s elegance and flexibility in diverse software development scenarios.
Also read: OOPS Concepts in Java: What It Is, Why Is it Called That, Advantages, and More
FAQs
What is Object-Oriented Programming (OOP) in Python?
Python's OOP is a programming paradigm that structures code around objects, combining data and functionality for modular and organised development.
What distinguishes Python objects?
In Python, everything is an object, each with a unique identity and attributes. Objects can be mutable or immutable, offering flexibility in coding.
How are custom objects created in Python?
Custom objects are created using classes, serving as blueprints for instances. The __init__ method initialises object attributes, facilitating dynamic typing.
What is the difference between == and is in Python?
The == operator checks object values, while the is operator verifies object identity by comparing memory addresses.
How does Python handle memory management for objects?
Python employs automatic garbage collection to reclaim memory from unused objects, handled by the built-in memory manager.
Why use composition over inheritance in Python?
Composition promotes flexibility by creating relationships between classes without establishing a rigid hierarchy, enhancing code maintainability.
What role do magic methods play in Python objects?
Magic methods, such as __str__ and __len__, provide customised behaviour for objects, making them adaptable and enhancing their usability in Python code.