Anagram in Java defines two strings or phrases considered anagrams if they contain the same letters but in different arrangements. In Java, identifying anagrams involves writing code that can detect whether two given strings meet this condition.
Anagram in Java: In computer science, anagrams refer to two strings or phrases that share the same set of letters but in different orders. For all Java developers, learning the art of detecting anagrams is a fundamental skill. And once you understand it, your path to master string manipulation, algorithmic thinking, and problem-solving techniques will open.
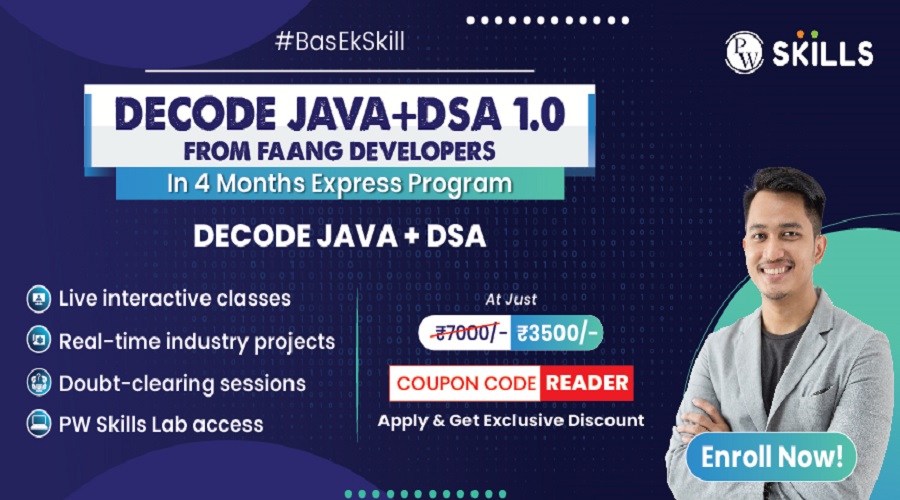
So, whether you need to solve any anagram problems in your assignment, upscale your skills to advance your programming career, or have a passion for coding, this guide with the best examples, programs, and their solution can help you learn more about Anagram in Java.
Moreover, if you want to master the art of Java development, we recommend Physics Wallah’s DSA Java course. As our regular readers, we suggest you apply the “READER” coupon code to get an exclusive discount on this course.
Anagram in Java Definition
An anagram in Java refers to a pair of words or phrases that are created by rearranging the letters of each other. In other words, two strings are considered anagrams if they have the same characters with the same frequency, but the order of the characters is different.
For example, “listen” and “silent” are anagrams because you can rearrange the letters in one to form the other. The critical characteristic of anagrams is that they contain the same set of letters.
In Java, creating a program to check if two strings are anagrams involves comparing the characters in both strings and ensuring that they have the same frequency of occurrence. This often requires removing spaces, converting letters to a typical case (uppercase or lowercase), and sorting or using other methods to compare the characters.
Anagram-related operations in Java may include
Checking if Two Strings are Anagrams
- Comparing characters case-insensitively.
- Ensuring that the two strings have the same length.
- Sorting characters or using other methods to compare.
Finding All Anagrams of a Given Word
- Generating permutations of the input word.
- Checking each permutation for anagram conditions.
Optimizing Anagram Checking
- Using data structures like maps to store character frequencies.
- Efficiently comparing the frequency maps for both strings.
Understanding anagrams is crucial in various applications, such as word games, cryptography, and even problem-solving scenarios where recognizing anagrams is necessary.Â
Java provides a flexible and powerful environment for efficiently implementing algorithms to work with anagrams.
Anagram in Java With Example
Below is an example of checking if two strings are anagrams in Java:
import java.util.Arrays;
public class AnagramExample {
    public static boolean areAnagrams(String str1, String str2) {
        // Remove spaces and convert to lowercase for case-insensitive comparison
        str1 = str1.replaceAll(“\\s”, “”).toLowerCase();
        str2 = str2.replaceAll(“\\s”, “”).toLowerCase();
        // Check if lengths are different
        if (str1.length() != str2.length()) {
            return false;
        }
        // Convert strings to char arrays and sort them
        char[] charArray1 = str1.toCharArray();
        char[] charArray2 = str2.toCharArray();
        Arrays.sort(charArray1);
        Arrays.sort(charArray2);
        // Compare the sorted char arrays
        return Arrays.equals(charArray1, charArray2);
    }
    public static void main(String[] args) {
        String word1 = “Listen”;
        String word2 = “Silent”;
        if (areAnagrams(word1, word2)) {
            System.out.println(word1 + ” and ” + word2 + ” are anagrams.”);
        } else {
            System.out.println(word1 + ” and ” + word2 + ” are not anagrams.”);
        }
    }
}
Explanation Of This Example!
- The areAnagrams method takes two strings, removes spaces, and converts them to lowercase for case-insensitive comparison.
- It checks if the lengths of the two strings are different. If they are, the strings cannot be anagrams.
- The characters in both strings are converted to char arrays and sorted.
- Finally, the sorted arrays are compared using Arrays.equals() to determine if the strings are anagrams.
When you run this program with the provided example words “Listen” and “Silent,” it will print:
Solution: Listen and Silent are anagrams.
Pangram in Java
A pangram is a sentence that includes each letter of the alphabet at least once.. Creating a Java program to check if a given sentence is a pangram involves checking for the presence of each letter in the alphabet. Here’s an example:
public class PangramChecker {
    public static boolean isPangram(String sentence) {
        // Convert the sentence to lowercase for case-insensitive comparison
        sentence = sentence.toLowerCase();
        // Create a boolean array to mark the presence of each letter
        boolean[] isPresent = new boolean[26];
        // Iterate through each character in the sentence
        for (int i = 0; i < sentence.length(); i++) {
            char currentChar = sentence.charAt(i);
            // Check if the character is a lowercase letter
            if (currentChar >= ‘a’ && currentChar <= ‘z’) {
                // Mark the presence of the letter in the boolean array
                isPresent[currentChar – ‘a’] = true;
            }
        }
        // Check if all letters are present in the boolean array
        for (boolean letterPresent : isPresent) {
            if (!letterPresent) {
                return false; // Not a pangram
            }
        }
        return true; // All letters are present, it’s a pangram
    }
    public static void main(String[] args) {
        String exampleSentence = “The quick brown fox jumps over the lazy dog”;
        if (isPangram(exampleSentence)) {
            System.out.println(“The sentence is a pangram.”);
        } else {
            System.out.println(“The sentence is not a pangram.”);
        }
    }
}
Explanation Of This Example!
The isPangram method takes a sentence as input.
- It converts the sentence to lowercase to perform a case-insensitive comparison.
- A boolean array isPresent is used to mark the presence of each letter in the alphabet.
- The method iterates through each character in the sentence, marks the presence of lowercase letters, and ignores non-alphabetic characters.
- Finally, it checks if all the letters are in the boolean array, indicating that the sentence is a pangram.
When you run this program with the provided example sentence, it will print:
Solution: The sentence is a pangram.
Anagram Program In Java Without Sorting
Creating a program anagram in Java without sorting involves a different approach. Instead of sorting the characters, you can use character frequency maps to compare the occurrence of each character in both strings. This approach is more efficient regarding time complexity, as it avoids the sorting step. Here’s an in-depth explanation and an example program:
import java.util.HashMap;
import java.util.Map;
public class AnagramWithoutSorting {
    public static boolean areAnagrams(String str1, String str2) {
        // Remove spaces and convert to lowercase for case-insensitive comparison
        str1 = str1.replaceAll(“\\s”, “”).toLowerCase();
        str2 = str2.replaceAll(“\\s”, “”).toLowerCase();
        // Check if lengths are different
        if (str1.length() != str2.length()) {
            return false;
        }
        // Use a map to store character frequencies for str1
        Map<Character, Integer> frequencyMap = new HashMap<>();
        // Populate frequency map for str1
        for (char c : str1.toCharArray()) {
            frequencyMap.put(c, frequencyMap.getOrDefault(c, 0) + 1);
        }
        // Check character frequencies for str2
        for (char c : str2.toCharArray()) {
            // If the character is not present in the map or its frequency is zero, not an anagram
            if (!frequencyMap.containsKey(c) || frequencyMap.get(c) == 0) {
                return false;
            }
            // Update the frequency in the map
            frequencyMap.put(c, frequencyMap.get(c) – 1);
        }
        return true; // Both strings are anagrams
    }
    public static void main(String[] args) {
        String word1 = “listen”;
        String word2 = “silent”;
        if (areAnagrams(word1, word2)) {
            System.out.println(word1 + ” and ” + word2 + ” are anagrams.”);
        } else {
            System.out.println(word1 + ” and ” + word2 + ” are not anagrams.”);
        }
    }
}
Explanation of this example:
- The areAnagrams method takes two strings, removes spaces, and converts them to lowercase for case-insensitive comparison.
- It checks if the lengths of the two strings are different. If they are, the strings cannot be anagrams.
- A Map (HashMap) named frequencyMap is used to store the frequency of each character in the first string (str1).
- The method iterates through each character in the first string, populating the frequency map.
- It then iterates through each character in the second string (str2) and checks if the character is present in the frequency map and if its frequency is greater than zero. If not, the strings are not anagrams. If yes, it updates the frequency in the map.
- The strings are considered anagrams if the method completes the iteration without returning false.
This approach avoids sorting and provides a more direct way of checking if two strings are anagrams. It’s beneficial when optimizing for time complexity, especially in scenarios with large strings.
When you run this program with the examples of “listen” and “silent,” it will print:
Solution: listen and silent are anagrams.
Also Read: 2D Array in Java – Example, Questions
Java Anagrams HackerRank Solution
It is a Problem Statement when we talk about Anagram In Java. Here’s what you need to know:
The “Java Anagrams” problem on HackerRank requires determining if two strings are anagrams of each other. Anagrams are words or phrases formed by rearranging the letters of another word or phrase, using all the original letters exactly once. The comparison should be case-insensitive.
Example
If the input strings are “anagram” and “mangaar,” the function should return “Anagrams.” If the input strings are “Hello” and “hello,” the function should return “Not Anagrams.”
Solution
To solve this problem, you need to check whether the two input strings have the same frequency of each character. You can achieve this by maintaining character frequency maps for both strings and comparing them.
Here’s a detailed explanation and the Java code:
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class Solution {
    static boolean isAnagram(String a, String b) {
        // Check if lengths are different
        if (a.length() != b.length()) {
            return false;
        }
        // Convert strings to lowercase for case-insensitive comparison
        a = a.toLowerCase();
        b = b.toLowerCase();
        // Create frequency maps for each string
        Map<Character, Integer> frequencyMapA = new HashMap<>();
        Map<Character, Integer> frequencyMapB = new HashMap<>();
        // Populate frequency map for string a
        for (char c : a.toCharArray()) {
            frequencyMapA.put(c, frequencyMapA.getOrDefault(c, 0) + 1);
        }
        // Populate frequency map for string b
        for (char c : b.toCharArray()) {
            frequencyMapB.put(c, frequencyMapB.getOrDefault(c, 0) + 1);
        }
        // Compare frequency maps
        return frequencyMapA.equals(frequencyMapB);
    }
    public static void main(String[] args) {
        Scanner scan = new Scanner(System.in);
        String a = scan.next();
        String b = scan.next();
        scan.close();
        boolean result = isAnagram(a, b);
        System.out.println(result ? “Anagrams” : “Not Anagrams”);
    }
}
Explanation For The Example
- The isAnagram method takes two strings, a and b, as input and returns a boolean indicating whether they are anagrams.
- The method first checks if the lengths of the two strings are different. If they are, the strings cannot be anagrams.
- It then converts both strings to lowercase for case-insensitive comparison.
- Two frequency maps (frequencyMapA and frequencyMapB) are created to store the count of each character in each string.
- The method iterates through each character in each string and populates the corresponding frequency map.
- Finally, it compares the two frequency maps. If they are equal, the strings are anagrams; otherwise, they are not.
- The main method takes input strings from the user, calls the isAnagram method, and prints “Anagrams” or “Not Anagrams” based on the result.
This solution provides a reliable and efficient way to determine whether two strings are anagrams in a case-insensitive manner.
Anagram Program In Java Using Hashmap
A program of Anagram In Java using a HashMap involves counting the frequency of characters in each string and then comparing these frequencies. Here’s an in-depth explanation along with the Java code:
import java.util.HashMap;
import java.util.Map;
public class AnagramWithHashMap {
    public static boolean areAnagrams(String str1, String str2) {
        // Remove spaces and convert to lowercase for case-insensitive comparison
        str1 = str1.replaceAll(“\\s”, “”).toLowerCase();
        str2 = str2.replaceAll(“\\s”, “”).toLowerCase();
        // Check if lengths are different
        if (str1.length() != str2.length()) {
            return false;
        }
        // Create frequency maps for each string
        Map<Character, Integer> frequencyMap1 = buildFrequencyMap(str1);
        Map<Character, Integer> frequencyMap2 = buildFrequencyMap(str2);
        // Compare frequency maps
        return frequencyMap1.equals(frequencyMap2);
    }
    private static Map<Character, Integer> buildFrequencyMap(String str) {
        Map<Character, Integer> frequencyMap = new HashMap<>();
        // Populate frequency map
        for (char c : str.toCharArray()) {
            frequencyMap.put(c, frequencyMap.getOrDefault(c, 0) + 1);
        }
        return frequencyMap;
    }
    public static void main(String[] args) {
        String word1 = “listen”;
        String word2 = “silent”;
        if (areAnagrams(word1, word2)) {
            System.out.println(word1 + ” and ” + word2 + ” are anagrams.”);
        } else {
            System.out.println(word1 + ” and ” + word2 + ” are not anagrams.”);
        }
    }
}
Explanation
- Removing Spaces and Lowercasing
The input strings are cleaned by removing spaces and converting them to lowercase. This ensures a case-insensitive and space-insensitive comparison.
- Checking Lengths
Before proceeding, the program checks if the lengths of the two strings are different. If they are, the strings cannot be anagrams.
- Frequency Map Construction
The buildFrequencyMap method is defined to construct a frequency map for a given string. It iterates through each character in the string and updates the frequency map accordingly.
- Frequency Maps for Both Strings
Frequency maps are constructed for both input strings (frequencyMap1 for str1 and frequencyMap2 for str2).
- Comparison of Frequency Maps
The program uses the equals method to compare the frequency maps. If the maps are equal, the strings have the same character frequencies, making them anagrams.
- Main Method
The main method demonstrates the usage of the areAnagrams method with example words. Based on the result, it prints whether the input words are anagrams.
This HashMap approach allows for efficient counting of character frequencies without explicitly sorting the characters. It’s a good alternative when you want to avoid the overhead of sorting, especially for longer strings or a large number of comparisons.
Anagram String Example
Let’s take an example to explain the concept of an anagram string in depth.
Example
Consider two strings, “listen” and “silent.” We want to determine if these two strings are anagrams.
Understanding Anagrams
Anagrams are words or phrases formed by rearranging the letters of another word or phrase, using all the original letters exactly once.
In our example, “listen” and “silent” are anagrams because you can rearrange the letters of “listen” to form “silent” and vice versa.
Character Frequency Analysis
- To check if two strings are anagrams, we can analyze the frequency of each character in both strings.
- For “listen”: {‘l’: 1, ‘i’: 1, ‘s’: 1, ‘t’: 1, ‘e’: 1, ‘n’: 1}
- For “silent”: {‘s’: 1, ‘i’: 1, ‘l’: 1, ‘e’: 1, ‘n’: 1, ‘t’: 1}
- Both strings have the same frequency of characters, so they are anagrams.
Code Implementation in Java
The following Java code checks if two strings are anagrams without sorting:
import java.util.HashMap;
import java.util.Map;
public class AnagramExample {
    public static boolean areAnagrams(String str1, String str2) {
        // Remove spaces and convert to lowercase for case-insensitive comparison
        str1 = str1.replaceAll(“\\s”, “”).toLowerCase();
        str2 = str2.replaceAll(“\\s”, “”).toLowerCase();
        // Check if lengths are different
        if (str1.length() != str2.length()) {
            return false;
        }
        // Create frequency maps for each string
        Map<Character, Integer> frequencyMap1 = buildFrequencyMap(str1);
        Map<Character, Integer> frequencyMap2 = buildFrequencyMap(str2);
        // Compare frequency maps
        return frequencyMap1.equals(frequencyMap2);
    }
    private static Map<Character, Integer> buildFrequencyMap(String str) {
        Map<Character, Integer> frequencyMap = new HashMap<>();
        // Populate frequency map
        for (char c : str.toCharArray()) {
            frequencyMap.put(c, frequencyMap.getOrDefault(c, 0) + 1);
        }
        return frequencyMap;
    }
    public static void main(String[] args) {
        String word1 = “listen”;
        String word2 = “silent”;
        if (areAnagrams(word1, word2)) {
            System.out.println(word1 + ” and ” + word2 + ” are anagrams.”);
        } else {
            System.out.println(word1 + ” and ” + word2 + ” are not anagrams.”);
        }
    }
}
Execution of the Code
When you run this Java program with the example words “listen” and “silent,” it will print:
Solution: listen and silent are anagrams.
This example of Anagram In Java demonstrates how to check if two strings are anagrams by analyzing the frequency of characters without explicitly sorting the characters. This efficient approach works well for various scenarios where anagram detection is required.
Also Read: About Java Language: Features, History, Full Meaning
Anagram Program In Java Using Scanner
Let’s create a program anagram in Java using the Scanner class to take user input for two strings and then determine if they are anagrams. We’ll provide an in-depth explanation along with the code:
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class AnagramWithScanner {
    public static boolean areAnagrams(String str1, String str2) {
        // Remove spaces and convert to lowercase for case-insensitive comparison
        str1 = str1.replaceAll(“\\s”, “”).toLowerCase();
        str2 = str2.replaceAll(“\\s”, “”).toLowerCase();
        // Check if lengths are different
        if (str1.length() != str2.length()) {
            return false;
        }
        // Create frequency maps for each string
        Map<Character, Integer> frequencyMap1 = buildFrequencyMap(str1);
        Map<Character, Integer> frequencyMap2 = buildFrequencyMap(str2);
        // Compare frequency maps
        return frequencyMap1.equals(frequencyMap2);
    }
    private static Map<Character, Integer> buildFrequencyMap(String str) {
        Map<Character, Integer> frequencyMap = new HashMap<>();
        // Populate frequency map
        for (char c : str.toCharArray()) {
            frequencyMap.put(c, frequencyMap.getOrDefault(c, 0) + 1);
        }
        return frequencyMap;
    }
    public static void main(String[] args) {
        // Create a Scanner object to take user input
        Scanner scanner = new Scanner(System.in);
        System.out.print(“Enter the first string: “);
        String word1 = scanner.nextLine();
        System.out.print(“Enter the second string: “);
        String word2 = scanner.nextLine();
        scanner.close(); // Close the scanner to avoid resource leaks
        // Check if the input strings are anagrams
        if (areAnagrams(word1, word2)) {
            System.out.println(word1 + ” and ” + word2 + ” are anagrams.”);
        } else {
            System.out.println(word1 + ” and ” + word2 + ” are not anagrams.”);
        }
    }
}
Explanation
- Scanner for User Input
- The program uses the Scanner class to take user input for two strings.
- It prompts the user to enter the first and second strings using System.out.print and scanner.nextLine().
- Removing Spaces and Lowercasing
Similar to the previous example, the input strings are cleaned by removing spaces and converting them to lowercase.
- Frequency Maps and Anagram Checking
- The areAnagrams method and buildFrequencyMap method are reused from the previous example.
- Frequency maps are constructed for both input strings and the method checks if they are equal.
- Closing the Scanner
- It’s crucial to close the Scanner object (scanner.close()) to avoid resource leaks when user input is no longer needed.
- Execution
- When you run this Java program, it will prompt you to enter two strings.
- After entering the strings, it will determine if they are anagrams and print the result.
This program allows the user to input two strings interactively, making it more versatile and user-friendly than hardcoding strings. It demonstrates how to use the Scanner class for user input and apply the anagram-checking logic.
Also Read: Algorithms In Java: List, Programs, Interview Questions
Anagram String In C
In C, you can create a program to check if two strings are anagrams by comparing the frequency of characters in each string. Here’s an example:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
// Function to remove spaces and convert to lowercase
void cleanString(char *str) {
    int i, j = 0;
    for (i = 0; str[i]; i++) {
        if (!isspace((unsigned char)str[i])) {
            str[j++] = tolower((unsigned char)str[i]);
        }
    }
    str[j] = ‘\0’;
}
// Function to check if two strings are anagrams
int areAnagrams(const char *str1, const char *str2) {
    // Clean strings (remove spaces and convert to lowercase)
    char cleanedStr1[strlen(str1) + 1];
    char cleanedStr2[strlen(str2) + 1];
    strcpy(cleanedStr1, str1);
    strcpy(cleanedStr2, str2);
    cleanString(cleanedStr1);
    cleanString(cleanedStr2);
    // Check if lengths are different
    if (strlen(cleanedStr1) != strlen(cleanedStr2)) {
        return 0; // Not anagrams
    }
    // Create frequency arrays for each string
    int frequencyMap1[26] = {0}; // Assuming only lowercase letters
    int frequencyMap2[26] = {0};
    // Populate frequency array for cleanedStr1
    for (int i = 0; cleanedStr1[i]; i++) {
        frequencyMap1[cleanedStr1[i] – ‘a’]++;
    }
    // Populate frequency array for cleanedStr2
    for (int i = 0; cleanedStr2[i]; i++) {
        frequencyMap2[cleanedStr2[i] – ‘a’]++;
    }
    // Compare frequency arrays
    for (int i = 0; i < 26; i++) {
        if (frequencyMap1[i] != frequencyMap2[i]) {
            return 0; // Not anagrams
        }
    }
    return 1; // Anagrams
}
int main() {
    // Input two strings
    char word1[100], word2[100];
    printf(“Enter the first string: “);
    scanf(“%s”, word1);
    printf(“Enter the second string: “);
    scanf(“%s”, word2);
    // Check if the input strings are anagrams
    if (areAnagrams(word1, word2)) {
        printf(“%s and %s are anagrams.\n”, word1, word2);
    } else {
        printf(“%s and %s are not anagrams.\n”, word1, word2);
    }
    return 0;
}
Explanation
- Cleaning the Strings
The cleanString function removes spaces from a string and converts it to lowercase. This ensures case-insensitive and space-insensitive comparison.
- Checking Lengths
The program checks if the lengths of the cleaned strings are different. If they are, the strings cannot be anagrams.
- Frequency Arrays
Frequency arrays (frequencyMap1 and frequencyMap2) are used to store the count of each character in the cleaned strings.
- Populating Frequency Arrays
The program iterates through each character in the cleaned strings and updates the corresponding frequency arrays.
- Comparing Frequency Arrays
It compares the frequency arrays to determine if the strings have the same character frequencies.
- Input and Output
The main function takes user input for two strings, calls the areAnagrams function, and prints whether the input strings are anagrams or not.
When you run this C program, it will prompt you to enter two strings, and it will determine if they are anagrams based on the provided logic.
Therefore these are some of the best examples, programs with their solutions with detailed explanation. We hope you have whatever your purpose to learn Anagram In Java will now be fulfilled.
You must check out our Physics Wallah’s DSA Java Course course and apply the coupon code “READER” and get an exclusive discount when subscribing to our best course.
For Latest Tech Related Information, Join Our Official Free Telegram Group :Â PW Skills Telegram Group
Anagram In Java FAQs
What is an anagram in Java?
An anagram in Java refers to two strings or phrases that share the same set of letters, but in different orders. Detecting anagrams involves comparing the characters in the strings and ensuring they have the same frequency.
What is an anagram in Javatpoint?
In Java, an anagram denotes two strings or phrases formed by rearranging the letters of each other. It involves checking if the strings have the same characters with the same frequency but in different arrangements.
How to check if two strings are anagrams in Java?
To check if two strings are anagrams in Java, you can compare their character frequencies after removing spaces, converting to lowercase, and checking for equal lengths. Sorting or using frequency maps are common approaches.
How to find all the anagrams of a word in Java?
Finding all anagrams of a word in Java typically involves generating permutations of the input word and checking each permutation for anagram conditions. This can be achieved through recursive algorithms or iterative methods.