API Full Form in Java is Application Programming Interface. It serves as a software mediator that facilitates communication between two distinct applications.
API Full Form in Java: Are you tired of constantly hearing the term “API” being thrown around but not fully understanding what it stands for or how it works? Look no further, as this blog post will provide you with a comprehensive overview of API in Java. What better way to do so than by joining Decode Java+DSA 1.0 by Physics Wallah – one of the best Java courses out there.Â
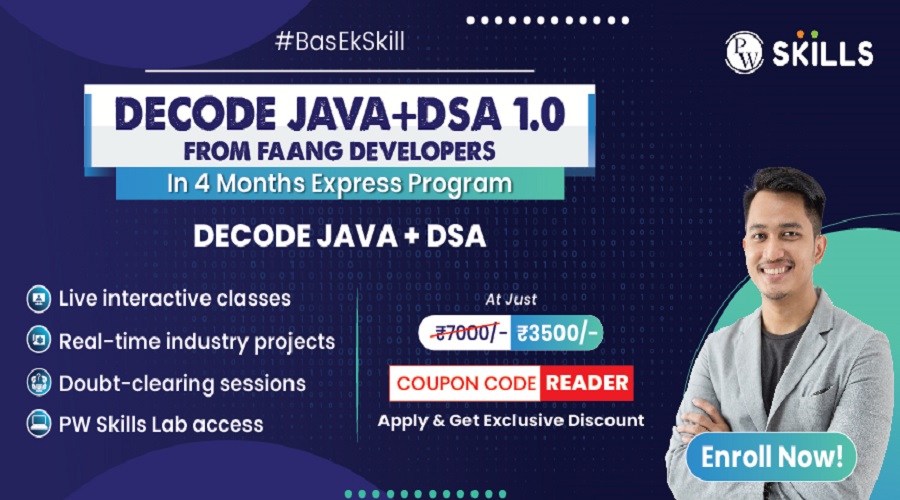
By utilizing their comprehensive syllabus and practical projects, you will not only gain a deeper understanding of API but also excel in your overall Java skills. Plus, applying the “READER” coupon at checkout will give you an exclusive discount!
API in Java Full Form
The full form of API in Java stands for “Application Programming Interface.” Java API is a collection of prewritten packages, classes, and interfaces that provide a set of rules and tools to facilitate interactions between different software components in Java applications.
API Full Form in Java Example
In the context of Java or any programming domain, the term “API” stands for “Application Programming Interface.” An API defines a set of rules, protocols, and tools that allow different software applications to communicate with each other. Here’s a simple example in Java to illustrate the concept of an API.
Let’s consider the ArrayList class from the Java Standard Library, which is part of the java.util package. ArrayList is a resizable array implementation in Java, providing various methods to manipulate and access elements.
import java.util.ArrayList;
public class ArrayListExample {
    public static void main(String[] args) {
        // Create an ArrayList of Strings
        ArrayList<String> fruits = new ArrayList<>();
        // Add elements to the ArrayList
        fruits.add(“Apple”);
        fruits.add(“Banana”);
        fruits.add(“Cherry”);
        // Print the ArrayList
        System.out.println(“ArrayList: ” + fruits);
        // Get the size of the ArrayList
        int size = fruits.size();
        System.out.println(“Size of ArrayList: ” + size);
        // Access elements using index
        String firstFruit = fruits.get(0);
        System.out.println(“First fruit: ” + firstFruit);
        // Remove an element from the ArrayList
        fruits.remove(“Banana”);
        System.out.println(“ArrayList after removing ‘Banana’: ” + fruits);
        // Check if the ArrayList contains a specific element
        boolean containsCherry = fruits.contains(“Cherry”);
        System.out.println(“ArrayList contains ‘Cherry’: ” + containsCherry);
        // Clear the ArrayList
        fruits.clear();
        System.out.println(“ArrayList after clearing: ” + fruits);
    }
}
In this example, the ArrayList class acts as an API, providing various methods like add(), size(), get(), remove(), contains(), and clear() that developers can use to manipulate the list of fruits. By utilizing these methods, developers can perform operations like adding elements, retrieving elements, removing elements, checking the presence of elements, and clearing the list, demonstrating the functionality encapsulated within the ArrayList API in Java.
Also Read: Anagram In Java: Examples, Programs, Solutions
Types of API in Java
In Java, APIs (Application Programming Interfaces) serve as a bridge between different software components, allowing them to interact and communicate with each other. There are several types of APIs in Java based on their functionalities and purposes. Here’s an overview of some common types of APIs in Java:
1) Java Standard APIs:
- Core Java APIs: These are foundational APIs provided by Java’s core libraries, such as java.lang, java.util, java.io, java.net, etc. They offer classes and interfaces for essential functionalities like data structures (lists, sets, maps), file I/O operations, networking, multithreading, and more.
- JavaFX APIs: JavaFX is a platform for creating rich client applications. Its APIs provide classes and interfaces for creating graphical user interfaces (GUIs), handling events, multimedia, and animations.
2) Java Enterprise APIs (Java EE):
- Servlet API: Used for developing web applications in Java, providing classes and interfaces for handling HTTP requests, responses, sessions, and servlet lifecycle management.
- JDBC API: Enables Java applications to interact with relational databases by providing classes and interfaces for database connectivity, executing SQL queries, and managing database resources.
- JPA (Java Persistence API): A part of the Java EE specification, JPA provides a set of interfaces and annotations for object-relational mapping (ORM), allowing Java applications to interact with databases using high-level object-oriented entities.
3) Java Microservices APIs:
- Spring Boot: While Spring Boot is not a part of the Java Standard APIs, it’s a popular framework for building microservices-based applications in Java. Spring Boot provides a comprehensive set of APIs and tools for creating standalone, production-ready applications, including web APIs, data access, security, and more.
- RESTful APIs: Representational State Transfer (REST) is an architectural style for designing networked applications. In Java, developers often use frameworks like Spring Boot to create RESTful APIs that adhere to REST principles, allowing clients to interact with services over HTTP using standard operations like GET, POST, PUT, DELETE.
4) External and Third-party APIs:
- Web Service APIs: Java supports various web service technologies like SOAP (Simple Object Access Protocol), REST, and XML-RPC. Developers can create or consume web services using APIs provided by libraries/frameworks like JAX-WS (Java API for XML Web Services), JAX-RS (Java API for RESTful Web Services), Apache CXF, etc.
- Third-party Libraries and SDKs: Java ecosystem has a vast array of third-party libraries and SDKs that provide specialized APIs for specific functionalities such as JSON processing (Jackson, Gson), HTTP client (OkHttp, Apache HttpClient), logging (Log4j, SLF4J), testing (JUnit, TestNG), and more.
5) Custom APIs:
- Domain-specific APIs: Developers can create custom APIs tailored for specific application domains or business requirements. By defining classes, interfaces, methods, and packages, developers encapsulate functionalities into reusable components, promoting code modularity, and abstraction.
Java offers a diverse range of APIs catering to various application development needs, including core libraries for fundamental functionalities, enterprise APIs for building robust applications, microservices APIs for scalable architectures, external libraries for extended capabilities, and custom APIs for specific domain requirements.
Also Read: Algorithms In Java: List, Programs, Interview Questions
How does API in Java Work?
An API (Application Programming Interface) in Java allows different software applications to communicate with each other. Java provides a rich set of APIs through its class libraries, which developers can use to perform various tasks without having to write code from scratch. Here’s a basic overview of how APIs work in Java:
1) Java Classes and Packages:Â
Java APIs consist of classes and interfaces organized into packages. A package is a namespace that organizes a set of related classes and interfaces. For example, the java.util package contains utility classes and interfaces for data structures like lists, sets, and maps.
2) Importing Packages:Â
To use classes or interfaces from a particular package, you need to import it into your Java code using the import statement. This allows you to access the classes and interfaces without specifying their fully qualified names.
import java.util.ArrayList;
3) Using Classes and Interfaces:Â
Once you’ve imported the necessary packages, you can create objects of the classes, call methods, and use interfaces as needed in your Java application.
import java.util.ArrayList;
public class Example {
    public static void main(String[] args) {
        // Create an ArrayList object
        ArrayList<String> list = new ArrayList<>();
        // Add elements to the list
        list.add(“Java”);
        list.add(“API”);
        list.add(“Example”);
        // Iterate over the list and print elements
        for (String str : list) {
            System.out.println(str);
        }
    }
}
4) Java Standard Library:Â
Java provides a comprehensive Standard Library that includes APIs for various functionalities such as:
- Collections Framework: Provides interfaces (like List, Set, Map) and classes (like ArrayList, HashSet, HashMap) for data storage and manipulation.
- Input/Output (I/O): Offers APIs for reading and writing data to files, streams, and other I/O devices.
- Networking: Allows developers to create networked applications using protocols like HTTP, TCP/IP, and UDP.
- Concurrency: Provides APIs for multithreading and concurrent programming.
5) Custom APIs:Â
Apart from the Java Standard Library, developers can create their own APIs by defining classes, interfaces, and packages. This allows for code modularity, reusability, and abstraction. For instance, you can create a custom API for a specific application domain like banking, healthcare, or e-commerce.
6) Documentation:Â
Java APIs come with comprehensive documentation, often generated using tools like Javadoc. This documentation provides detailed information about classes, interfaces, methods, parameters, return types, exceptions, and usage examples. Developers can refer to this documentation to understand how to use different components of the API effectively.
APIs in Java facilitate modular and reusable code development by providing a set of classes, interfaces, and methods that developers can use to build applications efficiently. By leveraging the Java Standard Library and creating custom APIs, developers can focus on implementing business logic without worrying about low-level details.
Also Read: About Java Language: Features, History, Full Meaning
API Full Form in Javascript
In the context of JavaScript (JS) or generally in computing, “API” stands for “Application Programming Interface.” The term refers to a set of rules, protocols, and tools that allow different software applications or components to communicate with each other.
In the realm of JavaScript:
- Web APIs: Browsers provide built-in APIs that JavaScript can interact with to access web functionalities. These Web APIs include interfaces like the DOM (Document Object Model), XMLHttpRequest (XHR) for making HTTP requests, Fetch API for modern asynchronous operations, and many others. These APIs allow JavaScript code to manipulate web pages, handle events, make network requests, and more.
- External APIs: JavaScript developers frequently interact with external APIs, often through AJAX (Asynchronous JavaScript and XML) requests or the Fetch API. These external APIs can be provided by third-party services or platforms, allowing applications to fetch data, perform operations, or integrate with various online services such as social media platforms, databases, payment gateways, and more.
- Node.js APIs: In the context of Node.js (a runtime environment for executing JavaScript code server-side), APIs refer to the modules and libraries provided by Node.js itself, as well as third-party packages available through npm (Node Package Manager). These APIs enable developers to build server-side applications, handle file operations, create web servers, interact with databases, and perform other server-related tasks using JavaScript.
In JavaScript, the term “API” encompasses a range of interfaces and functionalities that facilitate communication between different software components, whether it’s interacting with web browsers, accessing external services, or building server-side applications using Node.js.
API Full Form in Python
In the context of Python (or generally in computing), “API” stands for “Application Programming Interface.” This term describes a set of rules, protocols, and tools that allows different software applications to communicate with each other. In Python, APIs can refer to:
- Python Standard Library APIs: Python comes with a comprehensive standard library that provides a wide range of built-in modules and packages. These modules offer various functionalities, such as file I/O operations, networking, data manipulation, and more. Developers can leverage these APIs to perform specific tasks without having to write code from scratch.
- External APIs: Python developers often interact with external APIs provided by third-party services or libraries. For example, web APIs allow applications to communicate over the internet, fetching data from servers, sending requests, or integrating with online services like social media platforms, weather services, payment gateways, and more. Python provides libraries like requests for making HTTP requests and interacting with web APIs.
- Custom APIs: In Python, developers can also create their own APIs by defining classes, functions, and modules that expose specific functionalities. These custom APIs allow for code modularity, reusability, and abstraction, enabling developers to build scalable and maintainable applications.
The term “API” in Python refers to a mechanism that facilitates interaction between different software components, whether it’s leveraging built-in functionalities, integrating with external services, or creating custom interfaces for specific application domains.
The Importance Of Using APIs In Java
Using APIs (Application Programming Interfaces) in Java offers several significant benefits and plays a crucial role in modern software development. Here’s a look at the importance of using APIs in Java:
- APIs allow developers to reuse pre-built components and functionalities, saving time and effort. Instead of writing code from scratch for common tasks, developers can leverage existing APIs, such as Java’s Standard Library or third-party libraries, to accelerate development and maintain consistency.
- APIs promote modular development by encapsulating functionalities into reusable components. By defining clear interfaces and contracts, APIs enable developers to create modular, maintainable, and scalable Java applications, facilitating code organization, separation of concerns, and collaboration among teams.
- APIs facilitate interoperability between different software components, systems, and platforms. Java APIs enable applications to communicate and exchange data seamlessly, regardless of underlying technologies, protocols, or architectures. This interoperability promotes integration, connectivity, and compatibility between disparate systems, promoting system cohesion and synergy.
- Using APIs in Java boosts developer productivity by providing a rich set of tools, libraries, and utilities that simplify complex tasks and streamline development workflows. APIs offer built-in functionalities, data structures, algorithms, and patterns that developers can leverage to focus on implementing business logic, solving problems, and delivering value without getting bogged down by low-level details.
- Java’s Standard APIs and frameworks promote standardization and consistency across applications by providing uniform interfaces, conventions, and practices. By adhering to established standards and guidelines, developers can ensure code quality, maintainability, and reliability, facilitating collaboration, code reuse, and knowledge sharing within the Java community.
- APIs in Java, such as concurrency utilities, collections framework, and networking libraries, enable developers to build scalable and performant applications. By leveraging optimized algorithms, data structures, and patterns, Java APIs empower developers to design efficient, responsive, and resource-efficient applications that can handle increasing workloads and user demands.
- Java APIs offer built-in security features, protocols, and best practices to help developers build secure and compliant applications. By providing APIs for encryption, authentication, authorization, and secure communication, Java enables developers to implement robust security measures, protect sensitive data, and adhere to regulatory requirements, ensuring application integrity, confidentiality, and trustworthiness.
The Java API Standard Library
The Java Standard Library API stands as a cornerstone of the Java programming language, contributing significantly to its widespread popularity. With a vast array of over 100 APIs, this library serves diverse purposes ranging from application development to graphics rendering and web service integration.
Designed with developers in mind, these APIs simplify various tasks by offering access to a wide spectrum of functionalities. For instance, they encompass essential utilities like mathematical operations encapsulated within the Math class, as well as more specialized functionalities such as X-Ray for advanced technologies. Moreover, these APIs facilitate seamless integration with external resources, enabling interactions with platforms like Google Maps or social media channels like Twitter.
For developers seeking sophisticated data management solutions, the library provides tools like the ObjectInputStream class. Similarly, for applications requiring offline capabilities, the Service Locator interface offers invaluable assistance. Overall, the Java Standard Library API empowers developers with a plethora of resources, fostering creativity and efficiency in achieving diverse programming objectives.
API Methods in Java
In Java, API methods refer to the public functions and procedures provided by Java classes and interfaces within its standard library or any custom libraries. These methods define the behavior and operations that developers can perform using the respective classes or interfaces. Here are some common categories of API methods in Java:
1) String Methods:Â
The String class in Java provides a wide range of methods for string manipulation, such as:
- charAt(int index): Returns the character at the specified index.
- length(): Returns the length of the string.
- substring(int beginIndex, int endIndex): Returns a new string that is a substring of this string.
- toUpperCase(): Converts all characters in the string to uppercase.
2) Collection Methods:Â
Java’s Collections Framework provides interfaces like List, Set, and Map along with their implementing classes like ArrayList, HashSet, and HashMap. Some common methods include:
- add(E element): Adds the specified element to the collection.
- remove(Object o): Removes the specified element from the collection.
- contains(Object o): Returns true if the collection contains the specified element.
3) File I/O Methods:Â
Java’s I/O (Input/Output) API offers methods to read from and write to files, such as:
- FileInputStream: Provides methods like read(), available().
- FileOutputStream: Provides methods like write(byte[] b), flush().
- BufferedReader: Provides methods like readLine().
- BufferedWriter: Provides methods like write(String s).
4) Networking Methods:Â
Java’s networking API allows developers to create networked applications using classes like Socket, ServerSocket, URLConnection, etc. Some common methods include:
- Socket: Methods like getInputStream(), getOutputStream().
- ServerSocket: Methods like accept().
- URLConnection: Methods like getInputStream(), getOutputStream().
5) Concurrency Methods:Â
Java’s java.util.concurrent package provides classes and interfaces for concurrent programming. Some common methods include:
- Thread: Methods like start(), join().
- ExecutorService: Methods like submit(Runnable task), shutdown().
6) Custom API Methods:Â
When creating custom APIs in Java, developers define their methods within classes and interfaces to expose specific functionalities. These methods can have various access modifiers (public, private, protected) and parameters based on the requirements of the API.
API methods in Java refer to the functions and operations exposed by classes, interfaces, and packages within the Java Standard Library or custom libraries. These methods provide a way for developers to interact with and manipulate data, perform I/O operations, create networked applications, and implement concurrent programming using Java programming language features.
As we wrap up this blog post, we would like to invite you to take your learning further with Physics Wallah’s Decode Java+DSA 1.0 course. This comprehensive course, created by the renowned educator Physics Wallah, is designed to help students master Java and Data Structures and Algorithms. And to make your learning experience even more rewarding, don’t forget to apply the “READER” coupon at checkout for exciting discounts.
For Latest Tech Related Information, Join Our Official Free Telegram Group :Â PW Skills Telegram Group
API Full Form in Java FAQs
What are the 4 types of API?
The four types of APIs are:
Open APIs (Public APIs)
Internal APIs (Private APIs)
Partner APIs (External APIs)
Composite APIs.
What is an API with an example?
An API (Application Programming Interface) defines a set of rules and protocols for building and interacting with software applications. Example: The Google Maps API allows developers to integrate Google Maps functionalities (like displaying maps, routes, and location data) into their applications.
Is JDK an API?
No, JDK (Java Development Kit) is not an API by itself. However, JDK includes the Java API (Application Programming Interface), which consists of classes, interfaces, methods, and packages provided by Java to facilitate application development.
How does the API work?
An API works by defining a set of rules and protocols that enable different software components, applications, or systems to interact and communicate with each other. Developers use API endpoints and methods to send requests, receive responses, exchange data, and perform specific functionalities, facilitating seamless integration and interoperability between software components.