Master the architecture of Spring Boot with our insightful blog. We’ll illustrate key concepts through examples, design patterns, and discuss the significance of a well-structured layered approach!
Architecture of Spring Boot: Several application development frameworks are available, but one of the most popular frameworks when working with Java is the Spring framework. You will be completely fascinated with the Spring framework and its ability to manage applications.
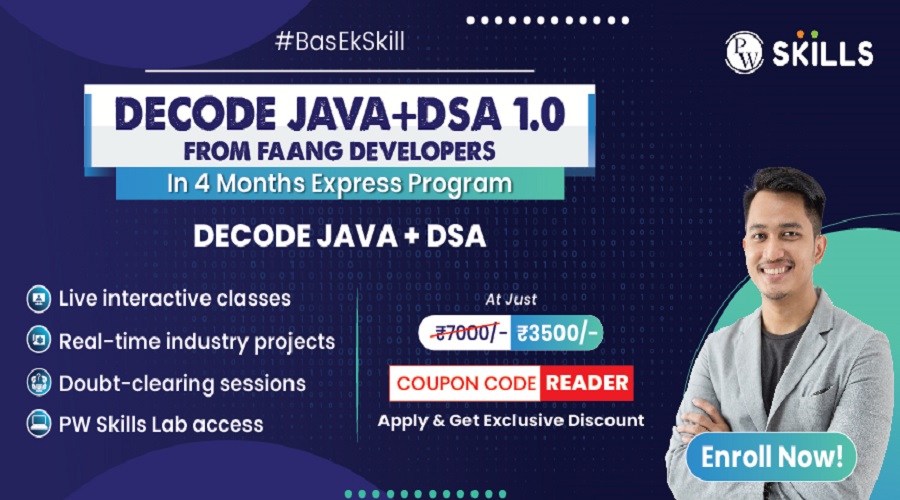
Spring Boot is a Spring Framework module. It is used to quickly construct standalone, production-grade Spring Based Applications. It is built on top of the Spring Framework. Spring Boot has a layered architecture in which each layer communicates with the layer below or above it.
In this article, we will learn about the architecture of Spring Boot. We will also learn about the different layers in the Spring boot architecture. We will also learn about flow in Spring Boot Architecture.
If you want to make a successful career in Full Stack Web Development, the PhysicsWallah’s Full Stack Web Development course is just what you need! Our course will equip you with all the necessary skills and knowledge needed to become a highly successful Full Stack Web Developer in 2024! So, don’t wait! Use the coupon code “READER” and avail an exclusive discount on all PW Skills courses.
Spring Boot Architecture Example
- Controller Layer:
- This layer is responsible for handling incoming HTTP requests.
- Controllers receive requests, process them, and interact with the service layer to retrieve or manipulate data.
- Controllers are often annotated with @Controller or @RestController.
- Service Layer:
- The service layer contains business logic and acts as an intermediary between the controller and the data access layer.
- It encapsulates the application’s business logic, ensuring separation of concerns.
- Services are often annotated with @Service.
- Repository/DAO Layer:
- This layer is responsible for interacting with the database or any external data source.
- It includes interfaces or classes that define methods for performing CRUD (Create, Read, Update, Delete) operations.
- Repositories are often annotated with @Repository.
- Entity Layer:
- Entities represent the application’s data model and are often mapped to database tables.
- These classes typically contain fields annotated with JPA annotations (@Entity, @Column, etc.) for database mapping.
- DTO (Data Transfer Object):
- DTOs are used to transfer data between layers and can help to encapsulate the data being sent between the client and the server.
- They can be used to shape the data for presentation without exposing the internal entity structure.
- Security Layer:
- This layer is responsible for handling authentication and authorization.
- Spring Security is commonly used to secure Spring Boot applications, providing features like authentication, authorization, and protection against common security vulnerabilities.
- Configuration Layer:
- This layer includes configuration files and classes.
- Configuration can be done using annotations like @Configuration, @Bean, or through XML configuration.
Remember that Spring Boot is highly customizable, and the architecture can be adapted based on project requirements. This overview provides a general structure that many Spring Boot applications follow.
Spring Boot Architecture Patterns
Spring Boot applications often follow certain architectural patterns to achieve modularity, scalability, and maintainability. Here are some common architectural patterns used in Spring Boot development:
- MVC (Model-View-Controller):
- Spring Boot follows the MVC pattern for building web applications.
- Model: Represents the application’s data and business logic.
- View: Responsible for rendering the user interface.
- Controller: Handles user requests, interacts with the model, and updates the view.
- Microservices Architecture:
- Spring Boot is commonly used in microservices architectures where the application is decomposed into small, independently deployable services.
- Each microservice is responsible for a specific business capability and communicates with other services through APIs.
- Restful API Design:
- Spring Boot promotes the design of RESTful APIs for communication between components.
- Controllers expose RESTful endpoints, and data is typically exchanged in JSON format.
- Layered Architecture:
- Organising the application into layers like presentation, service, repository, and entity helps maintain a separation of concerns.
- This layered architecture promotes modularity and testability.
- Dependency Injection (DI) / Inversion of Control (IoC):
- Spring Boot relies heavily on the principle of dependency injection, where dependencies are injected into components rather than being created by the components themselves.
- This reduces tight coupling and makes the application more maintainable.
- Event-Driven Architecture:
- Spring Boot supports event-driven architecture through the use of Spring Events.
- Components can publish and subscribe to events, allowing for loosely coupled communication between different parts of the application.
- CQRS (Command Query Responsibility Segregation):
- CQRS separates read and write operations, allowing for independent scaling of the query (read) and command (write) sides.
- Spring Boot can be used to implement CQRS patterns using different components for command and query responsibilities.
- Saga Pattern:
- In microservices architectures, the saga pattern is often used to manage distributed transactions.
- Spring Boot applications can implement sagas by coordinating a series of transactions across multiple microservices.
- Gateway Pattern:
- API gateways are used to manage and route requests in microservices architectures.
- Spring Cloud provides tools like Spring Cloud Gateway for building API gateways.
These patterns can be combined and adapted based on the specific requirements of the application. The flexibility of Spring Boot allows developers to choose and implement the patterns that best suit their needs.
Also Read: Algorithms In Java: List, Programs, Interview Questions
Spring Boot Layered Architecture Example
Let’s walk through a layered architecture example for a Spring Boot application without providing specific code. This example assumes a typical three-layered architecture: Presentation, Service, and Data Access layers.
- Presentation Layer:
-
-
- This layer handles the interaction with the user, receives HTTP requests, and sends responses.
- Controllers in this layer are annotated with @RestController.
- The controllers interact with the Service layer to process requests and obtain results.
- DTOs (Data Transfer Objects) may be used to transfer data between the Presentation and Service layers.
-
- Service Layer:
-
-
- The Service layer contains business logic and orchestrates the flow of data between the Presentation and Data Access layers.
- Service classes are annotated with @Service.
- They use the Data Access layer to retrieve or manipulate data.
- This layer is responsible for enforcing business rules and ensuring data consistency.
-
- Data Access Layer:
-
- The Data Access layer is responsible for interacting with the database or any other data source.
- It includes repository interfaces or classes annotated with @Repository.
- Entities represent the data model and are often annotated with JPA annotations for database mapping.
Example Workflow:
- A user sends an HTTP request to a REST endpoint in the Presentation layer.
- The controller in the Presentation layer receives the request and invokes the appropriate method in the Service layer.
- The Service layer performs business logic, possibly involving multiple service classes, and interacts with the Data Access layer to retrieve or persist data.
- The Data Access layer communicates with the database to perform CRUD operations on entities.
- The Service layer returns the result to the Presentation layer, possibly transforming entities into DTOs.
- The Presentation layer sends an HTTP response to the user.
Benefits:
- Modularity: Each layer has a specific responsibility, making the application more modular and easier to maintain.
- Separation of Concerns: The separation of layers allows for a clear distinction between presentation, business logic, and data access concerns.
- Testability: Each layer can be tested independently, promoting easier unit testing and maintenance.
- Scalability: Components can be scaled independently based on the specific needs of each layer.
Considerations:
- Care should be taken to avoid tight coupling between layers. Dependency injection and interfaces can help achieve loose coupling.
- DTOs may be used to transfer data between layers, but excessive use should be avoided to prevent overcomplicating the architecture.
Remember that this is a high-level overview, and specific project requirements may lead to variations in the architecture.
Architecture of Spring Boot Microservices
The architecture of Spring Boot microservices typically follows principles that align with the characteristics of microservices, such as modularity, independence, and distributed systems. Here’s a conceptual overview of the architecture for a Spring Boot microservices application:
- Microservices:
- The application is decomposed into small, independent, and loosely coupled services, each focused on a specific business capability.
- Microservices communicate with each other through well-defined APIs, often exposed as RESTful endpoints.
- Service Registry and Discovery:
- Services register themselves with a service registry (e.g., Spring Cloud Netflix Eureka).
- Service discovery allows services to locate and communicate with each other dynamically.
- API Gateway:
- An API Gateway (e.g., Spring Cloud Gateway, Netflix Zuul) is used to manage and route external requests to the appropriate microservices.
- It can handle authentication, load balancing, and routing.
- Configuration Management:
- Centralised configuration management (e.g., Spring Cloud Config) allows microservices to retrieve configuration properties dynamically.
- Configuration can be stored in a version-controlled repository and updated without redeploying services.
- Distributed Data Management:
- Microservices often have their own databases, and the choice of database depends on the specific requirements of each service.
- Eventual consistency and distributed data management strategies may be employed.
- Event-Driven Architecture:
- Events and messaging systems (e.g., Spring Cloud Stream, Apache Kafka) are used for communication between microservices.
- Event-driven architecture supports loose coupling and scalability.
- Polyglot Architecture:
- Each microservice can be implemented in a different programming language or use a different technology stack, known as a polyglot architecture.
- The choice of technology is based on the specific requirements of each service.
- Testing and Continuous Integration/Continuous Deployment (CI/CD):
- Microservices are independently testable, allowing for easier unit testing and integration testing.
- CI/CD pipelines enable automated testing and deployment of microservices.
- Domain-Driven Design (DDD):
- Microservices are often designed based on domain-driven design principles, emphasising a clear understanding of the business domain and its interactions.
This architecture allows for flexibility, scalability, and easy maintenance of a microservices-based system. Spring Cloud provides a set of tools and libraries to implement many of these patterns and streamline the development of microservices in a Spring Boot environment.
Also Read: Spring Boot Tutorial
Controller Layer in Spring Boot
In a Spring Boot application, the controller layer is a crucial component responsible for handling incoming HTTP requests, processing them, and producing appropriate responses. It plays a key role in the Model-View-Controller (MVC) architectural pattern. Below are key aspects and responsibilities of the controller layer in Spring Boot:
- Annotation:
- Controllers are typically annotated with @Controller or @RestController. The @RestController annotation is often used for RESTful APIs and includes the @Controller and @ResponseBody annotations.
- Request Mapping:
- Controllers define methods that handle specific HTTP requests. These methods are annotated with @RequestMapping or more specialised annotations like @GetMapping, @PostMapping, etc.
- Request mappings specify the URI path and HTTP method that the method should respond to.
- Method Parameters:
- Controller methods can have parameters that are automatically populated with data from the HTTP request, such as path variables (@PathVariable), request parameters (@RequestParam), request headers (@RequestHeader), and the request body.
- Response Handling:
- Controller methods return data that will be included in the HTTP response. The return type can be a Java object, which is automatically converted to JSON (or another format) using Spring’s content negotiation.
- For RESTful APIs, the @ResponseBody annotation can be used to indicate that the return value should be serialised directly to the response body.
- View Rendering (for MVC):
- In traditional MVC applications, where server-side rendering is used, the controller may return a logical view name that is resolved by the ViewResolver to an actual view template.
- For RESTful APIs, the focus is on data returned as JSON or XML rather than rendering HTML views.
- Exception Handling:
- Controllers can handle exceptions using @ExceptionHandler methods. These methods define how the application should respond when a specific exception occurs during request processing.
- Validation:
- Controllers may perform input validation by using validation annotations such as @Valid in method parameters. Validation errors can be captured and handled in the controller.
- Interception and Filters:
- Controllers can be intercepted using interceptors (HandlerInterceptor) to perform pre- or post-processing logic.
- Filters (javax.servlet.Filter) can also be applied at the servlet level for more fine-grained request processing.
- Cross-Origin Resource Sharing (CORS) Handling:
- For web applications, controllers can configure CORS settings using annotations like @CrossOrigin to control which domains are allowed to access the resources.
- Security:
- Controllers often play a role in handling security aspects such as authentication and authorization. Spring Security can be integrated to secure controller methods.
- Testing:
- Controllers are unit-testable. Spring provides testing support through @WebMvcTest for testing the controller layer in isolation.
The controller layer acts as an interface between the external world (HTTP requests) and the application’s business logic (service layer). It helps in maintaining a clear separation of concerns in the application architecture.
What is Spring Boot Architecture?
Spring Boot follows a modular and opinionated architectural pattern that simplifies the development of production-ready, stand-alone Spring-based applications. It builds on the concepts of the broader Spring Framework and provides defaults for convention over configuration, allowing developers to quickly set up and deploy applications.
Here’s an overview of the key components in the architecture of a Spring Boot application:
- Standalone Application:
- Spring Boot applications are designed to be standalone, meaning they are self-contained and can be run with minimum configuration. They don’t require external servlet containers like Tomcat or Jetty, as they embed an embedded server (typically Tomcat, Jetty, or Undertow).
- Opinionated Defaults:
- Spring Boot provides a set of defaults and conventions for configuration, reducing the need for extensive boilerplate code.
- It aims to minimise the effort required for developers to configure and set up the application, making it easier to get started quickly.
- Spring Framework Core:
- At its core, Spring Boot is built on top of the Spring Framework. It inherits the fundamental concepts of Inversion of Control (IoC), Dependency Injection (DI), and Aspect-Oriented Programming (AOP) from the Spring Framework.
What Is the Production Architecture of Spring Boot?
The production architecture of a Spring Boot application typically involves deploying microservices in containerized environments with orchestration (e.g., Kubernetes), load balancing, and service discovery. Cloud-native principles, such as containerization with Docker, API gateways (e.g., Spring Cloud Gateway), security measures (e.g., OAuth), and continuous integration/deployment (CI/CD) pipelines are employed. Monitoring tools (e.g., Spring Boot Actuator), logging (e.g., SLF4J with Logback), and resilience patterns (e.g., circuit breakers) ensure robustness.
The choice of databases, scalability, and adherence to compliance regulations contribute to a production-ready architecture, providing high availability, fault tolerance, and efficient resource utilisation.
Also Read: Spring Boot Rest API: Rest APIS with Java and Spring Boot
What is the Architecture of Spring Boot Repository?
The architecture of a Spring Boot repository typically involves the use of Spring Data JPA or other data access technologies. Repositories, annotated with @Repository, encapsulate data access logic and connect the application’s service layer to the underlying database. Entities represent the data model, annotated with JPA annotations for database mapping.
The Service layer orchestrates business logic and interacts with repositories to perform CRUD operations. Spring Boot’s auto-configuration simplifies repository setup, and custom repositories may extend CrudRepository or JpaRepository. This architecture supports modular, maintainable, and scalable applications with efficient database interactions.
What is 3 tier Architecture in Spring Boot?
The 3-tier architecture in Spring Boot divides an application into three layers: Presentation, Service, and Data Access. The Presentation layer includes controllers handling user interactions, the Service layer encapsulates business logic, and the Data Access layer manages interactions with the database.
Controllers process HTTP requests, invoking service methods that orchestrate business logic. Service classes interact with repositories or DAOs, which, in turn, perform database operations on entities. This modular architecture enhances maintainability, scalability, and separation of concerns, allowing each layer to be developed and tested independently, fostering a clearer organisation of code and facilitating future modifications.
Spring Boot offers a streamlined architecture for building Java applications. Its key features include auto-configuration, embedded servers, and a simplified setup, making it a popular choice for developing robust and production-ready applications with minimal boilerplate code and configuration.
For Latest Tech Related Information, Join Our Official Free Telegram Group : PW Skills Telegram Group
Architecture of Spring Boot FAQs
What is the key component of Spring Boot architecture?
The core component is the embedded web server that eliminates the need for external servlet containers, making Spring Boot applications standalone and easily deployable.
How does Spring Boot simplify configuration?
Spring Boot simplifies configuration through auto-configuration, providing sensible defaults and reducing the need for extensive manual configuration.
What role does the Spring Boot Actuator play in production?
Spring Boot Actuator provides production-ready features for monitoring and managing applications, including health checks, metrics, and application environment details.
How does Spring Boot support containerization?
Spring Boot applications can be easily containerized using Docker, allowing for consistent deployment across different environments and facilitating cloud-native development.
What is the significance of Spring Boot Starters?
Spring Boot Starters are pre-configured templates that include common dependencies, simplifying dependency management and accelerating the setup of specific functionalities like web applications, data access, and messaging.