AJAX Javascript stands for Asynchronous JavaScript and XML. Contrary to its name, AJAX is not a programming language but rather a combination of technologies.
AJAX Javascript: In today’s fast-paced digital world, where instant gratification has become the norm, website users have no tolerance for slow-loading pages or the need to constantly refresh to see updated content. This is where AJAX (Asynchronous JavaScript and XML) comes into play.Â
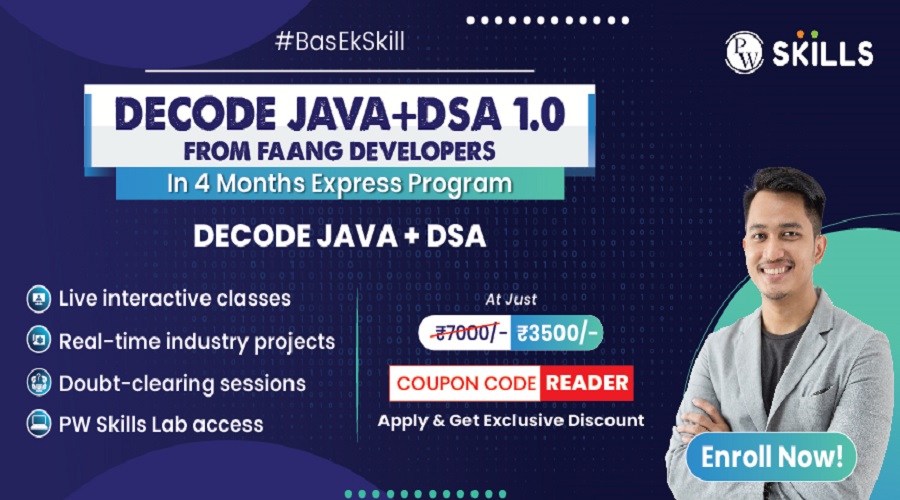
AJAX, a web development technique, allows websites to dynamically update content without the need for a full page reload. By combining the power of JavaScript and XML (though it can also work with JSON or HTML), AJAX enables a smoother and more interactive user experience. In this blog post, we will explore the purpose and benefits of AJAX in JavaScript, and how it has revolutionized web development, ultimately enhancing the overall user experience.
If you want to stay ahead of the game then look no further than Physics Wallah’s Decode Java+DSA 1.0 course.
What is AJAX?
AJAX stands for Asynchronous JavaScript And XML. AJAX is not a programming language; instead, it’s a combination of technologies. Its name, AJAX, can be misleading since it does not solely involve JavaScript and XML. AJAX is a set of web development technologies used to create more responsive web applications.
By leveraging AJAX, web pages can fetch data from the server asynchronously without needing to reload the entire page. The primary benefit of AJAX is enhancing user experience by allowing parts of a web page to update dynamically without requiring a full page refresh.
AJAX Jquery
Using jQuery simplifies the process of making AJAX requests compared to using plain JavaScript. Below is an example demonstrating how to fetch data asynchronously using AJAX with jQuery.
HTML File (index.html)
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<title>AJAX jQuery Example</title>
<script src=”https://code.jquery.com/jquery-3.6.0.min.js”></script> <!– Include jQuery library –>
<script src=”script.js”></script>
</head>
<body>
<h2>Fetching Data Using AJAX with jQuery</h2>
<button id=”fetchDataBtn”>Fetch Data</button>
<div id=”dataContainer”>
  <!– Data will be displayed here –>
</div>
</body>
</html>
JavaScript File (script.js)
// Wait for the document to be fully loaded
$(document).ready(function() {
  // Attach a click event handler to the button
  $(‘#fetchDataBtn’).click(function() {
    // Make an AJAX GET request using jQuery
    $.ajax({
      url: ‘https://jsonplaceholder.typicode.com/todos/1’, // Sample JSON endpoint
      type: ‘GET’, // Request type
      dataType: ‘json’, // Expected data type
      success: function(data) { // Callback function for successful request
        // Display the fetched data on the webpage
        $(‘#dataContainer’).html(`
          <h3>User ID: ${data.userId}</h3>
          <p>Title: ${data.title}</p>
          <p>Completed: ${data.completed ? ‘Yes’ : ‘No’}</p>
        `);
      },
      error: function(error) { // Callback function for request failure
        console.error(‘Error fetching data:’, error);
      }
    });
  });
});
Explanation:
- The index.html file includes the jQuery library via a CDN link and the script.js file.
- Inside the script.js file, the jQuery $(document).ready() function ensures that the script executes after the DOM is fully loaded.
- The jQuery $.ajax() method is used to make an asynchronous GET request to a sample JSON endpoint (https://jsonplaceholder.typicode.com/todos/1).
- If the request is successful, the success callback function displays the fetched data (a sample to-do item) inside the dataContainer div.
- If an error occurs during the request, the error callback function logs the error message to the console.
AJAX Javascript Example
Below is a simple example of how AJAX (Asynchronous JavaScript and XML) works using plain JavaScript to fetch data asynchronously from a server.
HTML File (index.html)
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<title>AJAX Example</title>
<script src=”script.js”></script>
</head>
<body>
<h2>Fetching Data Using AJAX</h2>
<button onclick=”fetchData()”>Fetch Data</button>
<div id=”dataContainer”>
  <!– Data will be displayed here –>
</div>
</body>
</html>
JavaScript File (script.js)
function fetchData() {
  // Create an XMLHttpRequest object
  let xhr = new XMLHttpRequest();
  // Configure the request (GET request to a sample JSON file)
  xhr.open(‘GET’, ‘https://jsonplaceholder.typicode.com/todos/1’, true); // Asynchronous request
  // Define a callback function to handle the response
  xhr.onload = function() {
    if (this.status === 200) {
      // Parse the JSON response
      let data = JSON.parse(this.responseText);
      // Display the fetched data on the webpage
      document.getElementById(‘dataContainer’).innerHTML = `
        <h3>User ID: ${data.userId}</h3>
        <p>Title: ${data.title}</p>
        <p>Completed: ${data.completed ? ‘Yes’ : ‘No’}</p>
      `;
    } else {
      console.error(‘Error fetching data:’, this.status);
    }
  };
  // Handle any errors that may occur
  xhr.onerror = function() {
    console.error(‘Request failed!’);
  };
  // Send the request
  xhr.send();
}
Explanation:
- When you click the “Fetch Data” button on the index.html page, the fetchData() function is invoked.
- Inside the fetchData() function, an instance of XMLHttpRequest is created and configured to make an asynchronous GET request to a sample JSON endpoint (in this case, “https://jsonplaceholder.typicode.com/todos/1”).
- Once the request is successfully completed, the data (a sample to-do item) is parsed from JSON format and displayed on the webpage inside the dataContainer div.
You can run this example by creating the index.html and script.js files, placing them in the same directory, and opening the index.html file in a web browser. When you click the “Fetch Data” button, it will fetch and display the sample to-do item using AJAX.
AJAX Full Form
AJAX stands for Asynchronous JavaScript and XML. However, it’s worth noting that while the term “AJAX” historically referred to “Asynchronous JavaScript and XML,” in modern web development, AJAX is commonly used to describe any asynchronous communication between a web client and server, regardless of the data format being XML, JSON, plain text, or others.
How does AJAX work?
AJAX (Asynchronous JavaScript and XML) is a web development technique that allows web pages to be updated asynchronously by exchanging data with a server in the background. AJAX combines multiple technologies, including JavaScript, XML or JSON for data interchange, and the XMLHttpRequest object to facilitate asynchronous communication. Here’s how AJAX works step-by-step:
1) User Action Trigger:
An AJAX request is typically initiated in response to a user action on a web page, such as clicking a button, submitting a form, or interacting with a specific element that requires data retrieval or updating content dynamically.
2) XMLHttpRequest Object:
Upon triggering a user action, JavaScript creates an instance of the XMLHttpRequest object, which serves as the core component for handling asynchronous HTTP requests and responses between the client and server.
3) Asynchronous Request:
The XMLHttpRequest object facilitates asynchronous communication with the server by sending an HTTP request to a specified URL, method (GET, POST, PUT, DELETE, etc.), and optional parameters (data) based on the user action or requirements.
4) Server-Side Processing:
Upon receiving the AJAX request, the server processes the request, performs necessary operations, such as querying a database, processing data, or executing server-side logic, and generates an appropriate response, typically in XML, JSON, HTML, or plain text format.
5) Asynchronous Response Handling:
Once the server processes the request and prepares the response, the XMLHttpRequest object asynchronously receives the response without requiring a page reload, triggering an event to handle the received data, status, and headers.
6) Data Manipulation and Update:
Upon receiving the server’s response, JavaScript processes the data (XML, JSON, HTML, etc.) within the client-side application, extracts relevant information, and dynamically updates specific elements, content, or components of the web page using the Document Object Model (DOM) manipulation techniques.
7) User Interface Refresh:
After updating the content or elements on the web page based on the server’s response, the user interface refreshes or displays the updated information, providing real-time feedback, dynamic content loading, and seamless user interaction without requiring full page reloads or interruptions.
Also Read: What is Java API? Definition, Types, & Uses
Purpose of AJAX in JavaScript
The primary purpose of AJAX (Asynchronous JavaScript and XML) in JavaScript-based web development is to enhance the user experience by enabling asynchronous data exchange between the client and server, without requiring a full page reload. AJAX facilitates the creation of dynamic, interactive, and responsive web applications by allowing specific portions of a web page to be updated asynchronously, thereby improving performance, usability, and interactivity. Here are some key purposes and benefits of using AJAX in JavaScript:
1) Asynchronous Data Retrieval:
- Efficient Data Exchange: AJAX enables asynchronous data retrieval from servers or databases, allowing web pages to load and display content dynamically without refreshing the entire page.
- Optimized Performance: By fetching only necessary data or content, AJAX minimizes server load, reduces bandwidth usage, and improves application performance, ensuring faster response times and smoother user interactions.
2) Dynamic Content Updating:
- Partial Page Updates: AJAX facilitates partial page updates by dynamically updating specific sections or elements of a web page based on user actions, such as form submissions, search queries, or interactions, without reloading the entire page.
- Enhanced User Experience: By providing real-time feedback and updates, AJAX enhances user experience and engagement by creating responsive and interactive web interfaces that adapt to user actions and preferences.
3) Seamless User Interaction:
- Interactive User Interfaces: AJAX enables the development of interactive user interfaces with features like auto-complete, infinite scrolling, sortable tables, and modal dialogs, allowing users to interact with content or perform tasks without navigating away from the current page or experiencing interruptions.
- Immediate Feedback: By facilitating asynchronous form submission and validation, AJAX provides immediate feedback on user input, errors, or actions, improving usability and reducing frustration by eliminating unnecessary page reloads.
4) Efficient API Integration:
- API Consumption: AJAX supports seamless integration with various APIs (Application Programming Interfaces), enabling developers to fetch, display, and manipulate data from external sources, services, or third-party platforms efficiently.
- Dynamic Data Presentation: By asynchronously retrieving and processing data from APIs, AJAX enables dynamic content presentation, enabling developers to create personalized and context-aware web applications that adapt to user preferences, behavior, or criteria.
5) Modular and Scalable Development:
- Modular Components: AJAX promotes modular development by separating data retrieval and presentation layers, allowing developers to create reusable components, improve code maintainability, and facilitate collaborative development.
- Scalable Applications: By leveraging AJAX for asynchronous data exchange and dynamic content loading, developers can build scalable and responsive web applications capable of handling increased user loads, data processing requirements, and evolving business needs.
Uses of AJAX Javascript
AJAX (Asynchronous JavaScript and XML) is a powerful web development technique that utilizes JavaScript to create interactive and dynamic web applications. Here are some common uses and applications of AJAX in JavaScript-based web development:
1) Dynamic Content Loading:
- Partial Page Updates: AJAX enables partial page updates by fetching and displaying specific data or content without reloading the entire web page, enhancing user experience and interactivity.
- Infinite Scrolling: By asynchronously loading additional content as users scroll through a web page, AJAX facilitates infinite scrolling functionality, allowing seamless navigation and exploration of content.
2) Form Submission and Validation:
- Asynchronous Form Submission: AJAX facilitates asynchronous form submission, enabling users to submit form data without refreshing the page, providing real-time feedback, and improving usability.
- Dynamic Form Validation: By utilizing AJAX, developers can implement dynamic form validation, validating user input on-the-fly and providing instant feedback on errors or validation issues without requiring page reloads.
3) Auto-complete and Search Suggestions:
- Real-time Search Suggestions: AJAX enables the implementation of real-time search suggestions or auto-complete functionality, retrieving and displaying relevant search results or suggestions as users type in search queries, enhancing search efficiency and user experience.
- Filtered Data Retrieval: By asynchronously fetching filtered data or search results based on user input, AJAX facilitates dynamic content presentation, displaying relevant information or content based on user preferences or criteria.
4) Interactive User Interfaces:
- Modal Windows and Dialogs: AJAX supports the creation of interactive modal windows, dialogs, or pop-up windows, enabling users to perform specific actions or tasks within the context of the current page without navigating away or reloading the page.
- Sortable and Filterable Tables: By utilizing AJAX, developers can implement sortable and filterable tables or lists, allowing users to interactively sort, filter, and navigate large datasets or content collections without page refreshes.
5) Data Retrieval from APIs and Services:
- API Integration: AJAX facilitates seamless integration with various APIs (Application Programming Interfaces), enabling developers to fetch, display, and manipulate data from external sources, services, or third-party platforms efficiently.
- Asynchronous Data Loading: By asynchronously retrieving data from servers or databases, AJAX enhances application responsiveness and performance, enabling users to interact with content or services without experiencing delays or interruptions.
AJAX in JavaScript-based web development offers a wide range of uses and applications, including dynamic content loading, form submission and validation, real-time search suggestions, interactive user interfaces, and data retrieval from APIs and services. By leveraging AJAX techniques and functionalities, developers can create responsive, interactive, and efficient web applications that enhance user experience, usability, and functionality across various devices and platforms.
Also Read: 10 Most Popular Java Applications in 2024
Advantages of AJAX Javascript
AJAX (Asynchronous JavaScript and XML) is a web development technique used to create interactive and dynamic web applications. When combined with JavaScript, AJAX offers several advantages that enhance user experience, improve performance, and simplify development. Here are some key advantages of using AJAX with JavaScript:
1) Enhanced User Experience:
- Asynchronous Requests: AJAX enables asynchronous data retrieval from a server without refreshing the entire web page, allowing users to interact with the application seamlessly.
- Dynamic Content Loading: By fetching data asynchronously, AJAX enables dynamic content loading, enhancing user interaction by updating specific parts of a web page without reloading the entire page.
2) Improved Performance:
- Reduced Server Load: AJAX minimizes server load by retrieving only necessary data or content, reducing bandwidth usage and improving server performance.
- Faster Response Times: Asynchronous requests facilitate faster response times as only essential data is exchanged between the client and server, optimizing application performance.
3) Responsive User Interfaces:
- Interactive Elements: AJAX enables the creation of interactive user interfaces with real-time feedback, enabling users to perform actions like form submissions, search queries, or data updates without page reloads.
- Progressive Enhancement: By implementing AJAX progressively, developers can enhance the user experience for modern browsers while maintaining basic functionality for older browsers, ensuring broad compatibility and accessibility.
4) Modular Development:
- Reusable Components: AJAX promotes modular development by separating data retrieval and presentation layers, allowing developers to create reusable components and improve code maintainability.
- Scalability: By leveraging AJAX for data retrieval and manipulation, developers can build scalable applications capable of handling increased user loads and data processing requirements.
5) Seamless Integration with APIs:
- API Consumption: AJAX facilitates seamless integration with various APIs (Application Programming Interfaces), enabling developers to fetch data from external sources, services, or third-party platforms efficiently.
- Data Exchange Formats: AJAX supports multiple data exchange formats like JSON (JavaScript Object Notation) and XML (eXtensible Markup Language), providing flexibility in handling and processing data from diverse sources or services.
Ajax GET and Post Request
An AJAX GET request is primarily used to retrieve data from a server asynchronously without reloading the entire web page. It’s suitable for fetching information like text, JSON, XML, or other formats from a server.
Lifecycle and readyState:
When making an AJAX GET request using XMLHttpRequest, you monitor the request’s progress through its lifecycle using the readyState property:
- 0 (UNSENT): The request is not yet initialized.
- 1 (OPENED): The request has been set up with the open() method.
- 2 (HEADERS_RECEIVED): The open() method has been invoked, and the headers and status are available.
- 3 (LOADING): The server is processing the request.
- 4 (DONE): The request is complete, and the response is ready.
The on ready state change event triggers whenever the readyState changes, allowing you to manage the request’s lifecycle and respond appropriately at each stage.
Also Read: Architecture Of Spring Boot – Examples, Patterns, Diagram
HTTP Status Codes:
Upon completion of the AJAX GET request, the server’s response includes an HTTP status code:
- 200 OK: The server processed the request successfully.
- 404 Not Found: The server cannot locate the requested resource.
- 503 Service Unavailable: The server is temporarily unable to handle the request.
AJAX POST Request
An AJAX POST request is employed to submit data to a server asynchronously. Unlike the GET method, which appends data to the URL, POST sends data in the body of the HTTP request, making it suitable for sending form data, files, or structured data to a server.
Form Data Submission:
To send form data using AJAX POST, you can utilize the FormData object or construct a query string with key-value pairs. When using a query string, it’s essential to set the appropriate content type header to application/x-www-form-urlencoded using setRequestHeader() before invoking the send() method.
Request Headers:
The setRequestHeader() method allows you to set specific headers before sending an AJAX request. Commonly used request headers include:
- text/html: For HTML content.
- text/plain: For plain text content.
- application/xml: For XML content.
- application/json: For JSON content.
Implementation:
Here’s a basic outline illustrating the implementation of AJAX GET and POST requests using XMLHttpRequest:
// AJAX GET Request
let xhrGet = new XMLHttpRequest();
xhrGet.open(‘GET’, ‘https://api.example.com/data’, true);
xhrGet.onreadystatechange = function() {
  if (this.readyState === 4 && this.status === 200) {
    // Process the response data
    console.log(JSON.parse(this.responseText));
  }
};
xhrGet.send();
// AJAX POST Request
let xhrPost = new XMLHttpRequest();
xhrPost.open(‘POST’, ‘https://api.example.com/submit’, true);
xhrPost.setRequestHeader(‘Content-type’, ‘application/x-www-form-urlencoded’);
xhrPost.onreadystatechange = function() {
  if (this.readyState === 4 && this.status === 200) {
    // Process the response data
    console.log(this.responseText);
  }
};
let formData = ‘username=user&password=pass’; // Example form data
xhrPost.send(formData);
It is crucial for developers to continue pushing the boundaries of what AJAX can do, constantly seeking ways to improve user experiences and enhance connectivity. And that is why we highly recommend Physics Wallah’s course Decode Java+DSA 1.0. Not only does it delve into the intricacies of AJAX and its role in cloud computing, but also provides practical hands-on training to expand your skill set. Plus, by using the “READER” coupon at checkout, you can avail a special discount!
For Latest Tech Related Information, Join Our Official Free Telegram Group : PW Skills Telegram Group
AJAX Javascript FAQs
What is AJAX JavaScript used for?
AJAX JavaScript is used for asynchronous communication between a web browser and server, allowing web pages to update content dynamically without reloading the entire page, thereby enhancing user experience and interactivity.
What is AJAX full form?
AJAX stands for Asynchronous JavaScript and XML.
Why use AJAX instead of JavaScript?
AJAX is not a replacement for JavaScript but rather utilizes JavaScript to enable asynchronous data exchange with the server, facilitating dynamic content updates without page reloads, improving performance, and enhancing user experience on web applications.
Is JavaScript AJAX still used?
Yes, AJAX with JavaScript is still widely used in web development to create interactive, responsive, and efficient web applications by enabling asynchronous data retrieval and submission between the client and server without full page reloads.