1LL In C++ denotes a literal constant of type “long long”, representing the integer value 1. It’s commonly used to specify “long long” data type explicitly. Learn more and get certified as the best C++ programmer.
1LL In C++: In the world of programming, many small intricacies and syntax rules can seem confusing at first. One example in C++ is the ‘1LL’ syntax. For those learning C++ or encountering this for the first time, the meaning may not be readily apparent.Â
However, it refers to an important concept – numeric literals and data types. Through this guide, we will clearly explain what ‘1LL’ means, why it is used, and when you need to use it in your C++ code. Whether you’re a complete beginner or have some C++ experience, understanding data types like long long int, numeric literals, suffixes like ‘LL’, and issues like integer overflow are key to properly writing and understanding C++ programs.
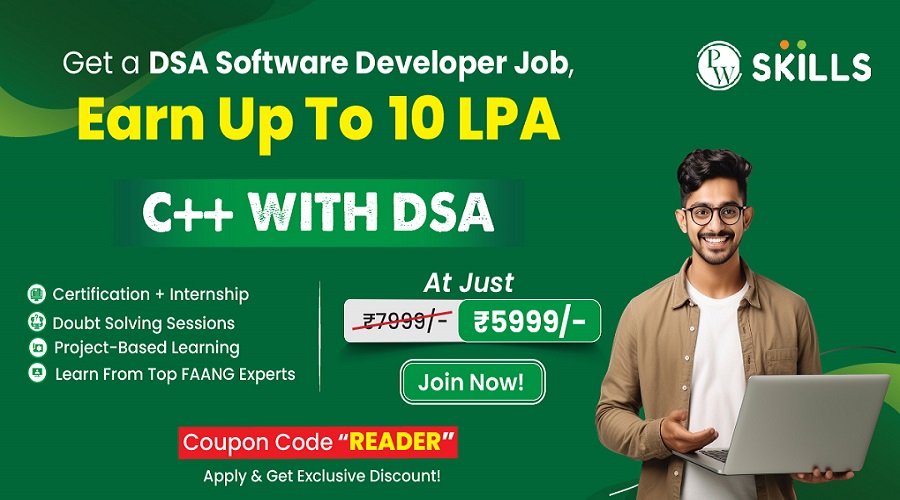
To make it more easy-to-understand you can subscribe to our physics wallas C++ with DSA Course here you’ll have access to the best examples and explanations aimed at the beginner level, we will clarify the purpose of ‘1LL’ and other related compile-time syntax in C++. This will provide the foundation for working with numbers in C++ and shed light on an obscure syntax that often puzzles newer programmers. You can apply coupon code READER to get an exclusive discount on this beginner to advanced course.
By the end, the meaning and purpose of ‘1LL’ will make complete sense and inform your own C++ programming going forward.
1LL In C++ Definition!
In C++, 1LL represents the literal value 1 as a long long type. Let’s break down this definition:
Literal Value: In programming, a literal is a notation for representing a fixed value in source code. In this case, 1 is the literal value representing the integer one.
Type Suffix LL: In C++, suffixes like LL specify the type of the literal. Here, LL indicates that the literal 1 should be treated as a long long type. A long long is an integer type that is guaranteed to be at least 64 bits in size.
Usage: The 1LL notation is often used when working with large integer values or when explicitly needing a long long type instead of the default integer type. It ensures that the value 1 is interpreted as a long long rather than a smaller integer type.
For example:
long long x = 1LL;
In this code snippet, x is declared as a long long variable and initialized with the value 1LL, ensuring that x is explicitly treated as a long long type.
Also Read: 0 in C++: How does the -‘0′ and +’0’ work in C?
1ll In C++ Example
Let’s delve into an example demonstrating the usage of 1LL in C++:
#include <iostream>
int main() {
    long long x = 1LL; // Declare a variable ‘x’ of type long long and assign the value 1LL to it
    std::cout << “Value of x: ” << x << std::endl;
   Â
    return 0;
}
Explanation:
- #include <iostream>: This line includes the input/output stream library, which provides functionality for input and output operations.
- int main() { }: This is the starting point of the C++ program. Execution begins from here.
- long long x = 1LL;: This line declares a variable named x of type long long and initializes it with the value 1LL.Â
Here’s what’s happening:
- long long: long long is a data type in C++ that represents integers with a size of at least 64 bits, providing a wider range of values compared to int or long.
- 1LL: This is a literal value. The LL suffix indicates that the literal should be treated as a long long type. It ensures that 1 is interpreted as a long long rather than a smaller integer type.
- std::cout << “Value of x: ” << x << std::endl;: This line outputs the value of x to the standard output (usually the console). It uses the << operator to concatenate the string “Value of x: ” with the value of x. std::endl is used to insert a newline character and flush the output buffer.
- return 0;: This statement indicates that the program has executed successfully and returns 0 to the operating system, indicating successful termination.
When you run this program, it will output:
Value of x: 1
This demonstrates how 1LL is used to explicitly define the value 1 as a long long integer, allowing for larger integer values or when the context requires a long long type.
2ll in c
In C, the 2LL represents the literal value 2 as a long long type. Let’s illustrate this with an example:
#include <stdio.h>
int main() {
    long long x = 2LL; // Declare a variable ‘x’ of type long long and assign the value 2LL to it
    printf(“Value of x: %lld\n”, x);
   Â
    return 0;
}
Explanation:
- #include <stdio.h>: This line includes the standard input/output library, which provides functionality for input and output operations.
- int main() { }: This is the entry point of the C program. Execution starts from here.
- long long x = 2LL;: This line declares a variable named x of type long long and initializes it with the value 2LL.Â
Here’s what’s happening:
- long long: long long is a data type in C that represents integers with a size of at least 64 bits, providing a wider range of values compared to int or long.
- 2LL: This is a literal value. The LL suffix indicates that the literal should be treated as a long long type. It ensures that 2 is interpreted as a long long rather than a smaller integer type.
- printf(“Value of x: %lld\n”, x);: This line prints the value of x to the standard output (usually the console) using printf function. %lld is a format specifier used for printing long long values.
- 4. return 0;: This statement indicates that the program has executed successfully and returns 0 to the operating system, indicating successful termination.
When you run this program, it will output:
Value of x: 2
This demonstrates how 2LL is used to explicitly define the value 2 as a long long integer in C, allowing for larger integer values or when the context requires a long long type.
Also Read: C Programming Language Syllabus (2024)
0ll in C++
In C++, 0LL represents the literal value 0 as a long long type. Here’s an example demonstrating its usage:
#include <iostream>
int main() {
    long long x = 0LL; // Declare a variable ‘x’ of type long long and assign the value 0LL to it
    std::cout << “Value of x: ” << x << std::endl;
   Â
    return 0;
}
Explanation:
- #include <iostream>: This line includes the input/output stream library, which provides functionality for input and output operations.
- int main() { }: This is the starting point of the C++ program. Execution begins from here.
- long long x = 0LL;: This line declares a variable named x of type long long and initializes it with the value 0LL.Â
Here’s what’s happening:
- long long: long long is a data type in C++ that represents integers with a size of at least 64 bits, providing a wider range of values compared to int or long.
- 0LL: This is a literal value. The LL suffix indicates that the literal should be treated as a long long type. It ensures that 0 is interpreted as a long long rather than a smaller integer type.
- std::cout << “Value of x: ” << x << std::endl;: This line outputs the value of x to the standard output (usually the console). It uses the << operator to concatenate the string “Value of x: ” with the value of x.
- return 0;: This statement indicates that the program has executed successfully and returns 0 to the operating system, indicating successful termination.
When you run this program, it will output:
Value of x: 0
This demonstrates how 0LL is used to explicitly define the value 0 as a long long integer in C++, allowing for larger integer values or when the context requires a long long type.
Also Read: Virtual Function in C++: Everything You Need to Know
cp in c++
In C++, cp itself doesn’t have a specific meaning or usage like 1LL or 0LL. It’s not a predefined keyword or identifier in the C++ language. However, cp might be a variable name chosen by a programmer. Let’s create an example to illustrate how cp could be used as a variable name in a C++ program:
#include <iostream>
#include <string>
int main() {
    // Declare a variable named ‘cp’ of type string and assign it a value
    std::string cp = “C++ Programming”;
   Â
    // Output the value of ‘cp’
    std::cout << “The value of cp: ” << cp << std::endl;
   Â
    return 0;
}
Explanation:
- #include <iostream>: This line includes the input/output stream library, which provides functionality for input and output operations.
- #include <string>: This line includes the string library, which provides functionality for working with strings in C++.
- int main() { }: This is the entry point of the C++ program. Execution starts from here.
- std::string cp = “C++ Programming”;: This line declares a variable named cp of type std::string and initializes it with the value “C++ Programming”.Â
Here’s what’s happening:
- std::string: std::string is a class in the C++ Standard Library that represents a sequence of characters.
- cp: This is the variable name chosen by the programmer. It’s a conventional name but doesn’t have any special meaning to the C++ compiler.
- “C++ Programming”: This is the initial value assigned to the variable cp.
- std::cout << “The value of cp: ” << cp << std::endl;: This line outputs the value of cp to the standard output (usually the console). It uses the << operator to concatenate the string “The value of cp: ” with the value of cp.
- return 0;: This statement indicates that the program has executed successfully and returns 0 to the operating system, indicating successful termination.
When you run this program, it will output:
The value of cp: C++ Programming
This demonstrates how cp can be used as a variable name in C++ to represent a string value. The choice of variable names, like cp, is up to the programmer and should be meaningful and descriptive within the context of the program.
In conclusion, understanding the significance of 1LL in C++ is crucial for any programmer, whether novice or experienced. It represents a literal constant of type “long long,” explicitly indicating a 64-bit integer value of 1. This notation becomes essential when working with large integer values or when precision beyond the default integer type is required. The use of suffixes like LL ensures clarity in data type specification and prevents unintended type conversions.
For those seeking comprehensive knowledge and mastery of C++, our Physics Wallas C++ with DSA Course provides an ideal learning environment. This course covers essential concepts, including numeric literals, data types like long long int, and practical applications of compile-time syntax. Whether you’re a beginner or aiming to advance your skills, our course, designed with clear examples and explanations, offers a structured path to becoming a proficient C++ programmer.
For Latest Tech Related Information, Join Our Official Free Telegram Group : PW Skills Telegram Group
1LL in C++ FAQs
Can I use other suffixes instead of LL for specifying a long long literal?
While LL is the most commonly used suffix for a long long literal, you can also use ll (lowercase Ls) or LLu (for unsigned long long). However, it is recommended to stick to the uppercase LL to maintain consistency and avoid confusion.
What happens if I use 1LL in an operation with other integers of different types?
When performing operations involving 1LL and other integer types, C++ promotes the smaller integer types to the larger one. For instance, if you add 1LL to a regular int, the int will be promoted to a long long for the calculation.
Is there a performance difference between using 1LL and just 1 in my code?
In most scenarios, the performance difference between using 1LL and 1 is negligible. Modern compilers are optimized to handle these situations efficiently. The primary consideration should be the need for a 64-bit integer, not performance implications.
Are there any scenarios where using 1LL may not be necessary?
Yes, for smaller integer values that fit within the range of a 32-bit integer, using just 1 is sufficient. The use of 1LL is essential when dealing with very large numbers that might exceed the limits of a 32-bit integer, preventing potential overflow issues.
Can I mix 1LL with other numeric types in expressions, such as floating-point values?
While it is possible to mix 1LL with other numeric types, keep in mind that the result of the expression will be determined by the type promotion rules. If precision matters, consider explicitly casting or using appropriate type suffixes to avoid unintended type conversions.