Master the art of abstract in Java. Learn more about interfaces, method classes, and understand their significance in the 21st century. Gain clarity on when and why abstraction is a game-changer!
Abstract In Java: Abstract in Java is a class that serves as a blueprint for other classes, allowing them to inherit its properties and methods. It cannot be instantiated on its own, but its subclasses can provide concrete implementations for its abstract methods. This promotes code reusability and enforces a consistent structure across related classes.
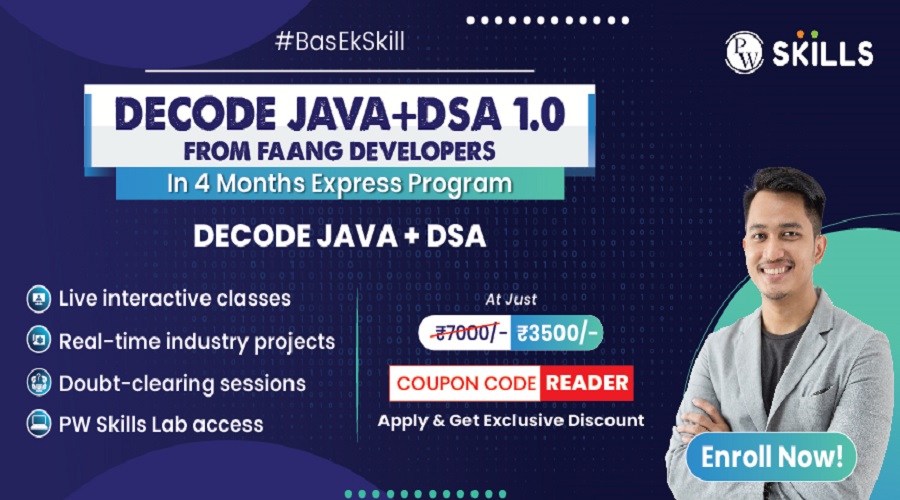
Abstract classes often encapsulate common functionality, facilitating the creation of modular and extensible code. By declaring abstract methods, developers mandate specific behaviours in derived classes, promoting a well-defined interface. Let’s learn more about abstract in Java in this blog.
If you want to pursue an impactful web developer career, PhysicsWallah’s Full Stack Web Development course is highly beneficial. With our course, you’ll always be ahead of your competition and will be equipped with everything you need to succeed as a web developer in 2024. So, don’t wait! Enroll now and use the coupon code “READER” to get an exclusive discount on all courses from PW Skills.
Interface in Java
In Java, an interface is a collection of abstract methods. It defines a contract that concrete classes must adhere to by providing implementations for all the methods declared in the interface. Interfaces allow for the definition of a common set of methods that different classes can implement, promoting code consistency and facilitating polymorphism.
Unlike abstract classes, interfaces can be implemented by multiple classes, enabling a form of multiple inheritance. In addition to abstract methods, interfaces can also include constants and default methods (methods with a default implementation) since Java 8.
Interfaces play a crucial role in achieving abstraction, encapsulation, and creating well-defined contracts between different parts of a Java program.
Abstract Method in Java
Here are the steps to define an abstract in Java:
- Declare the Method:
- Use the abstract keyword in the method signature to indicate that it is an abstract method.
- Abstract methods do not have a body (no implementation within curly braces).
- End the Method with a Semicolon:
- Unlike regular methods, abstract methods end with a semicolon (;) instead of curly braces containing the method body.
- Include the Abstract Keyword in the Class:
- If a class contains at least one abstract method, the class itself must be declared as abstract.
- Use the abstract keyword before the class keyword in the class declaration.
- Provide Concrete Implementation in Subclasses:
- Subclasses that extend the abstract class must provide concrete implementations for all the abstract methods declared in the parent abstract class.
- Failure to do so will result in a compilation error.
- Instantiate Concrete Subclasses:
- You cannot instantiate an object of an abstract class directly.
- Instantiate objects of concrete subclasses that provide implementations for all abstract methods.
By following these steps, you can create an abstract method in Java, ensuring that subclasses provide specific implementations while adhering to the contract established by the abstract class.
Abstract Method in Java Example
Consider a scenario where you are designing a drawing application. You have a base class called Shape that represents various geometric shapes like circles, rectangles, and triangles. However, each specific shape has its own way of calculating the area.
- Abstract Class (Shape):
- Declare an abstract method calculateArea().
- This method is common to all shapes but has no specific implementation in the Shape class.
- Concrete Subclasses (Circle, Rectangle, Triangle):
- Each subclass extends the Shape class.
- Each subclass provides a specific implementation for the abstract method calculateArea().
- For example, the Circle class may use the formula π * r^2 to calculate the area.
- Client Code:
- In your application, you can work with instances of the Shape class, treating them as generic shapes.
- You can call the calculateArea() method on a Shape without knowing the specific type of shape.
This abstraction allows you to represent the commonality among different shapes without specifying the exact details of how each shape calculates its area. Concrete subclasses provide the necessary details when extending the abstract class.
The abstract method acts as a placeholder for functionality that must be implemented by concrete subclasses, ensuring a consistent interface while allowing for variations in behaviour. This approach promotes code flexibility and maintainability in scenarios where shared functionality exists among different classes.
Abstract class vs Interface Java
In Java, both abstract classes and interfaces are mechanisms for achieving abstraction and defining contracts for classes. However, they have some key differences in terms of their features and use cases. Here’s a comparison between abstract classes and interfaces:
Abstract Class
- Keyword: Declared using the abstract keyword.
- Constructor: Can have constructors, and they are called when a concrete subclass is instantiated.
- Fields (Variables): Can have instance variables (fields), which can be either abstract or concrete.
- Access Modifiers: Can have access modifiers (public, private, protected) for methods and fields.
- Method Implementation: Can have both abstract methods (without implementation) and concrete methods (with implementation).
- Single Inheritance: Supports single-class inheritance, meaning a class can extend only one abstract class.
- Version Compatibility: Abstract classes allow the addition of new methods in later versions without breaking existing code.
Also Read: Best Online Platform To Learn Java Programming Course
Interface
- Keyword: Declared using the interface keyword.
- Constructor: Cannot have constructors. Interfaces are meant to declare a contract, not to instantiate objects.
- Fields (Variables): Can only have constants (public static final) fields, which are implicitly public, static, and final.
- Access Modifiers: All methods in an interface are implicitly public and abstract (except for static and default methods in Java 8 and later).
- Method Implementation: Can only declare abstract methods (prior to Java 8) or include static and default methods (Java 8 and later).
- Multiple Inheritance: Supports multiple interface inheritance, meaning a class can implement multiple interfaces.
- Version Compatibility: Adding new methods to an interface can break existing code unless the new methods are implemented in the implementing classes.
When to Use
- Use abstract classes when you want to provide a common base class with shared functionality among related classes and when you need to support single inheritance.
- Use interfaces when you want to define a contract for unrelated classes, want to achieve multiple inheritance through interfaces, or when you want to provide a common interface for classes that may have different base classes.
In practice, it’s common to use a combination of abstract classes and interfaces based on the design requirements of your application. Each has its strengths, and the choice depends on the specific needs of your design.
Abstract in C#
In C#An abstract class is a class that cannot be instantiated on its own and often serves as a base class for other classes. Here are some key points about abstract classes in C# without providing actual code:
- Abstract Keyword:
- Abstract classes are declared using the abstract keyword.
- Cannot Be Instantiated:
- You cannot create an instance of an abstract class directly. It is meant to be subclassed by other classes.
- Abstract Methods:
- Abstract classes can contain abstract methods, which are methods without a body. These abstract methods must be implemented by any non-abstract subclass.
- Concrete Methods:
- Abstract classes can also have concrete methods with a defined implementation. Subclasses inherit both the abstract and concrete methods.
- Constructors:
- Abstract classes can have constructors. These constructors are typically used to initialise fields or perform other common tasks.
- Access Modifiers:
- Abstract classes can use access modifiers like public, private, or protected for their methods and fields.
- Single Inheritance:
- C# supports single-class inheritance, meaning a class can inherit from only one base class, whether it’s abstract or not.
- Fields and Properties:
- Abstract classes can have fields and properties that are inherited by subclasses.
- Common Functionality:
- Abstract classes are often used when you want to provide a common base with shared functionality among related classes.
- Polymorphism:
- Abstract classes support polymorphism, allowing code to work with instances of the abstract class and its subclasses through a common interface.
- Version Compatibility:
- Similar to Java, abstract classes in C# allow for the addition of new methods in later versions without breaking existing code.
Abstract classes in C# provide a way to define a common base for classes, enforcing a consistent structure while allowing for specific implementations in subclasses. They support polymorphism and code organisation, making them a powerful tool in object-oriented design.
Also Read: Spring Boot Tutorial
Abstract Class in Java Example
Consider a scenario where you are designing a system to represent vehicles. You have a base class called Vehicle that captures common characteristics shared among different types of vehicles, such as cars, motorcycles, and bicycles.
- Abstract Class (Vehicle):
- Declare an abstract method start().
- Declare an abstract method stop().
- These abstract methods define a contract that all subclasses must adhere to but have no specific implementation in the Vehicle class.
- Concrete Subclasses (Car, Motorcycle, Bicycle):
- Each subclass extends the Vehicle class.
- Each subclass provides a specific implementation for the start() and stop() methods based on the behaviour of that particular type of vehicle.
- For example, a Car may start and stop its engine, while a Bicycle may start and stop pedalling.
- Client Code:
- In your application, you can work with instances of the Vehicle class, treating them as generic vehicles.
- You can call the start() and stop() methods on a Vehicle without knowing the specific type of vehicle.
This abstraction allows you to represent the commonality among different types of vehicles without specifying the exact details of how each type starts or stops. Concrete subclasses provide the necessary details when extending the abstract class.
The abstract class acts as a blueprint for common behaviour, and concrete subclasses fill in the specific details. This approach promotes code flexibility and maintainability in scenarios where shared functionality exists among different classes.
What is an Abstract Method?
An abstract method is a method declared in an abstract class or an interface that does not have an implementation in the class/interface where it is declared. It only includes the method signature (name, return type, and parameters), without providing the actual code or implementation of the method.
The purpose of declaring abstract methods is to define a contract or a blueprint for functionality that must be implemented by any concrete subclass or class that implements the interface. Abstract methods ensure that the subclasses or implementing classes provide their own specific implementation for the declared method, enforcing a consistent structure across different classes.
In an abstract class, abstract methods are declared using the abstract keyword. In an interface, all methods are abstract by default (prior to Java 8), or explicitly declared as such (Java 8 and later).
Also Read: Spring Boot Rest API: Rest APIS with Java and Spring Boot
What is Abstract and Interface in Java?
Abstract Class:
- Declaration: An abstract class is declared using the abstract keyword.
- Instantiation: You cannot create an instance (object) of an abstract class directly. It is meant to be subclassed by other classes.
- Abstract Methods: An abstract class can have abstract methods, which are declared without implementation (using the abstract keyword) in the abstract class. Subclasses must provide concrete implementations for these abstract methods.
- Concrete Methods: Abstract classes can also have concrete methods (methods with actual implementation) that are inherited by the subclasses.
- Purpose: Abstract classes are used to define a common base class with shared functionality among related classes. They provide a way to define a template for other classes while allowing for specific implementations in subclasses.
- Constructors: Abstract classes can have constructors, and they are called when a concrete subclass is instantiated.
Concrete Class:
- Declaration: A concrete class is a regular class without the abstract keyword.
- Instantiation: You can create instances (objects) of a concrete class directly.
- Methods: All methods in a concrete class have actual implementations. Concrete classes may or may not have abstract methods, but if they do, they must provide implementations for those abstract methods.
- Purpose: Concrete classes are used to create objects directly and provide specific implementations for methods. They can be instantiated, and their methods can be called directly.
- Constructors: Concrete classes can have constructors, and they are called when an object of that class is instantiated.
Abstract classes are meant to be subclassed and provide a way to share common functionality among related classes, while concrete classes are used for direct instantiation and provide specific implementations for their methods. Abstract classes can have a mix of abstract and concrete methods, whereas concrete classes have only concrete methods.
Also Read: Java OOPs Concepts
What is the difference between Abstraction and Abstract Class in Java?
Abstraction
- Definition: Abstraction is a broader concept that refers to the process of simplifying complex systems by modelling classes based on the essential properties and behaviours relevant to the problem at hand, while ignoring the irrelevant details.
- Purpose: The goal of abstraction is to focus on the essential aspects of an object or system, hiding the unnecessary details. It helps in managing complexity, improving understanding, and facilitating code maintenance.
- Examples: Encapsulation, inheritance, and polymorphism are all aspects of abstraction. Using abstract data types, interfaces, and design patterns are also examples of applying abstraction in software design.
Abstract Class
- Definition: An abstract class in Java is a class that cannot be instantiated on its own and may contain both abstract (unimplemented) and concrete (implemented) methods.
- Purpose: The purpose of an abstract class is to serve as a blueprint for other classes. It provides a common base with shared functionality among related classes while allowing specific implementations to be defined in its subclasses.
- Declaration: Abstract classes are declared using the abstract keyword. They may have abstract methods, which are methods without implementation, and concrete methods, which have actual implementations.
Also Read: How to Start Learn to Code in Java, Basics, Tips, and Strategies
Abstract in Java is a broader concept, referring to simplifying complex systems by modelling essential properties. An abstract class, specifically in the context of Java programming, is a class marked with the abstract keyword, serving as a blueprint for subclasses.
Abstract classes allow the definition of common structures and behaviours, incorporating both abstract (unimplemented) and concrete (implemented) methods. Through abstraction and abstract classes, Java facilitates code organisation, reusability, and the creation of well-defined class hierarchies, enabling developers to manage complexity and build modular, extensible software systems.
Abstract in Java FAQs
What is an abstract class in Java?
An abstract class is a class marked with the abstract keyword, serving as a blueprint for other classes. It can contain both abstract (unimplemented) and concrete (implemented) methods.
How does abstraction differ from an abstract class?
Abstraction is a broader concept, simplifying complex systems. An abstract class, in Java, is a specific implementation of abstraction, providing a common base with shared functionality for subclasses.
Can an abstract class have constructors?
Yes, abstract classes in Java can have constructors. These constructors are typically used to initialise fields or perform common tasks when a concrete subclass is instantiated.
What's the purpose of abstract methods in Java?
Abstract methods provide a way to declare a method in an abstract class or interface without providing its implementation. Subclasses must provide concrete implementations for these methods.
How does an abstract class support code reusability?
Abstract classes promo code reusability by defining common structures and behaviours shared among related classes. Subclasses extend the abstract class, inheriting and customising its functionality as needed.