Algorithms in Java refer to sequences of finite steps designed to solve specific problems. Java offers various algorithms for tasks like sorting and searching, making it a powerful language for implementing efficient solutions.
Algorithms in Java: Algorithms are the building blocks that power modern technology. As a Java developer, gaining fluency with common algorithms and data structures is critical for career success. From pulling search results on Google to scrolling through photos on Instagram, algorithms quietly work behind the scenes to organize information and improve our digital experiences every day.
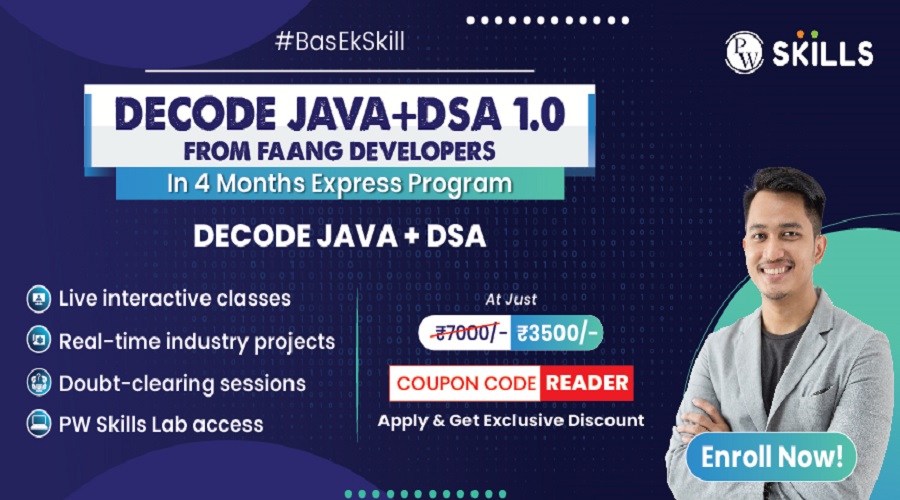
So whether you’re preparing for technical interviews or wanting to take your programming abilities to the next level, keep reading to supercharge your knowledge of algorithms in Java.
With an extensive list of programs and interview questions provided in this blog post, along with the tips and tricks mentioned, you are now equipped with the necessary knowledge to excel in your Java coding journey. And to take your learning to the next level, we highly recommend Physics Wallah’s “Decode Java+DSA 1.0” course. Plus, as a reader of this blog post, you can use our exclusive “READER” coupon code for discounts on the course fee!
List of Algorithms in Java
Java, being a versatile programming language, supports a wide range of algorithms that are fundamental to computer science and software development. Here’s a list of some common algorithms in Java along with a brief explanation of each:
1) Sorting Algorithms:
Bubble Sort:
- Explanation: Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
- Java Implementation: It involves nested loops and swapping elements.
public void bubbleSort(int[] arr) {
    int n = arr.length;
    for (int i = 0; i < n – 1; i++) {
        for (int j = 0; j < n – i – 1; j++) {
            if (arr[j] > arr[j + 1]) {
                // Swap arr[j] and arr[j+1]
                int temp = arr[j];
                arr[j] = arr[j + 1];
                arr[j + 1] = temp;
            }
        }
    }
}
Selection Sort:
- Explanation: Selection Sort divides the input list into two parts: a sorted part and an unsorted part. It repeatedly selects the smallest element from the unsorted part and swaps it with the first unsorted element.
- Java Implementation: Requires looping through elements and finding the minimum value.
public void selectionSort(int[] arr) {
    int n = arr.length;
    for (int i = 0; i < n – 1; i++) {
        int minIndex = i;
        for (int j = i + 1; j < n; j++) {
            if (arr[j] < arr[minIndex]) {
                minIndex = j;
            }
        }
        int temp = arr[minIndex];
        arr[minIndex] = arr[i];
        arr[i] = temp;
    }
}
Insertion Sort:
- Explanation: Insertion Sort builds a sorted array one element at a time. It takes one element from the input data, finds the location it belongs to in the sorted array, and inserts it there.
- Java Implementation: Involves comparing elements and shifting them.
public void insertionSort(int[] arr) {
    int n = arr.length;
    for (int i = 1; i < n; ++i) {
        int key = arr[i];
        int j = i – 1;
        while (j >= 0 && arr[j] > key) {
            arr[j + 1] = arr[j];
            j = j – 1;
        }
        arr[j + 1] = key;
    }
}
Merge Sort:
- Explanation: Merge Sort is a divide-and-conquer algorithm that divides the input array into two halves, sorts them, and then merges the two sorted halves to produce a single sorted array.
- Java Implementation: Uses a recursive approach and merging technique.
public void mergeSort(int[] arr, int l, int r) {
    if (l < r) {
        int m = (l + r) / 2;
        mergeSort(arr, l, m);
        mergeSort(arr, m + 1, r);
        merge(arr, l, m, r);
    }
}
public void merge(int[] arr, int l, int m, int r) {
    // Merge logic here
}
Quick Sort:
- Explanation: Quick Sort is another divide-and-conquer algorithm that picks an element as a pivot and partitions the given array around the pivot, placing the pivot element in its correct position.
- Java Implementation: It’s efficient and works recursively.
public void quickSort(int[] arr, int low, int high) {
    if (low < high) {
        int pi = partition(arr, low, high);
        quickSort(arr, low, pi – 1);
        quickSort(arr, pi + 1, high);
    }
}
public int partition(int[] arr, int low, int high) {
    // Partition logic here
    return 0;
}
2) Searching Algorithms:
Linear Search:
- Explanation: Linear Search sequentially checks each element in the list until the desired element is found or the list ends.
- Java Implementation: Involves a loop to iterate through elements.
public int linearSearch(int[] arr, int x) {
    for (int i = 0; i < arr.length; i++) {
        if (arr[i] == x) {
            return i;
        }
    }
    return -1;
}
Binary Search:
- Explanation: Binary Search works on sorted arrays by repeatedly dividing the search interval in half until the element is found or the interval is empty.
- Java Implementation: It’s more efficient than linear search but requires a sorted array.
public int binarySearch(int[] arr, int x) {
    int low = 0, high = arr.length – 1;
    while (low <= high) {
        int mid = low + (high – low) / 2;
        if (arr[mid] == x) {
            return mid;
        }
        if (arr[mid] < x) {
            low = mid + 1;
        } else {
            high = mid – 1;
        }
    }
    return -1;
}
3) Graph Algorithms:
Breadth-First Search (BFS):
- Explanation: BFS explores all the vertices at the current depth before moving to vertices at the next depth level.
- Java Implementation: Utilizes a queue data structure to keep track of vertices.
Depth-First Search (DFS):
- Explanation: DFS explores as far as possible along each branch before backtracking.
- Java Implementation: Can be implemented recursively or using a stack.
4) Dynamic Programming:
Fibonacci Sequence:
- Explanation: Dynamic programming can efficiently solve problems by breaking them down into smaller sub-problems. The Fibonacci sequence is a classic example where each number is the sum of the two preceding ones.
- Java Implementation: Can use memoization to store computed values.
public int fibonacci(int n) {
    if (n <= 1) {
        return n;
    }
    return fibonacci(n – 1) + fibonacci(n – 2);
}
Algorithms in Java Interview Questions
Here are some common interview questions related to algorithms in Java:
1) What is an algorithm?
An algorithm is a step-by-step procedure or formula to solve a problem. In programming, it’s a set of rules to be followed in calculations or other problem-solving operations.
2) Explain the difference between a stable and an unstable sorting algorithm.
A stable sorting algorithm maintains the relative order of records with equal keys (e.g., in a sorting scenario, if two elements have the same key, their relative order remains unchanged after sorting). An unstable sorting algorithm does not guarantee this relative order.
3) What is the time complexity of the quicksort algorithm?
The average time complexity of the quicksort algorithm is O(n log n), but it can degrade to O(n^2) in the worst-case scenario.
4) How does the Java HashMap work internally?
Internally, a Java HashMap uses an array and a linked list (or a red-black tree after a certain threshold) to store key-value pairs. The hash code of keys determines the index where the value will be stored or retrieved.
5) Explain dynamic programming in Java with an example.
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems and storing their solutions. An example is the Fibonacci sequence calculation using memoization to store previously calculated values to optimize performance.
6) What is the difference between ArrayList and LinkedList in Java?
ArrayList is implemented as a resizable array, providing fast random access and slower insertion/deletion at specific positions. In contrast, LinkedList is implemented as a doubly-linked list, providing fast insertion/deletion but slower random access.
7) How would you detect a cycle in a linked list?
One common approach is to use Floyd’s Cycle-Finding Algorithm (also known as the “tortoise and hare” method), where two pointers traverse the list at different speeds to detect a cycle if they meet at some point.
8) Explain the concept of Big O notation.
Big O notation is used to describe the performance or complexity of an algorithm concerning the input size. It provides an upper bound on the growth rate of an algorithm, helping to understand its efficiency and scalability.
9) What is recursion, and can you provide an example of a recursive function in Java?
Recursion is a programming technique where a function calls itself to solve a problem. An example in Java is the factorial function: int factorial(int n) { return (n <= 1) ? 1 : n * factorial(n – 1); }.
10) How does the Java Arrays.sort() method work, and what is its time complexity?
The Arrays.sort() method in Java uses a modified quicksort algorithm for arrays of objects or a tuned merge sort for arrays of primitives. The time complexity is O(n log n) for both algorithms.
Also Read: Advance Java Tutorial – For Beginners, Core Java vs ADV Java
Basic Java Program Algorithm
Creating a basic Java program involves understanding the fundamental structure and syntax of the Java language. Here’s a step-by-step algorithm to write a simple Java program that displays “Hello, World!” on the console:
1) Initialize Development Environment:
- Ensure that the Java Development Kit (JDK) is installed on your computer.
- Set up an Integrated Development Environment (IDE) like Eclipse, IntelliJ IDEA, or use a text editor like Visual Studio Code to write and run your Java programs.
2) Create a Java Class:
- Open your IDE or text editor and create a new Java file. By convention, the filename should match the class name (with a .java extension).
- For this example, name the file HelloWorld.java.
3) Define the Class:
- Inside the HelloWorld.java file, define a public class named HelloWorld. In Java, every application begins with a class definition.
public class HelloWorld {
    // Code will go here
}
4) Add the Main Method:
- Inside the HelloWorld class, add the main method. The Java application starts executing from the main method.
public static void main(String[] args) {
    // Code will go here
}
5) Print “Hello, World!”:
- Inside the main method, use the System.out.println() method to print “Hello, World!” to the console. This is a built-in Java method used to display output.
public static void main(String[] args) {
    System.out.println(“Hello, World!”); // Display the string
}
6) Save and Compile the Program:
- Save the HelloWorld.java file after adding the code.
- Open a terminal or command prompt and navigate to the directory where the HelloWorld.java file is saved.
- Compile the Java program using the javac command:
javac HelloWorld.java
If there are no errors, this will generate a HelloWorld.class file in the same directory.
7) Run the Program:
- After successfully compiling, run the Java program using the java command:
java HelloWorld
- The output “Hello, World!” will be displayed in the console.
This basic Java program serves as a starting point to understand the foundational concepts of Java programming. As you progress, you can explore more advanced topics, such as variables, data types, control structures, object-oriented programming principles, and more, to build complex and interactive applications using Java.
Also Read:Â Addition Program In Java: Example, Leetcode
Sorting Algorithms in Java
Sorting is a fundamental operation in computer science and programming. In Java, various sorting algorithms are available, each with its advantages, disadvantages, and use cases. Understanding these algorithms helps in selecting the most appropriate one based on the specific requirements of the task at hand.
Common Sorting Algorithms in Java:
1) Bubble Sort:
- Description: This is one of the simplest sorting algorithms. It compares adjacent elements and swaps them if they are in the wrong order.
- Time Complexity: O(n2) in the worst and average cases, O(n) in the best case (when the array is already sorted).
Example Code:
public static void bubbleSort(int[] arr) {
    int n = arr.length;
    for (int i = 0; i < n – 1; i++) {
        for (int j = 0; j < n – i – 1; j++) {
            if (arr[j] > arr[j + 1]) {
                // Swap arr[j] and arr[j+1]
                int temp = arr[j];
                arr[j] = arr[j + 1];
                arr[j + 1] = temp;
            }
        }
    }
}
2) Selection Sort:
- Description: Selection sort divides the input list into two parts: a sorted portion and an unsorted portion. It repeatedly selects the smallest (or largest) element from the unsorted portion and swaps it with the first unsorted element.
- Time Complexity: �(�2)O(n2) in all cases.
Example Code:
public static void selectionSort(int[] arr) {
    int n = arr.length;
    for (int i = 0; i < n – 1; i++) {
        int minIndex = i;
        for (int j = i + 1; j < n; j++) {
            if (arr[j] < arr[minIndex]) {
                minIndex = j;
            }
        }
        // Swap arr[minIndex] and arr[i]
        int temp = arr[minIndex];
        arr[minIndex] = arr[i];
        arr[i] = temp;
    }
}
3) Insertion Sort:
- Description: Insertion sort builds the final sorted array one item at a time. It takes each element from the input list and inserts it into its correct position in the sorted portion of the array.
- Time Complexity: �(�2)O(n2) in the worst and average cases, �(�)O(n) in the best case.
Example Code:
public static void insertionSort(int[] arr) {
    int n = arr.length;
    for (int i = 1; i < n; i++) {
        int key = arr[i];
        int j = i – 1;
        while (j >= 0 && arr[j] > key) {
            arr[j + 1] = arr[j];
            j = j – 1;
        }
        arr[j + 1] = key;
    }
}
When to Use Which Algorithm?
- Bubble Sort: Useful for educational purposes due to its simplicity. Not efficient for large datasets due to its �(�2)O(n2) complexity.
- Selection Sort: Similar to bubble sort in terms of performance. Can be useful for small lists or when memory writes are expensive.
- Insertion Sort: Efficient for small datasets or nearly sorted data. It’s also used in hybrid algorithms like Timsort, which is the default sorting algorithm in Java’s Arrays.sort() method for objects.
While these are some basic sorting algorithms in Java, the Java standard library provides efficient sorting methods like Arrays.sort() and Collections.sort(), which are based on more sophisticated algorithms like Timsort (a hybrid sorting algorithm derived from merge sort and insertion sort). When implementing sorting in Java, it’s crucial to consider the specific requirements like the size of the dataset, stability, and performance characteristics to select the most appropriate algorithm.
Also Read: Abstract In Java – Interface, Examples
Polymorphic Algorithms in Java
Polymorphism is a fundamental concept in object-oriented programming (OOP) that allows objects of different classes to be treated as objects of a common super class. In Java, this is achieved through various mechanisms such as method overriding and method overloading. When it comes to algorithms, polymorphic algorithms provide flexibility by allowing different data types or data structures to be processed uniformly through a single algorithm interface.
At its core, polymorphism allows you to define methods in a superclass and have them be dynamically bound to the appropriate subclass implementations at runtime. This means that a method call on a superclass reference can behave differently based on the actual object it refers to (whether it’s a superclass object or any of its subclasses).
Polymorphic Algorithms
In Java, polymorphic algorithms leverage the power of inheritance and method overriding to create methods that can work on different types of objects. Here’s how it can be achieved:
1) Using Method Overriding:
You can create a method in a superclass and then override that method in one or more subclasses to provide specific implementations. When you call this method using a reference of the superclass, the JVM determines the actual object type at runtime and executes the appropriate overridden method.
class Shape {
    void draw() {
        System.out.println(“Drawing a shape”);
    }
}
class Circle extends Shape {
    @Override
    void draw() {
        System.out.println(“Drawing a circle”);
    }
}
class Square extends Shape {
    @Override
    void draw() {
        System.out.println(“Drawing a square”);
    }
}
public class PolymorphicAlgorithmDemo {
    public static void main(String[] args) {
        Shape shape1 = new Circle();
        Shape shape2 = new Square();
        shape1.draw(); // Output will be “Drawing a circle”
        shape2.draw(); // Output will be “Drawing a square”
    }
}
2) Applying Polymorphic Algorithms:
Algorithms can be designed to work on a superclass type, ensuring they can process any subclass instances that override necessary methods. For example, you might have an algorithm that calculates the area of different shapes without knowing their exact types.
class Shape {
    double area() {
        return 0;
    }
}
class Circle extends Shape {
    double radius;
    Circle(double radius) {
        this.radius = radius;
    }
    @Override
    double area() {
        return Math.PI * radius * radius;
    }
}
class Square extends Shape {
    double side;
    Square(double side) {
        this.side = side;
    }
    @Override
    double area() {
        return side * side;
    }
}
public class AreaCalculator {
    public static void main(String[] args) {
        Shape[] shapes = {new Circle(5), new Square(4)};
        for (Shape shape : shapes) {
            System.out.println(“Area: ” + shape.area());
        }
    }
}
Benefits of Polymorphic Algorithms
- Flexibility: You can write generic algorithms that operate on superclass references but can handle any subclass instances, making the code more adaptable to changes.
- Code Reusability: By leveraging polymorphism, you can reuse algorithms for different types of objects, reducing redundancy and improving maintainability.
- Extensibility: It’s easier to add new subclasses or modify existing ones without altering the algorithms that use them, promoting a more modular design.
Polymorphic algorithms in Java exploit the principles of OOP to create flexible, reusable, and extensible code structures. By designing algorithms that operate on superclass references, you can achieve a higher level of abstraction and adaptability in your Java applications.
With the plethora of interview questions related to algorithms in Java available, you can be well-prepared and confident when appearing for job interviews. But don’t just take our word for it – try decoding Java+DSA 1.0 by Physics Wallah for yourself! With their expert guidance and comprehensive course material, you’ll gain a solid understanding of not only algorithms but also data structures and other important topics in Java.
And as a special treat for our readers, use the coupon code “READER” at checkout to avail exclusive discounts on the course. So what are you waiting for? Take the first step towards mastering algorithms in Java today and witness how it transforms your coding skills into something extraordinary!
For Latest Tech Related Information, Join Our Official Free Telegram Group : PW Skills Telegram Group
Algorithms in Java FAQs
What are the 4 types of algorithm?
The four primary types of algorithms are: Divide and Conquer, Dynamic Programming, Greedy Algorithms, and Recursive Algorithms.
What are the most popular algorithms in Java?
Some popular algorithms in Java include sorting algorithms like QuickSort and MergeSort, searching algorithms like Binary Search, and data structure-related algorithms like BFS (Breadth-First Search) and DFS (Depth-First Search).
What are the basics of algorithms?
The basics of an algorithm include defining a clear set of steps or instructions to solve a particular problem. It should have a definite start and end, be precise, produce a result, and be finite in nature.
What is the algorithm in coding?
In coding, an algorithm is a step-by-step procedure or formula to solve a particular problem. It serves as a blueprint for the computer to execute specific tasks or calculations to achieve the desired outcome.