Analysis of Algorithms in DAA is a crucial component of computational complexity theory, which offers a theoretical approximation of the resources needed by an algorithm to address a particular computing issue. Determining how much time and space are required to execute an algorithm is known as algorithm analysis. Keep reading to learn more!
Analysis of Algorithm In DAA Overview
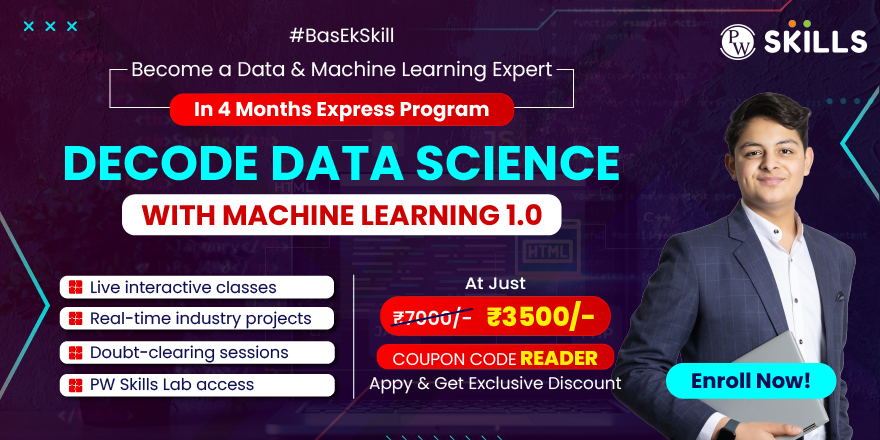
Recommended Technical CourseÂ
- MERN Full Stack Development Course
- Generative AI Course
- System Design Workshop
- Java+DSA 1.0 Course
- Full Stack Web Dev 1.0 Course
- Data Science with ML 1.0 Course
Algorithm analysis in DAA involves evaluating the efficiency and performance of algorithms. It helps in understanding how different algorithms behave under varying conditions.
The analysis of algorithms typically delves into two key aspects: time complexity and space complexity. Time complexity refers to measuring the computational time of an algorithm as a function of input size. It indicates how the runtime scales with an increase in the input size and is often represented using Big O notation.Â
On the other hand, space complexity evaluates the memory usage of an algorithm as a function of input size, showcasing how memory requirements change with different inputs.
A fundamental metric used in Analysis of Algorithms In DAA is Big O notation, providing an upper bound on the growth rate of time and space complexity.
In real-world applications, algorithm analysis influences the selection of algorithms for tasks such as sorting, searching, and data processing. The process aids in identifying the algorithms that can deliver optimal performance based on specific requirements and constraints.
Various tools and libraries are available for algorithm analysis in Python. The time module, profiling tools like cProfile and memory-profiler, and benchmarking tools such as timeit offer valuable insights into an algorithm’s performance. Additionally, NumPy and matplotlib can be utilized for data analysis and visualization, enhancing the overall understanding of algorithm behavior.
Analysis of Algorithm In DAA Overview | |
Aspect of Analysis | Description |
Definition | Algorithm analysis in DAA involves evaluating the efficiency and performance of algorithms. It helps in understanding how different algorithms behave under varying conditions. |
Key Aspects | Focuses on time complexity (runtime) and space complexity (memory usage) of algorithms. |
Time Complexity | Measures computational time as a function of input size, indicating how runtime scales with increasing input. Represented using Big O notation. |
Space Complexity | Evaluates memory usage as a function of input size, indicating how memory requirements change with different inputs. Also represented using Big O notation. |
Notable Metrics | Big O notation provides an upper bound on the growth rate of time and space complexity. Other metrics like Omega (lower bound) and Theta (tight bound) may be used for more precise analysis. |
Importance | Crucial in decision-making for choosing the most efficient algorithms in various applications. Plays a fundamental role in computer science and programming. |
Real-world Application | Influences the choice of algorithms for tasks like sorting, searching, and data processing. |
Tools and Libraries | Python offers tools like the time module, profiling tools (cProfile, memory-profiler), and benchmarking tools (timeit). NumPy and matplotlib aid in data analysis and visualization. |
Considerations | When performing analysis for real-world applications, factors such as input size, data distribution, hardware, and task requirements must be considered. |
Objective | To guide developers in making informed decisions about algorithm selection to achieve optimal performance in different scenarios. |
What is the Analysis of Algorithm In DAA?
In the theoretical algorithms analysis, assessing their complexity in an asymptotic sense is customary, aiming to estimate the complexity function for inputs of arbitrarily large sizes.
Algorithm analysis constitutes a pivotal aspect of computational complexity theory, offering theoretical insights into an algorithm’s requisite resources to solve a specific computational problem. Given that most algorithms are crafted to handle inputs of variable lengths, analyzing algorithms involves determining the time and space resources necessary for their execution.
Typically, the efficiency or runtime of an algorithm is expressed as a function that correlates the input length to the number of steps, referred to as time complexity or the volume of memory consumed, known as space complexity. This analytical approach in Analysis of Algorithm In DAA provides a framework for understanding how algorithms scale with input size, enabling predictions about their performance for increasingly larger datasets.
Analysis of Algorithm in DAA With Example
Example 1: Linear Search vs. Binary Search
Algorithm 1: Linear Search
def linear_search(arr, target):
    for i in range(len(arr)):
        if arr[i] == target:
            return i
    return -1
Algorithm 2: Binary Search (Assumes a sorted array)
def binary_search(arr, target):
    low, high = 0, len(arr) – 1
    while low <= high:
        mid = (low + high) // 2
        if arr[mid] == target:
            return mid
        elif arr[mid] < target:
            low = mid + 1
        else:
            high = mid – 1
    return -1
Analysis:
Time Complexity:
- Linear Search: O(n) (linear time, where n is the size of the array)
- Binary Search: O(log n) (logarithmic time due to the halving of the search space)
Space Complexity:
- Both algorithms have constant space complexity, O(1), using a fixed amount of memory regardless of the input size.
Example Usage:
- Linear search is suitable for small, unsorted arrays.
- Binary search shines in large, sorted datasets.
Example 2: Bubble Sort
Algorithm: Bubble Sort
def bubble_sort(arr):
    n = len(arr)
    for i in range(n):
        for j in range(0, n-i-1):
            if arr[j] > arr[j+1]:
                arr[j], arr[j+1] = arr[j+1], arr[j]
Analysis:
Time Complexity:
- Worst Case: O(n^2) (quadratic time when the array is in reverse order)
- Best Case: O(n) (linear time when the array is already sorted)
Space Complexity:
- O(1) (constant space, as only a temporary variable is used)
Stability:
- Bubble sort is stable, meaning it maintains the relative order of equal elements.
Example Usage:
- Bubble sort is straightforward but less efficient for large datasets. It is commonly used for educational purposes due to its simplicity.
Performance Analysis of Algorithm in DAA
Performance analysis of algorithms in the context of Design and Analysis of Algorithms (DAA) is crucial to evaluate their efficiency and make informed design decisions. Here are the key aspects of performance analysis:
Time Complexity Analysis:
Time complexity is a fundamental metric that quantifies an algorithm’s time to complete concerning the input size. Big O notation is commonly used to express the upper bound on the growth rate of an algorithm’s running time.
Space Complexity Analysis:
Space complexity evaluates the amount of memory an algorithm requires concerning the input size. It includes the analysis of additional data structures, variables, and storage needed during the execution of an algorithm.
Worst, Average, and Best Case Analysis:
Algorithms may behave differently under different scenarios. Performance analysis considers the worst-case, average-case, and best-case scenarios to provide a comprehensive view of the algorithm’s behavior under various conditions.
Asymptotic Analysis:
Asymptotic analysis assesses the efficiency of algorithms for large input sizes. It focuses on how the algorithm behaves as the input approaches infinity. Common notations like Big O, Omega, and Theta express different asymptotic behavior aspects.
Empirical Performance Evaluation:
Beyond theoretical analysis, empirical evaluations involve practical testing and measurement of algorithm performance. This includes implementing algorithms, running real-world or simulated data experiments, and measuring execution times.
Benchmarking:
Benchmarking involves comparing the performance of different algorithms under standardized conditions. It helps identify the most efficient algorithm for a specific problem by running them on the same input instances and comparing their execution times.
Scalability Assessment:
Scalability analysis explores how well an algorithm adapts to increasing input sizes. It assesses whether the algorithm’s performance remains acceptable as the size of the input data grows, a critical consideration in real-world applications.
Trade-off Analysis:
Performance analysis often involves trade-off considerations, such as time and space complexity. It helps make informed decisions about the optimal balance between performance metrics.
Optimization Strategies:
Performance analysis provides insights into potential optimization strategies. By understanding the bottlenecks and inefficiencies in an algorithm, designers can implement targeted optimizations to enhance its overall performance.
Let’s consider a simple example to illustrate the performance analysis of an algorithm in the context of Design and Analysis of Algorithms (DAA). We’ll use the problem of finding the maximum element in an array as our example algorithm.
def find_max_element(arr):
    “””
    Algorithm to find the maximum element in an array.
    “””
    max_element = arr[0] # Assume the first element is the maximum
    for element in arr[1:]:
        if element > max_element:
            max_element = element # Update max_element if a larger element is found
               return max_element
- The time complexity of the find_max_element algorithm is O(n), where n is the size of the input array. This is because, in the worst case, the algorithm needs to iterate through all array elements once.
- The space complexity is O(1), constant space, as the algorithm uses a fixed amount of additional space regardless of the input size. The only variable used is max_element.
- The worst case occurs when the maximum element is at the end of the array, requiring a full traversal. The worst-case time complexity is O(n).
- The average case is also O(n) as we assume no specific distribution of maximum elements.
- The best case occurs when the maximum element is at the beginning, and the algorithm only needs one comparison. The best-case time complexity is O(1).
- The asymptotic behavior is expressed as O(n) for large input sizes.
Also Read Technical Topics
Importance of Analysis of Algorithm In DAA
The analysis of algorithms in the context of Design and Analysis of Algorithms (DAA) holds paramount importance for several reasons:
- Performance Evaluation: It allows for a systematic evaluation of the performance of different algorithms. Analyzing their time and space complexity allows one to compare and contrast algorithms to identify the most efficient solution for a given problem.
- Resource Utilization: Efficient resource utilization is a critical aspect of algorithm design. Analysis helps understand how algorithms consume computational resources such as time and memory, aiding in selecting algorithms that optimize resource usage.
- Predictive Insights: Algorithm analysis provides predictive insights into the behavior of algorithms for varying input sizes. This predictive capability is crucial for anticipating algorithm performance in real-world applications and scenarios.
- Optimization Opportunities: Through analyzing algorithms, one can identify potential optimization opportunities. This may involve tweaking certain algorithm aspects to enhance efficiency or exploring alternative algorithmic approaches.
- Algorithmic Design Choices: The analysis guides algorithmic design choices by offering a quantitative understanding of how different design decisions impact the overall efficiency of an algorithm. It aids in making informed decisions during the design phase.
- Scalability Considerations: As datasets grow in size, the scalability of algorithms becomes a critical factor. Algorithm analysis helps assess how well an algorithm scales with increasing input sizes, guiding the selection of scalable solutions.
- Decision-Making in Software Development: In software development, where resources are often constrained, the analysis of algorithms plays a crucial role in decision-making. It helps developers choose algorithms that align with the performance requirements of the intended application.
- Educational Significance: Understanding algorithmic analysis is fundamental for students and professionals in computer science and related fields. It gives them a structured approach to evaluating and designing algorithms, fostering computational thinking.
Decode Data Science With Machine Learning 1.0 by PW is a comprehensive course designed to equip learners with the skills needed for a successful career in data science. Developed by PW, a renowned platform for quality education, this course stands out as an excellent foundation for individuals interested in mastering the principles of machine learning and data science.
Course Highlights
Comprehensive Curriculum:
- The course covers many topics, providing a solid data science and machine learning foundation.
- From fundamental concepts to advanced techniques, learners understand the subject matter holistically.
Hands-on Learning:
- This course by PW emphasizes practical application through hands-on projects and real-world scenarios.
- Students have the opportunity to work on industry-relevant projects, gaining valuable experience.
Expert Guidance:
- Experienced instructors with expertise in data science and machine learning lead the course.
- Learners benefit from the guidance of industry professionals who provide insights and tips for success.
Algorithmic Thinking:
- The course delves into algorithmic thinking and problem-solving, which are crucial for mastering the Analysis of Algorithms in DAA.
- Students are introduced to various algorithms and learn how to analyze their efficiency.
Integration of DAA Concepts:
- Understanding the importance of algorithm analysis, the course seamlessly integrates concepts of DAA into the broader context of data science.
- Students learn to optimize algorithms for better performance in data-driven applications.
Capstone Projects:
- The program includes capstone projects that challenge learners to apply their knowledge to solve real-world problems.
- This practical experience enhances their ability to analyze algorithms in diverse contexts.
Continuous Learning Support:
- This course provides continuous learning support, ensuring students access to resources, updates, and a supportive community.
Why Is Full Stack Data Science Pro Course By PW Best for Analysis of Algorithms in DAA?
- Relevance to Industry: The course is designed to align with industry requirements, making it highly relevant for individuals seeking roles in data science and machine learning.
- Skill Enhancement: Full Stack Data Science Pro goes beyond theoretical concepts, focusing on skill enhancement to meet the demands of the dynamic field of data science.
- Career Opportunities: Graduates of the course are well-equipped for various career opportunities, ranging from data scientists to machine learning engineers.
- DAA as a Core Competency: Recognizing the significance of algorithm analysis, the course ensures that learners develop DAA as a core competency alongside other data science skills.
Decode Data Science With Machine Learning 1.0 and Generative AI Data Science courses are ideal choices for those aiming to excel in data science, with a strong emphasis on mastering the principles of algorithm analysis in DAA.
Types of Analysis of Algorithm In DAA
Here are the types of analysis of algorithm in DAA:
Types of Analysis of Algorithm In DAA | |
Type of Analysis | Description |
Time Complexity Analysis | Measures the amount of time an algorithm takes to run as a function of the input size.  |
Space Complexity Analysis | Evaluates the amount of memory an algorithm requires relative to the input size. |
Worst Case Analysis | Focuses on the maximum time or space required for any input of a given size.  |
Average Case Analysis | Examines the expected performance over all possible inputs, taking into account probabilities. |
Best Case Analysis | Considers the minimum time or space required for a specific input size.      |
Asymptotic Analysis | Studies the growth rate of the resource requirements for very large input sizes.   |
Big O Notation | Represents the upper bound of an algorithm’s complexity in the worst-case scenario.  |
Omega Notation | Represents the lower bound of an algorithm’s complexity in the best-case scenario. |
Theta Notation | Indicates both the upper and lower bounds of an  algorithm’s complexity, providing a tight bound. |
FAQs
What is the Analysis of Algorithms in DAA?
The Analysis of Algorithms in DAA is the process of evaluating and understanding algorithms' efficiency and performance characteristics. It involves studying how the algorithm's running time and space requirements grow as the input size increases.
Why is Algorithm Analysis critical in DAA?
Algorithm Analysis is crucial in DAA because it helps predict and understand algorithms' resource utilization. It allows us to compare and choose the most efficient algorithm for a specific problem and make informed decisions about algorithmic choices.
What is Time Complexity in Algorithm Analysis?
Time Complexity in Algorithm Analysis measures the time an algorithm takes to complete as a function of the input size. It estimates the worst-case, average-case, or best-case running time using Big O notation.
How is Space Complexity different from Time Complexity?
Space Complexity in Algorithm Analysis assesses the additional memory or space an algorithm requires for input size. While Time Complexity focuses on the time taken, Space Complexity focuses on memory usage.
What is the significance of Worst Case Analysis?
Worst Case Analysis concentrates on determining the maximum time or space required by an algorithm across all possible inputs. It guarantees that the algorithm will perform within a specified bound for any input.
Can an algorithm have different time complexities for different inputs?
Yes, an algorithm can exhibit different time complexities for various inputs. The worst-case, best-case, and average-case scenarios may differ based on the nature of the algorithm and the specific input characteristics.
How is Asymptotic Analysis Functional in Algorithm Analysis?
Asymptotic Analysis helps study the growth rate of an algorithm's time or space complexity as the input size approaches infinity. It provides a high-level understanding of the algorithm's efficiency, commonly expressed using Big O, Omega, and Theta notations.
Is Algorithm Analysis only theoretical, or does it have practical applications?
While Algorithm Analysis has theoretical foundations, it has practical applications. It aids in making informed decisions about algorithm selection, optimizing code, and predicting performance in real-world scenarios.
What is the role of Empirical Performance Evaluation in Algorithm Analysis?
Empirical Performance Evaluation involves executing an algorithm on real-world inputs to measure and analyze its observed performance. It complements theoretical analyses and provides insights into an algorithm's behavior in practical scenarios.
How can Algorithm Analysis contribute to decision-making in software development?
Algorithm Analysis assists in choosing algorithms that align with specific application requirements. It helps developers make decisions about algorithmic efficiency, scalability, and suitability for different use cases.