Learn how Analysis of Algorithms in Data Structure works in this article. Algorithm analysis in the context of data structures provides a nuanced understanding of how algorithms perform in real-world scenarios, considering the intricacies of different data structures. Continue reading to know more in detail!
Analysis of Algorithm in Data Structure: Algorithm analysis in data structures involves a systematic evaluation of how algorithms behave concerning time complexity, space complexity, and practical efficiency when manipulating different types of data. By delving into this realm, computer scientists and developers gain insights into optimizing algorithmic performance, making informed decisions about algorithm selection, and crafting solutions tailored to specific data structure requirements.
This article encompasses diverse aspects, including time complexity analysis, which scrutinizes how the running time of an algorithm changes concerning input size. Space complexity analysis, on the other hand, focuses on the memory requirements and storage usage of algorithms, shedding light on their scalability and resource utilization.
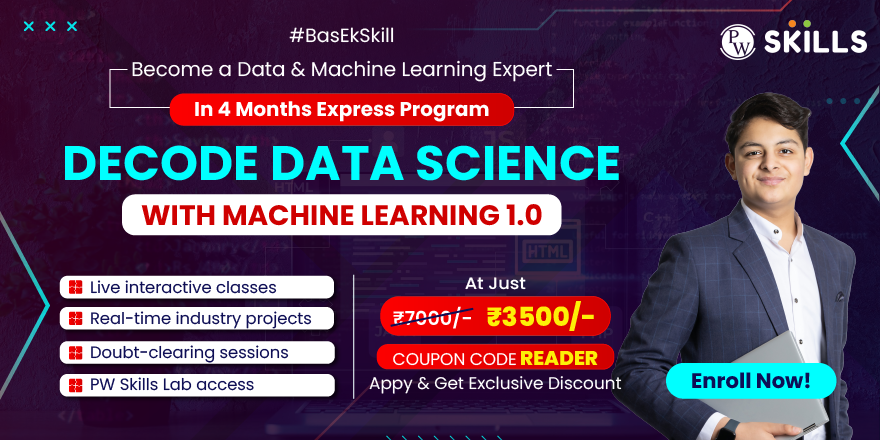
Recommended Technical CourseÂ
- MERN Full Stack Development Course
- Generative AI Course
- System Design Workshop
- Java+DSA 1.0 Course
- Full Stack Web Dev 1.0 Course
- Data Science with ML 1.0 Course
Analysis of Algorithm in Data Structure Overview
Analysis of Algorithms in Data Structure involves evaluating the efficiency and performance of algorithms when applied to various data structures. This analysis is crucial for understanding how well algorithms perform in different scenarios and helps make informed decisions about their usage in specific applications.
Data structure analysis assesses how algorithms interact with and manipulate different data structures. This entails determining the best-case, worst-case, and average-case situations for algorithms, assessing their time and space complexity, and comprehending how they behave with different input quantities.
Developers can choose algorithms with greater knowledge and efficiency, resulting in scalable and more effective software solutions, by thoroughly analyzing algorithms in the context of data structures.
Analysis of Algorithm in Data Structure with Example
Analyzing algorithms in the context of data structures involves evaluating their efficiency and performance when interacting with different data structures. Let’s explore this concept with a couple of examples:
Example 1: Searching Algorithm with Arrays
Consider a simple linear search algorithm applied to an array. In this case, the algorithm aims to find the position of a specific element in the array.
def linear_search(arr, target):
    for i in range(len(arr)):
        if arr[i] == target:
            return i
    return -1
Analysis:
- O(n) in the worst case (linear time), where ‘n’ is the size of the array.
- Space Complexity: O(1), as the algorithm uses constant extra space.
Example 2: Sorting Algorithm with Linked Lists
Let’s take the example of a simple sorting algorithm, such as bubble sort, applied to a linked list.
class Node:
    def __init__(self, data):
        self.data = data
        self.next = None
def bubble_sort_linked_list(head):
    if not head:
        return None
    swapped = True
    while swapped:
        swapped = False
        current = head
        while current.next:
            if current.data > current.next.data:
                current.data, current.next.data = current.next.data, current.data
                swapped = True
            current = current.next
# Example usage:
# (Assuming ‘head’ is the head of a linked list)
# bubble_sort_linked_list(head)
Analysis:
- O(n^2) in the worst case (quadratic time), where ‘n’ is the number of elements in the linked list.
- Space Complexity: O(1) as the algorithm uses constant extra space.
Also read:Â Bubble Sort Algorithm, Code, Advantages
Analysis of Algorithm in Data Structure in C
Analyzing algorithms in data structures using the C programming language involves evaluating their efficiency and performance. Let’s explore some examples to illustrate various types of analysis:
Time Complexity Analysis:
Example: Linear Search
int linearSearch(int arr[], int n, int key) {
    for (int i = 0; i < n; i++) {
        if (arr[i] == key) {
            return i; // Key found at index i
        }
    }
    return -1; // Key not found
}
Time Complexity: O(n) (linear time) – The time taken increases linearly with the size of the input.
Space Complexity Analysis:
Example: Dynamic Array
int* createDynamicArray(int n) {
    int* arr = (int*)malloc(n * sizeof(int));
    return arr;
}
Space Complexity: O(n) – The space required increases linearly with the size of the array.
Worst-Case Analysis:
Example: Bubble Sort
void bubbleSort(int arr[], int n) {
    for (int i = 0; i < n-1; i++) {
        for (int j = 0; j < n-i-1; j++) {
            if (arr[j] > arr[j+1]) {
                // Swap if the element found is greater
                int temp = arr[j];
                arr[j] = arr[j+1];
                arr[j+1] = temp;
            }
        }
    }
}Â
Worst-Case Time Complexity: O(n^2) – The algorithm performs poorly when the array is in reverse order.
Best-Case Analysis:
Example: Insertion Sort
void insertionSort(int arr[], int n) {
    for (int i = 1; i < n; i++) {
        int key = arr[i];
        int j = i – 1;
        while (j >= 0 && arr[j] > key) {
            arr[j+1] = arr[j];
            j = j – 1;
        }
        arr[j+1] = key;
    }
}
Best-Case Time Complexity: O(n) – The algorithm performs well when the array is already sorted.
Average-Case Analysis:
Example: Quick Sort
int partition(int arr[], int low, int high) {
    int pivot = arr[high];
    int i = low – 1;
    for (int j = low; j <= high-1; j++) {
        if (arr[j] < pivot) {
            i++;
            // Swap arr[i] and arr[j]
            int temp = arr[i];
            arr[i] = arr[j];
            arr[j] = temp;
        }
    }
    // Swap arr[i+1] and arr[high] (pivot)
    int temp = arr[i+1];
    arr[i+1] = arr[high];
    arr[high] = temp;
    return i+1;
}
void quickSort(int arr[], int low, int high) {
    if (low < high) {
        int pi = partition(arr, low, high);
        quickSort(arr, low, pi – 1);
        quickSort(arr, pi + 1, high);
    }
}
Average-Case Time Complexity: O(n log n) – On average, the algorithm performs efficiently.
Performance Analysis of Algorithm in Data Structure
Performance analysis of algorithms in data structures involves evaluating how well an algorithm performs in terms of time and space. Let’s delve into an example to illustrate performance analysis:
Example: Merge Sort
#include <stdio.h>
void merge(int arr[], int left, int mid, int right)Â
{
    int n1 = mid – left + 1;
    int n2 = right – mid;
    // Create temporary arrays
    int L[n1], R[n2];
    // Copy data to temporary arrays L[] and R[]
    for (int i = 0; i < n1; i++)
        L[i] = arr[left + i];
    for (int j = 0; j < n2; j++)
        R[j] = arr[mid + 1 + j];
    // Merge the temporary arrays back into arr[left…right]
    int i = 0, j = 0, k = left;
    while (i < n1 && j < n2) {
        if (L[i] <= R[j]) {
            arr[k] = L[i];
            i++;
        }Â
else {
            arr[k] = R[j];
            j++;
        }
        k++;
    }
    // Copy the remaining elements of L[], if there are any
    while (i < n1) {
        arr[k] = L[i];
        i++;
        k++;
    }
    // Copy the remaining elements of R[], if there are any
    while (j < n2) {
        arr[k] = R[j];
        j++;
        k++;
    }
}
void mergeSort(int arr[], int left, int right) {
    if (left < right) {
        // Same as (left+right)/2, but avoids overflow for large left and right
        int mid = left + (right – left) / 2;
        // Sort first and second halves
        mergeSort(arr, left, mid);
        mergeSort(arr, mid + 1, right);
        // Merge the sorted halves
        merge(arr, left, mid, right);
    }
}
int main() {
    int arr[] = {12, 11, 13, 5, 6, 7};
    int arr_size = sizeof(arr) / sizeof(arr[0]);
    printf(“Given array is \n”);
    for (int i = 0; i < arr_size; i++)
        printf(“%d “, arr[i]);
    printf(“\n”);
    // Perform Merge Sort
    mergeSort(arr, 0, arr_size – 1);
    printf(“Sorted array is \n”);
    for (int i = 0; i < arr_size; i++)
        printf(“%d “, arr[i]);
    printf(“\n”);
    return 0;
}
Performance Analysis:
- Time Complexity: Merge Sort has a time complexity of O(n log n) in all cases (worst-case, average-case, and best-case). This makes it highly efficient for large datasets.
- Space Complexity: The space complexity of Merge Sort is O(n) due to the additional space required for temporary arrays during the merging process. This makes it a good choice for scenarios with limited memory.
- Stability: Merge Sort is a stable sorting algorithm, meaning that it maintains the relative order of equal elements in the sorted output. This is advantageous in situations where the original order of equal elements needs to be preserved.
Also read:Â 7 Data Structure for Java That Java Programmers Need to Know in 2024
Goals Of Analysis of Algorithm in Data Structure
Analyzing algorithms in the context of data structures serves several crucial goals, aiming to understand and enhance the efficiency of algorithms when dealing with different data structures. Here are the key goals:
Efficiency Evaluation:
- Objective: Assess how well an algorithm performs regarding time and space.
- Goal: Identify algorithms that can handle large datasets or complex structures efficiently.
Resource Utilization:
- Objective: Determine the optimal use of resources (time and memory) during algorithm execution.
- Goal: Minimize resource consumption while achieving the desired outcome.
Performance Comparison:
- Objective: Compare the efficiency of different algorithms when applied to the same or similar problems.
- Goal: Select the most suitable algorithm for a specific task based on performance metrics.
Scalability Assessment:
- Objective: Understand how an algorithm’s performance scales with the input size.
- Goal: Ensure the algorithm remains efficient as the data structure size grows.
Identifying Bottlenecks:
- Objective: Pinpoint areas where an algorithm may experience inefficiencies or performance bottlenecks.
- Goal: Optimize specific sections of the algorithm to enhance overall efficiency.
Algorithm Design Improvement:
- Objective: Enhance the design of algorithms to make them more adaptive to different data structures.
- Goal: Develop algorithms that are versatile and can handle diverse datasets with optimal efficiency.
Algorithmic Choices:
- Objective: Facilitate informed decision-making when selecting algorithms for specific applications.
- Goal: Choose algorithms based on their performance characteristics and suitability for the given data structure.
Predictive Analysis:
- Objective: Predict the behavior of an algorithm under various conditions and inputs.
- Goal: Anticipate and mitigate potential performance issues before deployment.
Resource Allocation Optimization:
- Objective: Optimize the allocation of computational resources to improve overall system performance.
- Goal: Strike a balance between time and space complexity to achieve optimal efficiency.
Algorithmic Adaptability:
- Objective: Ensure that algorithms can seamlessly adapt to different data structures.
- Goal: Develop algorithms that are versatile and can accommodate diverse data scenarios.
Physics Wallah’s “Decode Data Science With Machine Learning 1.0” course is a comprehensive journey into the world of data science and machine learning.Â
Key Features:
- Algorithmic Thinking: The course instills algorithmic thinking by exploring various machine learning algorithms, enhancing the ability to analyze and optimize algorithms in diverse data scenarios.
- Data Handling Techniques: Learners acquire skills in efficient data handling, preprocessing, and manipulation—essential aspects for algorithm analysis within different data structures.
- Scalability: Understanding the scalability of machine learning models lays the groundwork for analyzing algorithms in data structures, ensuring efficiency as data volumes increase.
How To Compare Analysis of Algorithms in Data Structure?
Evaluating the performance of algorithms in data structures through measures like scalability, space complexity, and time complexity is a crucial part of comparing their analysis. Let’s go over a basic example of comparing two algorithms in the context of a common data structure: finding an element in an array. One algorithm is optimized for time efficiency, while the other is targeted for space efficiency.
Algorithm 1: Linear Search
def linear_search(arr, target):
    for i in range(len(arr)):
        if arr[i] == target:
            return i
    return -1
Algorithm 2: Binary Search
def binary_search(arr, target):
    low, high = 0, len(arr) – 1
    while low <= high:
        mid = (low + high) // 2
        if arr[mid] == target:
            return mid
        elif arr[mid] < target:
            low = mid + 1
        else:
            high = mid – 1 Â
    return -1
Now, let’s compare these algorithms:
Time Complexity:
- Linear Search: O(n) – Linear time complexity, where ‘n’ is the size of the array.
- Binary Search: O(log n) – Logarithmic time complexity due to the halving of the search space.
Space Complexity:
- Linear Search: O(1) – Constant space complexity, as it uses a fixed amount of memory.
- Binary Search: O(1) – Constant space complexity, as it does not use additional space proportional to the input.
Scalability:
- Linear Search: Performs well for small arrays but becomes inefficient as the array size increases.
- Binary Search: Scales efficiently for large sorted arrays, making it suitable for extensive datasets.
Adaptability:
- Linear Search: Adaptable to unsorted arrays but not optimized for large datasets.
- Binary Search: Specifically designed for sorted arrays, ensuring optimal performance.
Use Case:
- Linear Search: Suitable for small datasets or when the order of elements is random.
- Binary Search: Ideal for large sorted datasets, where efficient searching is crucial.
In this example, the choice between linear and binary search depends on the specific requirements of the task. A linear search might be sufficient if the array is small or unsorted. However, the binary search provides a significant performance advantage for larger sorted arrays. The comparison involves considering the trade-offs between time and space complexity, as well as the characteristics of the data structure.
Also read:Â Binary Search Trees And Its Uses
What is Running Time Analysis?
Running time analysis involves the time taken for processing relative to the size of the input. The nature of the input can vary depending on the problem at hand. Common types of inputs include – Size of an array, Number of elements in a matrix, Polynomial degree, Number of bits in binary representation and Edges and vertices in a graph.
The “Full Stack Data Science Pro” course by Physics Wallah is a comprehensive program designed to take learners from foundational concepts to advanced techniques in data science. This course is tailored to enhance the skills needed for in-depth analysis of algorithms within various data structures.
Key Highlights:
- Big Data Analytics: Understanding and working with big data analytics prepares learners to analyze algorithms in scenarios where massive datasets and complex data structures are involved.
- Real-world Projects: Engaging in real-world projects enables learners to apply algorithmic analysis to practical situations, enhancing problem-solving skills.
- Full-Stack Exposure: The course offers exposure to the full stack of data science, from data preprocessing to model deployment. This holistic approach enhances the ability to choose and implement algorithms effectively.
Types of Analysis of Algorithm in Data Structure
Analyzing algorithms in data structures involves evaluating their efficiency and performance. Different types of analyses help us understand how algorithms behave in various scenarios. Here are the key types of analysis of algorithms in the context of data structures:
Types of Analysis of Algorithm in Data Structure | |
Type of Analysis | Description |
Time Complexity Analysis | Time complexity measures the amount of time an algorithm takes to complete as a function of the input size. It helps in understanding how the algorithm’s performance scales with increasing input. |
Space Complexity Analysis | Space complexity evaluates the amount of memory space an algorithm requires to execute relative to the input size. It provides insights into the algorithm’s efficiency in utilizing memory resources. |
Big-O Notation | Big-O notation describes the upper bound of an algorithm’s time or space complexity in the worst-case scenario. It offers a simplified way to express the growth rate of an algorithm as input increases. |
Omega Notation | Omega notation represents the lower bound of an algorithm’s time complexity in the best-case scenario. It helps in understanding the minimum time required for the algorithm under certain conditions. |
Theta Notation | Theta notation combines both the upper and lower bounds, providing a tight bound on the algorithm’s complexity. It gives a more precise understanding of the algorithm’s growth rate in all scenarios. |
Amortized Analysis | Amortized analysis assesses the average time or space complexity per operation over a sequence of operations. It helps in understanding the overall performance of an algorithm in the long run. |
Average Case Analysis | Average case analysis evaluates the expected time or space complexity of an algorithm by considering the average input distribution. It provides a more realistic view of an algorithm’s performance. |
Also read:Â Analysis of Algorithms Explained
FAQs
What is the significance of analyzing algorithms in the context of data structures?
Understanding algorithms within the context of data structures is crucial for optimizing software performance. It enables efficient manipulation of diverse data types and aids in making informed decisions about algorithm selection based on specific data structure requirements.
What aspects does algorithm analysis in data structures cover?
Algorithm analysis encompasses time complexity, focusing on how algorithmic running time changes with input size, and space complexity, which delves into memory requirements and storage usage. The best, average, and worst-case analyses provide insights into algorithm behavior under varying scenarios.
How does amortized analysis contribute to algorithmic understanding?
Amortized analysis evaluates the average cost of sequences of operations, offering a dynamic perspective on algorithmic performance. It provides a more realistic portrayal, especially in scenarios where individual operations may exhibit different costs.
How does algorithm analysis guide the selection of algorithms for specific data structures?
By scrutinizing algorithm behavior concerning different data structures such as arrays, linked lists, trees, and graphs, analysts can choose algorithms that align seamlessly with the characteristics and requirements of each structure, optimizing overall system performance.
What role does real-world implementation play in algorithm analysis within data structures?
Real-world implementations validate theoretical analyses and provide practical insights into algorithmic behavior. Observing how algorithms perform in real-world scenarios contributes to their applicability, effectiveness, and scalability.
Why is understanding time complexity essential in algorithm analysis?
Time complexity analysis provides insights into how algorithmic running time scales with input size. It helps in predicting and optimizing the efficiency of algorithms, ensuring they meet performance expectations in various situations.
How does space complexity analysis contribute to efficient algorithm design?
Space complexity analysis evaluates the memory requirements and storage usage of algorithms. This understanding is crucial for optimizing resource utilization, ensuring scalability, and avoiding potential bottlenecks related to memory consumption.
Can algorithm analysis be applied to various types of data structures
Yes, algorithm analysis is versatile and applicable to various data structures, including arrays, linked lists, trees, graphs, and more. The analysis helps tailor algorithms to the specific characteristics and requirements of each data structure, ensuring optimal performance.