Algorithm analysis is an integral part of computational theory, which offers theoretical estimation for the needed resources of an algorithm to solve a particular computational problem. Please keep reading to know about it in detail!
Algorithm analysis is a fundamental tool that aids in predicting how algorithms will perform and selecting the most suitable algorithm for a given purpose. It provides valuable insights into an algorithm’s behavior under various conditions.Â
By examining an algorithm’s time and space complexity, organizations can estimate its runtime and memory usage across different input sizes. When a business encounters issues like prolonged execution times or excessive memory consumption with a particular algorithm, algorithm analysis can help identify the root causes of these problems.Â
Armed with this understanding, organizations can then embark on optimization efforts to enhance the algorithm’s performance. Additionally, algorithm analysis provides insights into an algorithm’s limitations, enabling organizations to anticipate its behavior under different scenarios. This knowledge is instrumental in determining when to employ a specific algorithm and when it’s prudent to explore alternative solutions.
Algorithm Analysis Overview
Algorithm analysis entails the analysis of algorithms to determine their computational complexity, which encompasses factors such as time, storage, and resource requirements for their execution. This typically involves deriving a function that correlates the size of an algorithm’s input with either the number of steps it necessitates (known as its time complexity) or the number of storage locations it employs (referred to as its space complexity).Â
An algorithm is considered efficient when this function yields small values or exhibits slow growth relative to increasing input size. It’s noteworthy that an algorithm’s behavior may differ with different inputs of the same size, making it essential to consider best-case, worst-case, and average-case scenarios.
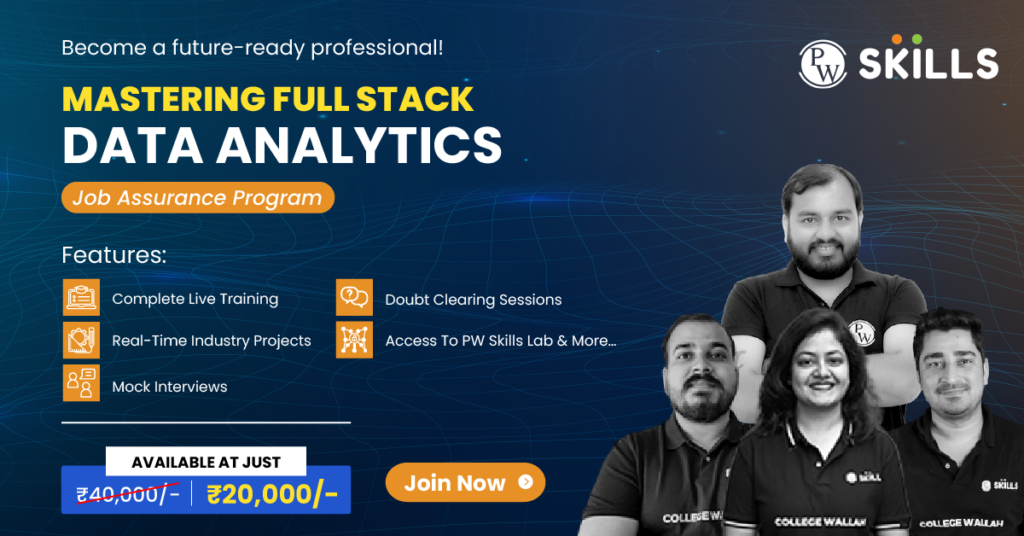
Without specific specifications, the function describing an algorithm’s performance typically represents an upper bound calculated from the most challenging inputs the algorithm might encounter.
The term “analysis of algorithms” was introduced by Donald Knuth. Algorithm analysis is a crucial component within the broader field of computational complexity theory, which furnishes theoretical estimations regarding the resources required by any algorithm designed to solve a particular computational problem. These estimates offer valuable insights into the pursuit of efficient algorithmic solutions.
What is an Algorithm?
An algorithm is a well-defined, step-by-step procedure or instructions designed to solve a particular problem or perform a specific task. It is a finite sequence of unambiguous actions or operations that, when executed, lead to the desired outcome.
As a computer scientist, it is imperative to have a comprehensive grasp of various algorithm types to utilize them effectively. When working on critical software projects, estimating the runtime performance becomes essential. This estimation becomes more reliable with a deep understanding of runtime analysis.
Moreover, in-depth knowledge of the involved algorithms is crucial for anticipating potential performance bottlenecks and ensuring the software delivers acceptable results.
However, there are instances where novel, uncharted problems emerge. When faced with such scenarios, you must devise innovative algorithms or apply existing ones creatively.
- Your proficiency in algorithms significantly enhances your ability to devise effective problem-solving strategies. Frequently, new challenges can be reduced to well-studied problems with relative ease, but this requires a foundational understanding of those established problems.
- Consider, for instance, the operation of an Internet switch. A switch connects to N cables, receiving data packets from these cables. The switch must analyze the packets and transmit them back via the appropriate cables. Like a computer, a switch operates in discrete time intervals, with packets sent at these intervals rather than continuously.Â
- In high-speed switches, the goal is to transmit as many packets as possible within each interval to prevent packet congestion and potential data loss. To achieve this, we aim to develop an algorithm that maximizes packet transmission during each break while maintaining the order of arrival.
Interestingly, it turns out that an algorithm used in a problem known as “stable matching” directly applies to our switch dilemma, despite initial appearances. This kind of insight can only be achieved through a solid foundation in algorithmic knowledge and understanding.
What is the Analysis of the Algorithm?
The analysis of an algorithm is the process of evaluating and understanding the performance characteristics and resource requirements of the algorithm. It involves assessing various aspects of the algorithm’s behavior, such as its:
- Time Complexity: This refers to the amount of time or number of basic operations (e.g., comparisons, assignments) an algorithm takes to complete as a function of its input size. Time complexity is usually expressed using Big O notation (e.g., O(n), O(n^2)), which provides an upper bound on the time required.
- Space Complexity: Space complexity pertains to the amount of memory or storage space an algorithm uses as a function of its input size. Like time complexity, space complexity is also expressed using Big O notation.
- Resource Utilization: This includes assessing the usage of other resources like network bandwidth or other hardware-specific considerations, depending on the context.
The analysis of an algorithm aims to answer questions like:
- How does the algorithm’s performance scale as the input size grows?
- What is the worst-case scenario for time or space usage?
- Is the algorithm efficient in terms of time and space use?
- How does the algorithm compare to alternative algorithms for the same task?
Types of Algorithm Analysis
By now, we are likely aware that an algorithm’s running time tends to increase as the size of the input grows. However, there are scenarios where the running time remains constant, regardless of the input’s size.
Interestingly, even when the input size is consistent, an algorithm’s running time can vary. This leads us to perform a comprehensive analysis of algorithms, which encompasses the best, average, and worst-case scenarios. These analyses help us understand how the algorithm may perform under various conditions, whether exceptionally efficiently or less so.
Types of Algorithm Analysis | ||
Analysis Type | Description | Example |
Time Complexity | Measures how the execution time grows with input size using Big O, Omega, and Theta notations. | Example: QuickSort – O(n^2) in worst case. |
Space Complexity | Assesses the memory usage concerning input size using space complexity notations. | Example: MergeSort – O(n) additional space. |
Worst-Case Analysis | Evaluates an algorithm’s performance under the condition that leads to the maximum execution time. | Example: Linear Search – O(n) in worst case. |
Average-Case Analysis | Considers the expected performance over all possible inputs based on a probability distribution. | Example: Hash table with successful lookups. |
Best-Case Analysis | Determines the minimum execution time an algorithm can achieve. | Example: QuickSort – O(n log n) with sorted input. |
Amortized Analysis | Assesses the average cost of a sequence of operations, even if individual operations vary in cost. | Example: Dynamic Array’s insert operation. |
Empirical Analysis | Measures an algorithm’s performance by running it on actual data or simulations. | Example: Benchmarking a sorting algorithm. |
Asymptotic Analysis | Focuses on the growth rate of resource consumption with a large input, typically using Big O notation. | Example: Bubble Sort – O(n^2) time complexity. |
Stability Analysis | Determines whether sorting algorithms maintain relative order of equal elements in the output. | Example: Stable sorting for student records. |
Parallelism Analysis | Evaluates an algorithm’s suitability for parallel and distributed computing with multiple processors. | Example: Parallel Matrix Multiplication. |
Algorithm Analysis Example
Let’s consider an example to illustrate algorithm analysis.
Binary Search (for Sorted Arrays)
Algorithm: Divide and conquer approach for sorted arrays.
Pseudocode:
left = 0
right = n – 1
while left <= right:
    mid = (left + right) // 2
    if arr[mid] is equal to target:
        return mid
    elif arr[mid] < target:
        left = mid + 1
    else:
        right = mid – 1
return -1
Time Complexity: O(log n) for sorted arrays, as it halves the search space with each comparison.
Hash Table Search (using Hashing)
- Algorithm: Store the elements in a hash table with their values as keys and indices as values. Then, look up the target element in the hash table.
Pseudocode
Create a hash table
for i from 0 to n-1:
    Add (arr[i], i) to the hash table
if hash_table contains target:
    return index of target in hash table
return -1
Time Complexity: O(1) on average for hash table operations, assuming a well-designed hash function.
In the above examples, Binary Search is efficient with a time complexity of O(log n), but it requires a sorted array. Hash Table Search offers O(1) average time complexity for lookups, provided the hash function is well-distributed. It is highly efficient but may consume more memory.
Also read: Data Science With Roles and Responsibilities
Importance of Algorithm Analysis
Algorithm analysis is pivotal in computer science and related fields due to its significant importance in various aspects. Here are some key reasons why algorithm analysis is crucial:
- Efficiency Optimization: Algorithm analysis helps in identifying and developing efficient algorithms. By evaluating the time and space complexity of algorithms, it is possible to optimize them for better performance. Efficient algorithms are critical when speed and resource usage matter, such as large-scale data processing, scientific simulations, and real-time systems.
- Algorithm Selection: For a specific problem, multiple algorithms may exist. Algorithm Analysis aids in selecting the most suitable one for the task. By comparing time and space complexities, you can choose the algorithm that performs optimally for the expected input sizes.
- Resource Management: Efficient algorithms are crucial in resource-constrained environments. For example, in embedded systems, mobile devices, or cloud computing, minimizing resource usage is essential. Algorithm Analysis helps in selecting algorithms that maximize resource utilization.
- Scalability: As data sizes grow, algorithm performance can change significantly. Understanding how algorithms scale is vital, especially in the age of big data. Algorithm Analysis helps predict how an algorithm will behave as input sizes increase.
- Comparative Analysis: Computer scientists often face multiple algorithm choices for solving the same problem. Algorithm analysis enables a comparative evaluation of these choices, helping to determine which algorithm is most suitable for a particular application.
- Predictive Insights: Through algorithm analysis, one can predict how an algorithm will behave under various conditions. This predictive capability is invaluable for system design, enabling engineers to make informed decisions about software and hardware requirements.
- Software Optimization: Efficient algorithms are at the heart of software optimization. Algorithm analysis provides insights into the bottlenecks and potential areas for improvement in software systems. This is particularly important in application domains where speed is critical, such as video games, financial trading, and scientific simulations.
- Algorithm Design and Innovation: Algorithm analysis fosters the design and innovation of new algorithms.Â
- Quality Assurance: Algorithm analysis helps prevent performance-related issues, ensuring a positive user experience and reducing the risk of system failures.
- Cost Savings: In the business world, efficient algorithms can save both time and money. Reduced execution time can lead to cost savings, while efficient memory usage can optimize hardware expenses.
Algorithm analysis is indispensable for addressing the ever-increasing computational demands of modern applications. It enables the design of more efficient algorithms and aids in resource optimization. It plays a vital role in selecting algorithms that meet the specific needs of diverse applications and industries.
Also read: 50 Most Asked Basic Coding Questions of All Time
Algorithm Analysis in Data Structure
Algorithm analysis in data structures refers to assessing and understanding the performance characteristics of algorithms used in data structure operations. It involves evaluating how efficient an algorithm is in terms of time and space usage when applied to various data structure tasks. The primary focus of algorithm analysis in data structures is to:
- Time Complexity Analysis: This aspect of algorithm analysis evaluates how execution time increases with the size of the input data. Algorithms can be analyzed for their best-case, average-case, and worst-case time complexities, typically denoted using big O notation (e.g., O(n), O(n^2)).
- Space Complexity Analysis: This involves determining the memory requirements of an algorithm about the input data size. Space complexity helps assess how an algorithm utilizes memory as the input grows.
- Performance Comparison: Algorithm analysis allows for comparing different algorithms for the same data structure operation. By understanding their performance characteristics, one can choose the most efficient algorithm for a specific task.
- Resource Utilization: It helps evaluate how efficiently an algorithm utilizes system resources such as CPU time, memory, and storage.
- Amortized Analysis: Amortized analysis assesses the average cost of a sequence of operations on a data structure. It ensures that while individual functions may have varying prices, the overall average is bounded.
- Asymptotic Analysis: Asymptotic analysis, particularly big O notation, describes the upper bound of an algorithm’s time or space complexity as the input size approaches infinity. It provides a high-level overview of algorithm efficiency.
- Experimental Analysis: Besides theoretical analysis, practical experiments, and empirical data are often used to assess algorithm performance on real-world data sets. This helps in fine-tuning algorithms for specific applications.
- Parallelism and Distributed Systems: With the advent of multi-core processors and distributed computing, algorithm analysis may also consider how algorithms behave in parallel or distributed environments, ensuring efficient resource utilization.
- Stability Analysis: Some data structure operations, such as sorting, may involve maintaining the relative order of equal elements. Stability analysis checks if an algorithm preserves the order of equal parts.
- Real-world Application: Algorithm analysis in data structures is crucial for developing efficient applications. It ensures that data-intensive software, such as databases, search engines, and simulations, performs optimally.
Algorithm analysis in data structures is a fundamental aspect of computer science and software engineering. It enables developers to choose, design, and implement data structure algorithms that meet the efficiency requirements of various applications, ensuring optimal performance and resource utilization.
Example of Algorithm analysis in data structure
Let’s consider a standard data structure operation: searching for an element in an array. We’ll analyze different algorithms for this operation in terms of time complexity.
Problem: Searching for a specific element in an array of integers.
Input: An array of integers arr of size n and a target element target.
Objective: Find the index of the target element in the array if it exists or return -1 if it does not.
Linear Search:
Algorithm: Iterate through the array one element at a time until the target element is found.
Pseudocode:
for i from 0 to n-1:
    if arr[i] is equal to target:
        return i
return -1Â
Time Complexity: In the worst case, when the element is not in the array or is at the last position, it takes O(n) comparisons.
In the above example, Linear Search has a time complexity of O(n), making it suitable for small arrays or when elements are not sorted.
Also check: Top 30 Most Asked Basic Programming Questions Asked During Interviews
Algorithm Analysis Python
Algorithm analysis in Python involves evaluating the efficiency of algorithms in terms of time and space complexity. Python, a high-level programming language, allows you to implement, analyze, and compare different algorithms easily. Let’s explore how you can perform algorithm analysis in Python:
Implement Algorithms
Start by implementing the algorithms you want to analyze. Write Python functions or classes that solve specific problems. For example, you might implement sorting algorithms like Bubble Sort, Selection Sort, and Quick Sort.
Measure Execution Time
Python provides the time module, which you can use to measure the execution time of your algorithms. You can record the start time, execute the algorithm, and then calculate the elapsed time to gauge its efficiency.
import time
def my_algorithm(data):
    start_time = time.time()
    # Execute your algorithm here
    elapsed_time = time.time() – start_time
    return elapsed_time
Use Profiling Tools
Python offers built-in tools like cProfile and third-party packages like line_profiler. These tools provide detailed statistics about the execution time of functions and lines of code, helping you identify bottlenecks.
Memory Profiling
You can analyze the memory usage of your algorithms using packages like memory profiler. Profiling memory usage is essential for understanding space complexity.
Plotting Libraries
Utilize Python plotting libraries like matplotlib to visualize the time and space complexities of different algorithms. Creating graphs and charts makes it easier to compare algorithms.
Benchmarking
To compare the performance of different algorithms, create benchmarks. Benchmarking involves running multiple algorithms on the same input data and recording their execution times. Python has libraries like timeit for benchmarking.
import timeit
def benchmark_algorithms():
    algorithm1_time = timeit.timeit(‘my_algorithm(data)’, globals=globals(), number=1000)
    algorithm2_time = timeit.timeit(‘another_algorithm(data)’, globals=globals(), number=1000)
    # Compare and analyze the results
Big O Notation
Use the Big O notation to provide a theoretical analysis of the algorithm’s efficiency. Compare the theoretical analysis with the empirical results obtained through execution time measurement.
OptimizationÂ
Python allows you to optimize your code by using efficient data structures and libraries. For example, using NumPy for numerical operations can significantly improve performance.
Here’s a basic example of how you can measure the execution time of a function using Python:
import time
def my_algorithm(n):
    result = 0
    for i in range(1, n + 1):
        result += i
    return result
n = 10000
start_time = time.time()
my_algorithm(n)
elapsed_time = time.time() – start_time
print(f”Execution time for n={n}: {elapsed_time} seconds”)
This script calculates the sum of the first 10,000 natural numbers and measures the execution time.
Algorithm Analysis FAQ's
How can I compare two algorithms for the same task?
To compare algorithms, evaluate their time and space complexities. Look for the algorithm with the lowest time complexity for large input sizes if execution time is the primary concern. Similarly, select the algorithm with lower space complexity if memory usage is the primary concern.
What is time complexity in algorithm analysis?
Time complexity measures the computational time an algorithm takes as a function of the input size. It helps us understand how an algorithm's runtime scales with increasing input.
How is time complexity represented in algorithm analysis?
Time complexity is often represented using Big O notation. It provides an upper bound on the growth rate of an algorithm's runtime as the input size increases.
What is space complexity in algorithm analysis?
Space complexity evaluates the memory usage of an algorithm as a function of the input size. It helps us understand how much memory an algorithm needs for different input sizes.
How is space complexity represented in algorithm analysis?
Space complexity is also represented using Big O notation. It describes the upper bound on an algorithm's memory usage as the input size increases.