Applet In Java Program may seem daunting at first, but with the right understanding and practice, you can easily incorporate these dynamic elements to enhance your programming skills.
Applet In Java Program: As software development becomes increasingly complex, the need for reusable and understandable program components grows ever more important. Java applets in particular have long been a powerful tool for delivering interactive multimedia experiences directly within web pages.
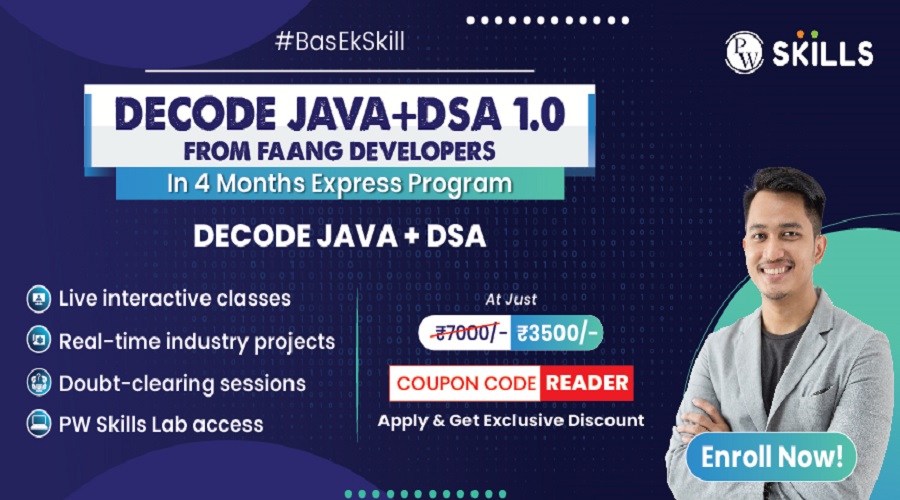
However, with evolving technologies and new design priorities, the role of applets finds itself at a crossroads. While many now assume applets to be obsolete, we believe a more nuanced perspective is needed.
If you’re looking for the perfect course to help you master Java programming and applet usage, then look no further! Decode Java+DSA 1.0 by Physics Wallah is the best Java course out there. And with our exclusive “READER” coupon code, you can get amazing discounts that will make learning even more rewarding. So don’t wait any longer and join us on this exciting journey into the world of applets in Java programming!
Applet In Java Program Definition
An applet in Java is a specialized program designed to run within a web browser, embedded within a webpage. Applets are Java programs specifically created to be integrated into web pages, allowing for dynamic content generation within the browser. Unlike standalone Java applications, applets operate on the client side, meaning they execute within the user’s web browser environment.
Despite running within a browser, an applet has access to the entire Java API, making it functionally equivalent to a regular Java application. Java applet serves as a tool to enhance web pages with interactive and dynamic functionalities, leveraging the capabilities of the Java programming language.
Java Applet Programs Examples With Output
Java applets were small applications written in the Java programming language that could be downloaded from web servers and run in a web browser. However, modern browsers have deprecated support for Java applets due to security concerns. Still, I can provide you with a simple example of a Java applet and its output for educational purposes.
Example: Simple Java Applet to Display a Message
Here’s a basic Java applet code that displays a message “Hello, World!” on the browser window:
import java.applet.Applet;
import java.awt.Graphics;
public class HelloWorldApplet extends Applet {
    public void paint(Graphics g) {
        g.drawString(“Hello, World!”, 50, 50); // Draws the string at x=50, y=50
    }
}
Steps to Run the Java Applet:
Compile the Applet: Save the above code in a file named HelloWorldApplet.java. Open a terminal, navigate to the directory where you saved the file, and compile it using the javac command:
javac HelloWorldApplet.java
Create HTML File: Create an HTML file (HelloWorldApplet.html) to embed and display the applet in a web browser:
<!DOCTYPE html>
<html>
<head>
    <title>HelloWorld Applet</title>
</head>
<body>
    <applet code=”HelloWorldApplet.class” width=”300″ height=”200″></applet>
</body>
</html>
Run the Applet: Open the HelloWorldApplet.html file with a web browser that supports Java applets (you might need to enable Java in your browser settings). You should see a window displaying the message “Hello, World!”.
Note:
- Ensure you have the Java Development Kit (JDK) installed on your machine to compile Java code.
- Modern browsers like Chrome, Firefox, and Edge no longer support Java applets. You may need to use an older browser or make specific configurations to run Java applets.
Also Read: Anagram In Java: Examples, Programs, Solutions
Applet Life Cycle in Java
The life cycle of a Java applet refers to the various stages an applet goes through from its initialization to its termination. Understanding the applet life cycle is essential for developing applets correctly. Here are the stages involved in the life cycle of a Java applet:
1) Initialization:
- Class Loading: The applet’s class is loaded by the Java Virtual Machine (JVM).
- Initialization: The init() method is invoked to initialize the applet. This method is called once in the lifecycle of an applet.
- Parameters: The getParameter() method retrieves any parameters specified in the HTML tag that embeds the applet.
2) Starting:
- Start Method: The start() method is called after the init() method to indicate that the applet is starting execution.
- Resuming: If an applet is stopped (using the stop() method), it can be restarted using the start() method.
3) Running:
- Running State: The applet remains in this state as long as it is visible on the web page.
- User Interaction: During this phase, users can interact with the applet, and the applet can process events and update its display as required.
4) Stopping:
- Stop Method: The stop() method is called when the applet is no longer visible on the screen or when the web page containing the applet is closed.
- Cleanup: In this method, you can release resources, stop threads, or perform any cleanup activities necessary when the applet stops.
5) Destroying:
- Destroy Method: The destroy() method is invoked when the applet is about to be unloaded from memory.
- Final Cleanup: You can perform any final cleanup activities, such as releasing resources, closing files, etc., in this method.
Methods Involved:
- init(): Initializes the applet and is called when the applet is loaded.
- start(): Starts the execution of the applet after it has been initialized.
- stop(): Stops the applet’s execution. It is called when the applet is no longer visible or active.
- destroy(): Performs any cleanup operations and is called before the applet is unloaded.
Example:
Here’s a simple example illustrating the life cycle methods of a Java applet:
In this example, you can observe the sequence of method calls when the applet goes through its life cycle stages.
import java.applet.Applet;
import java.awt.Graphics;
public class AppletLifeCycleDemo extends Applet {
    public void init() {
        System.out.println(“Initializing Applet…”);
    }
    public void start() {
        System.out.println(“Starting Applet…”);
    }
    public void stop() {
        System.out.println(“Stopping Applet…”);
    }
    public void destroy() {
        System.out.println(“Destroying Applet…”);
    } Â
    public void paint(Graphics g) {
        g.drawString(“Applet Life Cycle Demo”, 50, 50);
    }
}
Types of Applet in JavaÂ
Java applets are specialized programs designed to run within a web browser environment. They offer interactive and dynamic content to web pages, making user interactions more engaging. Applets are categorized into different types based on their execution context and origin. Here are the two primary types of applets:
1) Local Applet:
Definition:
A local applet is an applet that is stored and executed from the user’s local machine or computer system. When a user accesses a web page containing a local applet, the applet is downloaded to the user’s computer and then executed within the browser.
Characteristics:
- Execution: Runs on the client-side, meaning the user’s local machine processes the applet.
- Security: Typically, local applets have fewer security restrictions compared to remote applets since they are considered more trustworthy by the browser.
- Performance: Generally offers faster response times as it operates directly on the user’s system resources.
- Example: An applet designed to interact with local hardware devices, such as printers or scanners, would typically be a local applet.
2) Remote Applet:
Definition:
A remote applet is an applet that is stored on a remote server and is executed on the client-side after being downloaded from that server. These applets are accessed through URLs and are not stored locally on the user’s machine.
Characteristics:
- Execution: Executes on the client-side after being downloaded from a remote server.
- Security: Due to security concerns, modern browsers often restrict the functionalities of remote applets to prevent potential security vulnerabilities, such as unauthorized access or malicious activities.
- Portability: Since remote applets are not stored locally, they can be accessed from any machine with an internet connection, making them highly portable.
- Example: A dynamic graphical interface or a data visualization tool embedded within a web page would likely be a remote applet, as it requires server-side resources and data processing capabilities.
Understanding the distinction between local and remote applets is essential for developers and users alike. While both types offer unique advantages and use cases, they also come with their respective considerations related to security, performance, and accessibility. As web technologies continue to evolve, the role and relevance of applets in modern web development contexts may shift, but their fundamental characteristics and classifications remain integral to understanding their functionality and implementation.
Simple Applet Program in JavaÂ
Creating a simple applet in Java involves defining a class that extends the Applet class provided by the java.applet package. Below is a basic example of how you can create a simple applet program in Java:
import java.applet.Applet;
import java.awt.Graphics;
// Defining a simple applet by extending the Applet class
public class SimpleAppletExample extends Applet {
    // Overriding the paint method to draw something on the applet window
    @Override
    public void paint(Graphics g) {
        // Using the Graphics object to draw a string on the applet window
        g.drawString(“Welcome to Simple Applet Programming!”, 50, 50);
    }
    // This method is invoked when the applet is started
    @Override
    public void start() {
        // Initialization code, if any, can be placed here
    }
    // This method is invoked when the applet is stopped
    @Override
    public void stop() {
        // Cleanup code, if any, can be placed here
    }
}
Steps to Compile and Run the Applet:
Create a Java File: Copy the above Java code and save it into a file named SimpleAppletExample.java.
Compile the Java File: Open a terminal or command prompt, navigate to the directory where you saved SimpleAppletExample.java, and then compile it using the following command:
javac SimpleAppletExample.java
Run the Applet: After successfully compiling, run the applet using the appletviewer tool (available in JDK) as follows:
appletviewer SimpleAppletExample.java
When you run the applet using the appletviewer tool, a window should appear displaying the text “Welcome to Simple Applet Programming!” as specified in the paint() method of the applet class.
Also Read: API Full Form In Java
Applet Architecture in Java
Applet architecture in Java is structured to provide a foundation for creating interactive web-based applications that can be embedded within web browsers. The architecture defines a lifecycle for applets and provides a set of methods and classes to facilitate the development of such applications. Below are the key components and aspects of the applet architecture in Java:
Key Components of Applet Architecture:
1) Applet Class (java.applet.Applet):
- All applets in Java are derived from the Applet class.
- It provides a base class for creating applets and defines several methods that you can override to control the applet’s behavior during its lifecycle.
2) Lifecycle Methods:
- init(): This method is called when an applet is initialized. It’s used for applet initialization tasks like setting up resources.
- start(): Invoked after the init() method or when the applet is revisited. Use it to start or resume operations.
- stop(): Called when the applet is no longer visible or needs to stop its operations.
- destroy(): Invoked when the applet is about to be unloaded or destroyed. Cleanup tasks are typically performed here.
3) Graphics Class (java.awt.Graphics):
- Used for rendering and drawing shapes, text, and images on the applet window.
- The paint(Graphics g) method of the applet class receives a Graphics object as an argument that you can use for drawing operations.
4) Event Handling:
- Applets can handle user interactions like mouse clicks, keypresses, etc., by implementing event-handling methods such as mouseClicked(), keyPressed(), etc.
5) Security Restrictions:
- Applets are subject to security restrictions imposed by the browser or Java runtime environment (JRE). They have limited access to resources and can’t perform certain operations for security reasons.
Applet Workflow:
1) Initialization:
- When an applet is loaded in a browser, the init() method is called to perform initialization tasks like setting up GUI components or loading resources.
2) Starting and Stopping:
- After initialization, the applet enters the started state, and the start() method is invoked. Here, you can start animations, threads, or any ongoing tasks.
- When the user navigates away from the applet’s page or minimizes the browser window, the stop() method is called to pause ongoing activities.
3) Rendering and Interaction:
- The paint(Graphics g) method is invoked whenever the applet needs to redraw its content, such as when it’s first loaded or when it’s uncovered after being minimized.
- User interactions trigger event-handling methods that you can override to respond to specific user actions.
4) Cleanup:
- When the applet is no longer needed or the page is unloaded, the destroy() method is called to release resources and perform cleanup operations.
Also Read: What is Java API? Definition, Types, & Uses
Methods of Applet in Java Program
In Java, the Applet class provides a range of methods that you can override to define the behavior and lifecycle of your applet. Here’s an overview of some of the key methods available in the Applet class:
1) Initialization Methods:
init() Method:
- This method is called when the applet is first loaded into the browser or applet viewer.
- It’s typically used for one-time initialization tasks like setting up GUI components, initializing variables, or loading resources.
- You can override this method to perform custom initialization for your applet.
2) Starting and Stopping Methods:
start() Method:
- Invoked after the init() method and whenever the applet is revisited after being stopped.
- Use this method to start or resume activities like animations, threads, or ongoing tasks.
stop() Method:
- Called when the applet is no longer visible, such as when the user navigates away from the applet’s page or minimizes the browser window.
- Use this method to pause ongoing activities, release resources, or perform cleanup tasks.
3) Rendering and Repainting:
paint(Graphics g) Method:
- This method is invoked whenever the applet needs to redraw its content, such as when it’s first loaded, resized, or uncovered after being minimized.
- Override this method to define custom drawing operations using the Graphics object passed as an argument.
4) Cleanup and Termination:
destroy() Method:
- Invoked when the applet is about to be unloaded or destroyed, such as when the page containing the applet is closed or refreshed.
- Use this method to release resources, stop threads, or perform any final cleanup operations.
5) Miscellaneous Methods:
getParameter(String name) Method:
- Returns the value of the specified parameter passed to the applet.
- Useful for retrieving parameters defined in the HTML tag that embeds the applet.
By overriding these methods in your applet class, you can define its behavior during various stages of its lifecycle, from initialization and rendering to cleanup and termination.
Learning Java can be a daunting task, especially for those new to coding. That’s why we highly recommend checking out Decode Java+DSA 1.0 by Physics Wallah. This comprehensive course not only teaches you the basics of Java but also dives into data structures and algorithms – essential concepts for any programmer. And as my loyal readers, we would like to offer you a special discount using the code “READER” at checkout.
For Latest Tech Related Information, Join Our Official Free Telegram Group :Â PW Skills Telegram Group
Applet In Java Program FAQs
What is a Java applet with an example?
A Java applet is a small application that runs within a web browser. Unlike standalone Java applications, applets are embedded in webpages and require the Java plugin to run. An example could be a simple applet that displays "Hello, World!" within a web browser when loaded.
What is the Java applet PDF?
A Java applet PDF typically refers to a Java applet embedded within a PDF document. This allows for interactive content in PDFs, such as forms or animations, powered by Java applets.
How can I run a Java applet program?
To run a Java applet, you typically embed the applet code within an HTML file and use a web browser that supports Java plugins. You can also use the appletviewer tool provided in the JDK to run applets directly without a browser.
What are the 5 methods of applet in Java?
The five methods of the Applet class in Java are:
init(): Initializes the applet and performs one-time setup tasks.
start(): Resumes or starts the applet's execution.
stop(): Pauses the applet's execution.
destroy(): Cleans up resources before the applet is terminated.
paint(Graphics g): Handles the rendering or redrawing of the applet's graphical content.