Data Structure for Java is a stepping stone toward mastering Java programming. At this point, staying informed about the latest trends and tools in data structure implementation would be an example of wise planning.Â
Data Structure for Java: As Java continues to develop and stay current with the latest technology advances, knowledge of certain data structures is becoming increasingly important for competent programming. Even experienced Java programmers may benefit from brushing up on their understanding of how these data structures work and when they should be applied.Â
By learning more about mainstream data structures now, you can stay ahead of the curve in 2024 and beyond! In this blog post, we’ll explore 7 different data structures that all Java programmers should know by heart—from trees to graphs and even priority queues. So if you’re ready to widen your skillset as a Java programmer, let’s dive right into it!
Ipf you are serious about developing your expertise within Java programming and DSAs, then taking a course such as Decode Java+DSA 1.0 from Physics Wallah could prove invaluable to you. Good luck with your progress!
Also read:Analysis of Algorithm in Data StructureÂ
Data Structures in Java with Examples
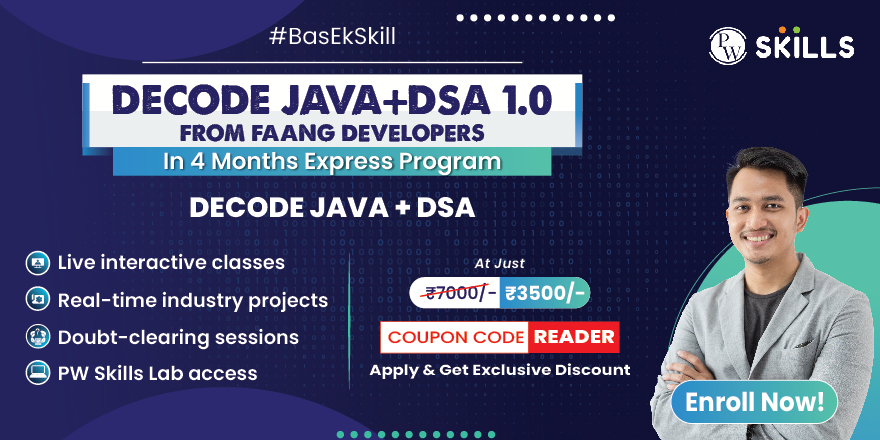
Data structures are fundamental components of computer science that allow us to organize and store data efficiently. In Java, various data structures are available, each serving specific purposes. Let’s explore some common data structures along with examples:
1) Arrays:
Arrays stand as one of the most straightforward and widely utilized data structures in Java. They represent a collection of elements of the same data type stored in sequential memory locations. Arrays maintain a fixed size, rendering them suitable for scenarios where the number of elements remains constant throughout the program’s execution. The process of accessing elements in an array is accomplished in constant time (O(1)), showcasing its efficiency for random access.
Example:
int[] numbers = {1, 2, 3, 4, 5};
2) Linked Lists:
A linked list is a dynamic data structure where elements are connected through nodes, and each node points to the next one.
Example:
class Node {
    int data;
    Node next;
    public Node(int data) {
        this.data = data;
        this.next = null;
    }
}
3) Stacks:
A data structure aligning with the Last-In-First-Out (LIFO) concept is known as a stack. The implementation of stacks in Java can be achieved using the Stack class or Deque interface. Stacks find common application in managing function calls, facilitating undo operations, and parsing expressions.
Example:
Stack<Integer> stack = new Stack<>();
stack.push(1);
stack.push(2);
int topElement = stack.pop();Â // Removes 2
4) Queues:
Unlike stacks, queues adhere to the First-In-First-Out (FIFO) principle. Java provides various queue implementations, such as LinkedList and PriorityQueue. Queues play a crucial role in tasks such as organizing jobs in a queue, scheduling processes, and implementing algorithms for breadth-first search.
Example:
Queue<String> queue = new LinkedList<>();
queue.add(“First”);
queue.add(“Second”);
String frontElement = queue.remove();Â // Removes “First”
5) Trees:
Trees are hierarchical data structures with a root node and branches leading to child nodes.
Example:
class TreeNode {
    int data;
    TreeNode left, right;
    public TreeNode(int data) {
           }
}
6) Graphs:
Graphs consist of nodes (vertices) and edges, representing connections between nodes.
Example:
class Graph {
    int vertices;
    LinkedList<Integer>[] adjacencyList;
    public Graph(int vertices) {
        this.vertices = vertices;
        this.adjacencyList = new LinkedList[vertices];
        for (int i = 0; i < vertices; i++) {
            this.adjacencyList[i] = new LinkedList<>();
        }
    }
}
7) Maps:
Maps serve as key-value data structures enabling the storage and retrieval of values associated with unique keys. In Java, HashMap and TreeMap are extensively used map implementations. HashMap ensures swift access to values based on their keys, while TreeMap organizes keys in a sorted order.
Example:
public class HashMapExample {
    public static void main(String[] args) {
        // Creating a HashMap
        Map<String, Integer> studentScores = new HashMap<>();
        // Adding key-value pairs
        studentScores.put(“Alice”, 95);
        studentScores.put(“Bob”, 80);
        studentScores.put(“Charlie”, 92);
        studentScores.put(“Diana”, 88);
        // Accessing values using keys
        System.out.println(“Bob’s Score: ” + studentScores.get(“Bob”));
        // Checking if a key is present
        String studentName = “Alice”;
        if (studentScores.containsKey(studentName)) {
            System.out.println(studentName + “‘s Score: ” + studentScores.get(studentName));
        } else {
            System.out.println(studentName + ” not found in the records.”);
        }
        // Iterating through the HashMap
        System.out.println(“Student Scores:”);
        for (Map.Entry<String, Integer> entry : studentScores.entrySet()) {
            System.out.println(entry.getKey() + “: ” + entry.getValue());
        }
                String studentToRemove = “Charlie”;
        studentScores.remove(studentToRemove);
        System.out.println(studentToRemove + “‘a score removed.”);
        // Updated HashMap after removal
        System.out.println(“Updated Student Scores:”);
        for (Map.Entry<String, Integer> entry : studentScores.entrySet()) {
            System.out.println(entry.getKey() + “: ” + entry.getValue());
        }
    }
}
Data Structures And Algorithms in Java
Below tables shows the data structures and algorithms in Java:
Data Structures and Algorithms in Java | |
Data Structures | Description |
Arrays | Ordered collection of elements of the same data type. |
Linked Lists | Dynamic data structure where elements are connected through nodes. |
Stacks | Follows Last In, First Out (LIFO) principle. |
Queues | Follows First In, First Out (FIFO) principle. |
Trees | Hierarchical data structures with a root node and branches. |
Graphs | Consists of nodes (vertices) and edges representing connections. |
Also read:Â Unlock The Power Of Java And Data Structures With Decode Java With DSA 1.0!
Data Structures and Algorithms in Java | ||
Algorithms | Description | Example |
Sorting Algorithms | Techniques to arrange elements in a specific order. | Quick Sort, Merge Sort |
Searching Algorithms | Techniques to find an element’s presence and locate its position. | Binary Search, Linear Search |
Graph Algorithms | Algorithms used to traverse or search through graphs. | Breadth-First Search, Depth-First Search |
Dynamic Programming | Optimizing problems with overlapping subproblems. | Fibonacci Series |
Divide and Conquer | Breaks a problem into smaller subproblems and solves them. | Binary Search |
Greedy Algorithms | Make locally optimal choices at each stage. | Dijkstra’s Algorithm |
Also read:Â Top 15 Java Projects With Source Code [2024]
If you’re looking for assistance in learning Java Data Structures and Algorithms, Decode Java+DSA 1.0 by Physics Wallah is an excellent course option! All the best of luck in your studies – may you achieve great heights with the help of these data structures!
FAQs
How does Stack work in Java?
A stack in Java follows the Last In, First Out (LIFO) principle. Elements are added (pushed) and removed (popped) from the top. It is commonly used in parsing expressions, backtracking, and managing function calls.
Explain the concept of Binary Trees in Java?
A binary tree is a hierarchical data structure where each node has, at most, two children – left and right. It is used for efficient searching, sorting, and representing hierarchical relationships.
What is the purpose of a Graph in Java?
A graph is a collection of nodes (vertices) and edges connecting them. It models various relationships, such as networks, social connections, and dependencies. Common algorithms include Breadth-First Search and Depth-First Search.
How do Java Arrays differ from ArrayLists?
Arrays are fixed-size and can store primitive data types, while ArrayLists are dynamic and part of the Java Collections Framework. ArrayLists provide methods for dynamic resizing, making them more flexible.
What is the significance of Sorting Algorithms in Java?
Sorting algorithms arrange elements in a specific order, making searching and retrieval more efficient. Common sorting algorithms include Quick Sort, Merge Sort, and Bubble Sort.
Why is Dynamic Programming used in Java?
Dynamic Programming optimizes solving problems with overlapping subproblems. It stores the results of subproblems, preventing redundant calculations. It is commonly used for optimization problems, like finding the Fibonacci series.