JAVA OOP Tutorial: Java programming supports Object Oriented Programming (OOPs). OOPs contain real-world objects and classes. Java OOP tutorial provides a strong, powerful framework for designing and developing software with the help of object-oriented programming.
Java OOPs provide encapsulation, abstraction, and inheritance, which makes programming effective and easy. Let us learn about the Java OOP tutorial. Read the complete article to learn the Java OOP tutorial and understand OOPs easily.
Start your Development career with the Java with DSA and System Design 2.0 course at an affordable course with placement assistance.
Java OOP Tutorial: What are OOPs?
OOP stands for Object Oriented Programming. OOPs in Java provide faster execution times and a clear program structure. The Java OOP tutorial helps to implement code reusability, which means that we do not have to write the same code repeatedly. Many important programming languages provide Java OOP tutorials, such as C++, C#, Java, Ruby, etc.
What is Object Oriented Programming in Java?
Object Oriented Programming in Java is the use of real-world objects containing different methods and functions inside classes. It is used to implement inheritance, encapsulation, polymorphism, and abstraction with the help of Java. It simplifies the programming by providing easier modification and reusability.
Object Oriented Programming in Java also deals with relationships between various objects, known as Associations. It also contains concepts like Cohesion, Coupling, Aggregation, and composition.
Java OOP Tutorial: Object Oriented Programming Syllabus
Candidates can check out the complete Java OOP syllabus in the table below.
Java OOP Tutorial: Content of the Course |
|
Java OOP Tutorial: Course Outcome
The Java OOP Tutorial course provides the following outcome.
Java OOP Tutorial: Course Outcome |
|
JAVA OOP Tutorial: What Are Objects in OOPs?
Objects in OOps are real-world entities having states and behaviours. For example: a bike, chair, table, animal, pen, etc. An object is also referred to as an Instance of class. One or more objects can interact with each other and form a relationship. Objects are made from the blueprint of a class.
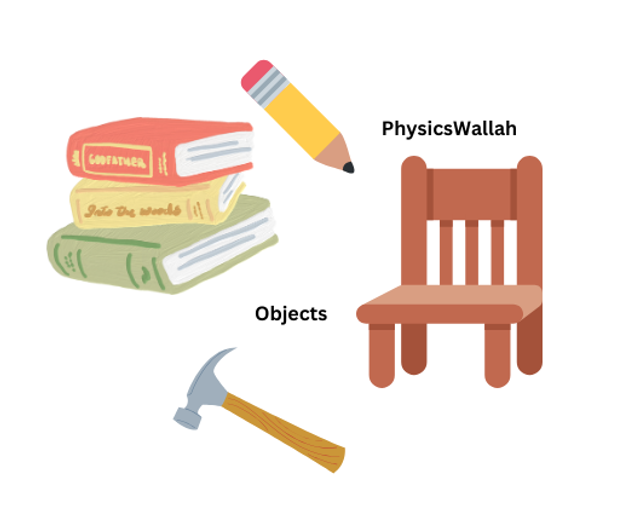
For example, let us suppose we are having a Class ‘Car’. The object in this class would be a specific car such as Hyundai, Tata, Mahindra, or Toyota. These are objects that have states and behaviours derived from the class.
Java OOP Tutorial: What Are Classes in OOPs?
Classes are referred to as blueprints, which describe the characteristics and behaviour of objects. It is used to create an object based on specific criteria. It is a collection of many real-world logical entities. A class contains different methods and attributes that operate on data.
Principles of Object Oriented Programming in Java
There are four major Principles of Object oriented programming in Java.
- Encapsulation: It is used to wrap essential data together in a single unit.
- Abstraction in OOPs: It hides the users’ internal implementation details and shows only the interactive details.
- Polymorphism: When a single method can be performed in more than one way, it is known as polymorphism.
- Inheritance: When an object from the derived class uses the properties and behaviour of its parent classes, it is known as Inheritance.
Learn more about the Principles of Object Oriented programming.
Association in OOPs
Association in oops is used to represent the relationship between various objects. There are many ways in which one object can be associated with one or many other objects. Let us know about four major types of associations in OOPs.
- One to One
- One to Many
- Many to One
- Many to Many
Learn more about Types of Association in object oriented programming in Java.
Aggregation in OOPs
Aggregation is a relationship that shows ‘has-a’ connection between one or many entities. One class contains the reference to another. In associations, deleting one class does not affect the other class object. For example, a driver class is associated with the Car class, but removing driver class will not affect the car class.
Java OOP Tutorial: Aggregation in OOPs |
// Association example
class Car { private String model; public Car(String model) { this.model = model; } public String getModel() { return model; } } class Driver { private String name; public Driver(String name) { this.name = name; } public void drive(Car car) { System.out.println(name + ” is driving a ” + car.getModel()); } } public class AssociationExample { public static void main(String[] args) { Car myCar = new Car(“Toyota”); Driver me = new Driver(“John”); me.drive(myCar); } } |
Composition in OOPs
Composition in Java oops is a type of relationship which represents strong relationship between objects. It refers to the “Belong to association”. The objects in composition cannot exist independently. For example, a car has objects like engine, start, break, but if we delete the Car class then these objects will no longer be accessible as they are strongly associated with the class Car.
Java OOP Tutorial: Composition in OOPs |
// Composition example
class Engine { public void start() { System.out.println(“Engine started”); } } class Car { private Engine engine; public Car() { this.engine = new Engine(); } public void start() { System.out.println(“Car started”); engine.start(); } } public class CompositionExample { public static void main(String[] args) { Car myCar = new Car(); myCar.start(); } } |
Recommended Reads
Data Science Interview Questions and Answers
Data Science Internship Programs
IIT Madras Data Science Course
Java OOP Tutorial FAQs
Q1. What is object oriented programming in Java?
Ans: OOP stands for Object Oriented Programming. OOPs in Java provide faster execution times and a clear program structure. Java OOPs provide encapsulation, abstraction, and inheritance, which makes programming effective and easy.
Q2. What is Abstraction in OOPs?
Ans: Abstraction in OOPs hides the users' internal implementation details and shows only the interactive details.
Q3. What are the four major principles of Object oriented programming?
Ans: The four major principles of Object oriented programming are.
Encapsulation
Abstraction
Polymorphism
Inheritance
Also, check the article for more details on the principles of polymorphism in OOPs.
Q4. What are inheritances in OOPs?
Ans: When an object from the derived class uses the properties and behaviour of its parent classes, it is known as Inheritance.