The OOP Principles is the basic foundation on which Object Oriented Programming works. OOPs use four major principles, such as abstraction, encapsulation, polymorphism and inheritance, as their foundation. The complete OOP principles revolve around the object and its behaviours. These principles in Oops make it more reliable and effective. In this article, we will learn about each one of them in detail.
What are the OOP Principles?
Object Oriented Programming is a programming concept which uses objects and classes to interpret different problem statements. There are four major OOP principles in programming: encapsulation, abstraction, polymorphism, and inheritance.
Encapsulation helps to hide the data from direct unauthorised access. Polymorphism allows objects to take different forms, and abstraction simplifies complex systems by hiding the implementation details. Inheritance helps to take different forms, just like abstraction.
4 Principles of Object Oriented Programming
There are four main principles of object-oriented programming. These are Encapsulation, abstraction, inheritance, and polymorphism. Let us learn them in detail.
1. Encapsulation in Object Oriented Programming
Encapsulation is one of the most essential principles of object-oriented programming. It helps to use an entity called a class, which contains attributes and methods.
It is used to hide the complete mechanism of how the implementation is taking place. It helps to secure our data by protecting it from direct access by unauthorised entities. It uses access specifiers to protect the internal state of our objects from outside entities.
There are three major types of access specifiers which help to control the access of data. These are public, private and protected specifiers. Learn more about Encapsulation in Object oriented programming.
Let us take an example to understand encapsulation in object oriented programming.
Encapsulation in object oriented programming |
public class BankAccount
{ private double balance; public double Balance { get { return balance; } private set { if (value >= 0) balance = value; } } public void Deposit(double amount) { if (amount > 0) Balance += amount; } public void Withdraw(double amount) { if (amount > 0 && amount <= Balance) Balance -= amount; } } |
The example above uses encapsulation to hide the important information double balance ().
2. Abstraction in Object Oriented Programming
Abstraction is used to simplify the complex working of a class or method by hiding unnecessary details and only highlighting the important details at the forefront. It helps users to interact with the system properly without worrying about how everything is working here.
Let us take a real-life example to understand abstraction. Let us suppose we want to buy a car, then we only need to have driving skills. We need not worry about the working of the car, how the wheels are moving, how the headlights work, and how the car starts. This explains abstraction in Object-oriented programming in the simplest way. However, check out Abstraction in Object oriented programming to know more about Abstraction in detail.
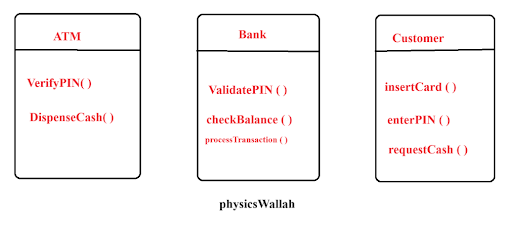
Also, check the bank and the customer example by clicking here to learn more about Abstraction in object-oriented programming.
3. Polymorphism in Object-oriented programming
Polymorphism in oops is one of the most important concepts in Object-oriented programming. It is a method in OOP principles that tells that a class or entity occurs in different forms. ‘Poly’ means many, and ‘morph’ means forms. Hence, polymorphism means to exist in many forms.
There are two main types of Polymorphism, compile-time polymorphism and runtime polymorphism.
- Compile Time Polymorphism: The compile time polymorphism in Oops is achieved by using method overloading. When more than one function has the same name but different parameters, it is called method overloading.
OOP principles: Compile time polymorphism |
public int multiply(int a, int b) {
int prod = a * b; return prod; } public int multiply(int a, int b, int c) { int prod = a * b * c; return prod; } } // Class 2 // Main class class Operation { // Main driver method public static void main(String[] args) { Product ob = new Product(); int prod1 = ob.multiply(1, 2); System.out.println( “Product of the two integer value :” + prod1); // Calling method to multiply 3 numbers int prod2 = ob.multiply(1, 2, 3); // Printing product of 3 numbers System.out.println( “Product of the three integer value:” + prod2); } } |
Here, we are using two same function names ‘multiply’ but with different parameters. It can be used in different conditions.
- Runtime polymorphism: Runtime polymorphism uses method overriding to achieve polymorphism. The method call here is resolved at the runtime. The method overriding method used in the subclass is already defined in its superclass which is executed at the runtime. Let us consider an example, where a class Animal has a method sound () which can be called either for dog or a cat depending upon the call.
OOP principles: Runtime polymorphism |
class Animal {
void sound() { System.out.println(“Animal makes a sound”); } } class Dog extends Animal { void sound() { System.out.println(“Dog barks”); } } class Cat extends Animal { void sound() { System.out.println(“Cat meows”); } } public class Main { public static void main(String[] args) { Animal myAnimal = new Dog(); myAnimal.sound(); // Output: Dog barks myAnimal = new Cat(); myAnimal.sound(); // Output: Cat meows } } |
4. Inheritance in Object Oriented Programming
Inheritance is one of the most important concepts in object oriented programming. It helps us to use the features and properties of an existing class in another class. The child class can use the features of the parent class. It promotes code re-usability.
Inheritance conveys “is-a” relationships, which means that the derived class is a special version of the base class. There are two important terms in inheritance.
- Base Class: The base class is the main part of inheritance from where the derived class uses the properties and features. It is also known as the parent class.
- Derived Class: The derived class uses the features of the base or parent class. It is also known as the child class.
OOP Principles: Inheritance |
// Base class (or superclass) Animal
class Animal { void eat() { System.out.println(“Animal is eating”); } void sleep() { System.out.println(“Animal is sleeping”); } } // Derived class (or subclass) Dog inheriting from Animal class Dog extends Animal { void bark() { System.out.println(“Dog is barking”); } } // Derived class (or subclass) Bird inheriting from Animal class Bird extends Animal { void chirp() { System.out.println(“Bird is chirping”); } } public class Main { public static void main(String[] args) { Dog myDog = new Dog(); Bird myBird = new Bird(); myDog.eat(); myDog.sleep(); myBird.eat(); myBird.sleep(); // Output: Animal is sleeping // Methods specific to subclasses myDog.bark(); // Output: Dog is barking myBird.chirp(); // Output: Bird is chirping } } |
OOP Principles FAQs
What are OOP principles?
The OOP Principles is the basic fundamental on which Object Oriented Programming works. OOPs use four major principles, such as abstraction, encapsulation, polymorphism and inheritance, as their foundation.
What are the four bases of object oriented programming?
The four main principles of object-oriented programming are encapsulation, abstraction, inheritance, and polymorphism. Learn more about them in the article above.
What is Encapsulation in Object-oriented programming?
Encapsulation is one of the most essential principles of object-oriented programming. It helps to use an entity called a class, which contains attributes and methods.
What is an abstraction in object-oriented programming?
Abstraction is used to simplify the complex working of a class or method by hiding unnecessary details and only highlighting the important details at the forefront.