Competitive coding questions are necessary for technical interviews for big tech companies like Amazon, Flipkart, and Microsoft. Most of the service-based and product-based companies include coding-related questions in their interview questions. Given here in this article are some of the top interview questions asked in the technical interview.
What is Competitive Programming?
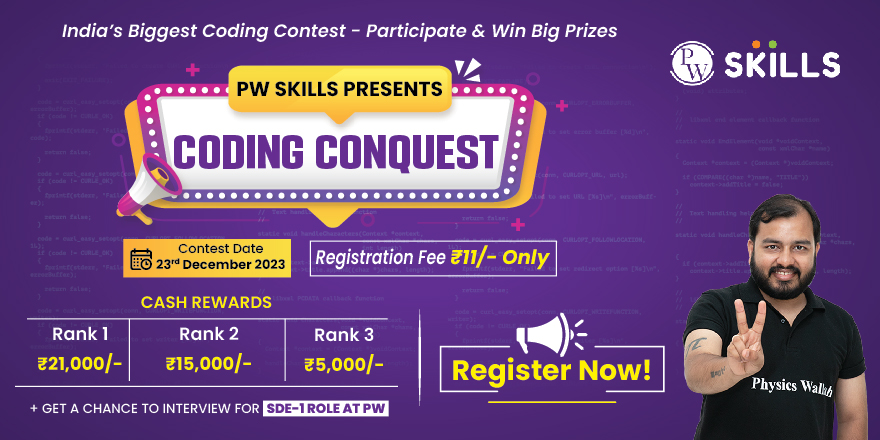
Competitive programming is a part of coding where we solve challenging questions and compete with other programmers based on some real life, well-oriented coding problems based on various topics. Many websites offer coding practice spaces and coding competitions.
We are organizing an all-India coding competition for everyone who is interested in coding and wants to earn a lot of prizes and benefits. Check out the PW Coding Conquest on December 21, 2024, and register soon.
Why is Competitive Programming Important?
Competitive programming is important as it helps to deepen your concept and help to boost your confidence level. The competitive programming contests keep your preparation in check and help you analyze your preparation level. Also, let us not forget the crazy amount of prizes and opportunities it offers. Candidates can find some of the coding competitions happening in 2024.
Top Competitive Coding Questions 2024
Let us now analyze some important coding questions in the article below.
Q1. What is an array?
Ans: An array is a data structure that stores a collection of elements using an index. The elements stored inside the array are contiguous and are the same type of elements.
Array in Data Structures |
# Creating an array
my_array = [1, 2, 3, 4, 5] (integer type elements stored in my_array.) # Accessing elements first_element = my_array[0] #Output 1 second_element = my_array[1] # Output 2 |
Q2. What is the importance of Data structures in Programming?
Ans: Data structures play a major role in programming. Let us know some of the important reasons.
- It is used or organise data and manage it efficiently.
- With the help of efficient data structures like linked lists, hash, trees and graphs, it is easy to store, insert, and manipulate data while programming.
- Efficient use of resources.
- It helps with problem-solving in computer science programming.
- It helps to provide optimal solutions for real-world applications.
Learn more about Data structures in Python.
Q3. What is sorting and which is the best sorting algorithm?
Ans: Sorting is arranging data elements in a regular order (ascending or descending). Data structure has many sorting algorithms, such as bubble sort algorithm, quicksort, selection, merge, and more.
Quick sort is the best sorting algorithm and most efficient algorithm. It uses the divide and conquer approach to sort the data based on pivot element. Learn more about the Quick Sort Algorithm.
Q4. What is a Stack data structure?
Ans: The stack data structure is a linear data structure used to perform operations in LIFO (Last in First Out) order. The elements in a stack can be accessed from the top. In FIFO we can access, store, and retrieve data.
Q5. What is Recursion?
Ans: Recursion is a process in which a function calls itself directly or indirectly. Recursive function must have a base condition. Learn more about Recursion in data structure and its uses.
Q6. What is Kadane’s algorithm?
Ans: With the help of Kadane’s algorithm we can find the maximum subarray sum in a given array of integers. The algorithm have a time complexity of O(N).
Kadane’s algorithm |
|
Q7. How do Reverse words in a string?
Ans: Check the C++ code to reverse string.
Reverse in String |
string reverseWords(string S)
{ string temp=””; string ans=””; for(int i=S.length()-1;i>=0;i–){ if(S[i]==’.’){ reverse(temp.begin(),temp.end()); ans=ans+temp; ans.push_back(‘.’); temp=””; } else{ temp.push_back(S[i]); } } reverse(temp.begin(),temp.end()); ans=ans+temp; return ans; } |
Q8. Write a code to find the longest palindrome in a string. You are given a string S[ i . . . . j ] where 0 ≤ i ≤ j < len(S).
Ans: The string S is a palindrome if the reverse of the S is = S. Let us check the code in the table below.
Longest Palindrome in a String |
string longestPalin (string S) {
// code here int n=S.size(); int start=0,end=0,maxl=1; //odd length for(int i=0;i<n;i++) { int l=i,r=i; while(l>=0 && r<n) { if(S[l]!=S[r]) { break; } l–; r++; } int len=r-l-1; if(len>maxl) { maxl=len; start=l+1; end=r-1; } }
//even length for(int i=0;i<n;i++) { int l=i,r=i+1; while(l>=0 && r<n) { if(S[l]!=S[r]) { break; } l–; r++; } int len=r-l-1; if(len>maxl) { maxl=len; start=l+1; end=r-1; } } return S.substr(start,maxl); } |
Q9. Difference between Linear and Non Linear Data Structure.
Ans: Let us find the major difference between linear and non linear data in the table below.
Competitive coding questions | |
Linear data structure | Non-linear data structure |
It is a structure in which data elements are adjacent to each other | It is a structure in which each data element can connect to over two adjacent data elements |
Examples of linear data structure include linked lists, arrays, queues, and stacks | Examples of nonlinear data structure include graphs and trees |
Q10. What is the difference between stack and Array?
Ans: Stacks and array are two different data structures. Let us know some major difference between them in the given table.
Difference between Stack and Array | |
A stack is a linear data structure which follows LIFO (Last In, First Out) | An array is a linear data structure that collects the data as index. |
The element can be accessed from the top of stack. | The element can be accessed from anywhere using the index. |
The primary operation on stack are push, pop, peek and others. | In array we can read, write, modify elements at any specific index inside array. |
Stack can be implemented using array, linked list and other data structures. | Array is a fundamental data structure and can help to implement other data structures. |
Q11. What are dynamic data structures?
Ans: A dynamic data structure can adjust themselves at run time. It is a flexible method of manipulating and organizing data.
Q12. Write a program to check if a string is a palindrome?
Ans:
Competitive coding Questions |
if (str.equals(reverse)) {
System.out.println(“Palindrome”); } else { System.out.println(“Not Palindrome”); } |
Q13. How to implement selection sort algorithm?
Ans: Check the table below to implement selection sort algorithm.
Selection Sort Algorithm |
#include <stdio.h>
void selection_sort(int arr[], int n) { int i, j, min_index; // Traverse through all array elements for (i = 0; i < n – 1; i++) { // Find the minimum element in the unsorted part of the array min_index = i; for (j = i + 1; j < n; j++) { if (arr[j] < arr[min_index]) { min_index = j; } } // Swap the found minimum element with the first element int temp = arr[i]; arr[i] = arr[min_index]; arr[min_index] = temp; } } int main() { int my_array[] = {64, 25, 12, 22, 11}; int n = sizeof(my_array) / sizeof(my_array[0]); // Perform selection sort selection_sort(my_array, n); // Print the sorted array printf(“Sorted array: “); for (int i = 0; i < n; i++) { printf(“%d “, my_array[i]); } printf(“\n”); return 0; } |
Q14. Write a code to implement quick sort algorithm.
Ans:
Quick sort algorithm |
#include <bits/stdc++.h>
using namespace std; // A utility function to swap two elements void swap(int* a, int* b) { int t = *a; *a = *b; *b = t; } int partition (int arr[], int l, int h) { int pivot = arr[h]; // pivot int i = (l – 1); // Index of smaller element and indicates the right position of pivot found so far for (int k = l; k <= h – 1; k++) { // When the actual element is less than the pivot if (arr[k] < pivot) { i++; // increment index of smaller element swap(&arr[i], &arr[k]); } } swap(&arr[i + 1], &arr[h]); return (i + 1); } //A function to implement quicksort void quickSort(int arr[], int l, int h) { if (l < h) { //pi is a partitioning index, and //arr[p] is now in the correct location. int pi = partition(arr, l, h); // Separately sort elements before // partition and after partition quickSort(arr, l, pi – 1); quickSort(arr, pi + 1, h); } } /* Function to print an array */ void print_array(int arr[], int size) { int i; for (i = 0; i < size; i++) cout << arr[i] << ” “; cout << endl; } int main() { int arr[] = {11, 13, 16, 1, 3, 5, 9}; int n = sizeof(arr) / sizeof(arr[0]); quickSort(arr, 0, n – 1); cout << “Sorted array: \n”; printArray(arr, n); return 0; } |
Q15. Write a code to reverse an array.
Ans:
We will traverse our array until the half length of the array. Now, replace the number at the given index with the given code. Here, we have an array a[] consisting of some integer elements.
Reverse an Array |
for (int t = 0; t < a.length / 2; t++) {
int tmp = a[t]; a[t] = a[a.length – t – 1]; a[a.length – t- 1] = tmp; } |
Competitive Coding Questions FAQs
Is coding important for a technical interview?
Yes, coding is important in technical interviews. Candidates must have knowledge of one of the programming languages, data structures, or some advanced technologies.
Is a linked list better than an array?
Yes, linked lists follow not contiguous memory allocation, where any element can be stored or connected using pointers. It is more efficient than arrays.
What is a graph data structure?
Graph is a data structure that contains ordered pairs of elements. The graph contains nodes, edges and vertices which is used to connect each other and store and retrieve the data.