Python is used by many of us and is one of the most preferred languages for programmers worldwide working in various domains. Python developers are always in demand by big tech companies like IBM, Netflix, NASA, Pixar, JP Morgan, Spotify, and many more. Python is considered beginner-friendly and easy to learn because of the advanced standard library support, community, and syntaxes.
We have prepared a set of top python interview questions in this article. The article covers some major important questions that are mostly asked during the interview round.
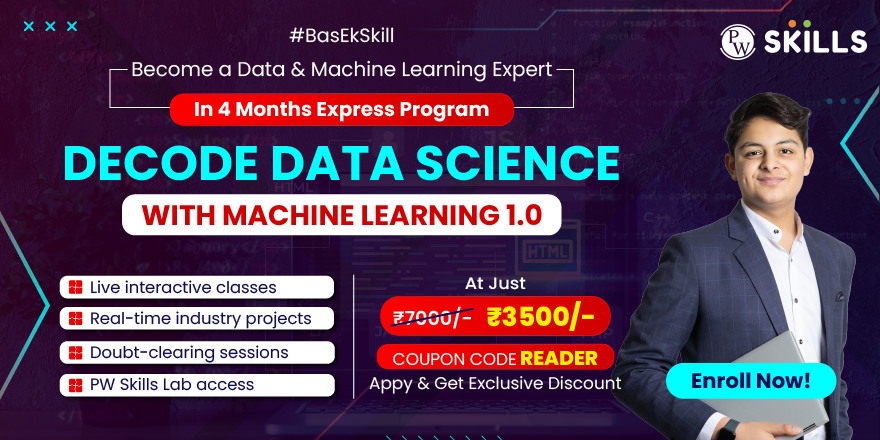
Top Python Interview Questions
Q1. What is the Python programming language?
Ans: Python is a high-level programming language which is used for various purposes, such as web development, machine learning algorithms, Artificial intelligence, Data science, software development , data analyst and many others. It was created by Guido Van Rossum in 1991.
Q2. Why is the Python program easy to use?
Ans: Python programs consist of easy syntax, library support, modules, and a lot of community support, which makes learning Python an easy task out of all the other languages.
Q3. What are some important features of Python language?
Ans: Some major features of Python language are given below:
- Object-Oriented Language
- High-Level Language
- Dynamically Typed language
- Extensive support Libraries
- Presence of third-party modules
- Open source and community development
- Portable and Interactive
- Portable across Operating systems
Q4. What is the use of the # symbol in Python?
Ans: The hashtag (#) symbol is used to comment between Python codes in a Python environment.
Q5. What is the difference between a Set and a dictionary?
Ans: The difference between a set and a dictionary is given in the table.
Python Interview Questions | |
Set | Dictionary |
A Set is an unordered collection of data. | Dictionary in Python stores data in the form of key-value pairs. |
Elements are accessed by iterating over the set. | Elements are accessed using keys. |
It contains only unique elements. | It contains duplicate elements. |
Q6. What is lambda function?
Ans: The lambda function is an anonymous function which supports only one statement but with any number of parameters.
Python Interview Questions | |
x = lamda a, b : a*b
print (x(10,11)) |
Q7. What is the advantage of the Numpy array?
Ans: Numpy arrays are faster and more efficient. It is stored in the form of modules, which we can import anytime in our code. Numpy is implemented in C and allows more storage, making it more memory efficient than Python lists.
Q8. What is the use of the// operator in Python?
Ans: In Python, //performs divisions and returns the quotient as output.
Q9. How to check whether a string contains alphanumeric letters or not?
Ans: We can check whether a string contains an alphanumeric in python using the inbuilt method isalnum().
Python Interview Questions | |
>> “abcd123”.isalnum()
Output: True >>”abcd@123#”.isalnum() Output: False Another way is to use regex as shown. >>import re >>bool(re.match(‘[A-Za-z0-9]+$’,’abcd123’)) Output: True >> bool(re.match(‘[A-Za-z0-9]+$’,’abcd@123’)) Output: False |
Q10. What are some major advantages of using Python?
Ans: Some major advantages of using Python are given below.
- Python is an object-oriented, high-level, interpreted language with dynamic semantics.
- Python is compatible with many platforms, including Linux, Mac, Windows, and Raspberry Pi.
- Python has straightforward punctuation when contrasted with different dialects.
- Python allows programmers to write programs with fewer lines of code than other programming languages.
- Python operates on an interpreter system, so code can be run immediately after it is written. It is beneficial to deliver a prototype promptly.
- One could characterise Python as taking a procedural, item-oriented, or utilitarian approach.
- The Python interpreter and the large standard library are freely distributable in binary or source form for all major platforms.
Q11. Name three types of numerical literals supported by Python.
Ans: There are mainly three types of numerical literal, integer, float and complex literal. Check the examples below.
Python Interview Questions | |
|
Q12. How can we pass the Python parameter into our code?
Ans: Python parameters can be passed using references and values.
Q13. What is overloading in Python?
Ans: In Python, we can define multiple functions with the same name having different parameter types or the number of parameters.
Python Interview Questions | |
def add_numbers(a, b=0, c=0):
return a + b + c result1 = add_numbers(1) result2 = add_numbers(1, 2) result3 = add_numbers(1, 2, 3) print(result1, result2, result3) # Output: 1 3 6 |
Q14. What is the difference between a remove () and a del statement?
Ans: The remove function is used to delete a specific object present in the list. However, with the help of del, we can delete an object at a specific index in the list. We use the remove function directly to delete an object, while del deletes the element present at a particular index.
Python Interview Questions | |
list1 = [3,5,2,9,1]
print(list1) list1.remove(3) print (list1) |
Output:
Output | |
[3, 5, 2, 9, 1 ]
[ 5, 2, 9, 1 ] |
For del() method:
Check the implementation of the del method using Python.
Python Interview Questions | |
list1 = [3,5,2,9,1]
print(list1) Del list1[0] print (list1) |
Output:
[3, 5, 2, 9, 1 ]
[ 5, 2, 9, 1 ] |
Q15: What does Python’s scope mean?
Ans: A variable’s scope is where we can locate it and access it when needed.
- Python local variable: Local variables are specific to a function and are initialized inside the function. It is not accessible from outside the function.
- Python global variables: These are variables that are declared and defined outside of functions and are not used by any specific functions.
- Module-level scope: This describes the program’s current module’s global objects that are accessible.
- Outermost scope: Any built-in names the application can call are included. The name that is being referred to is the last item in this scope.
Q16. Differentiate between the append() and extend() function.
Ans: Append is used to add elements to the end of a list in Python. Extend is used to add elements from an iterable to the end of a list.
Q17. What is a docstring in Python?
Ans: Docstrings in Python are used to provide various Python modules, classes, and methods.
Python Interview Questions | |
def add_numbers(a, b):
“”” This function takes two numbers as input and returns their sum. Parameters: – a (int): The first number. – b (int): The second number. Returns: int: The sum of the two input numbers. “”” return a + b # Accessing the docstring print(add_numbers.__doc__) |
Q18. Differentiate between list and tuple.
Ans: Some major difference between list and tuple is given below.
Python Interview Questions | |
Lists | Tuples |
Mutable (can be modified) | Immutable (cannot be modified after creation) |
Generally more | Generally less |
Better for these operations | Immutability makes these operations impossible. |
Time-consuming | Faster iterations |
Can access elements | Can access elements |
Q19. How to debug a Python program?
Ans: You can easily debug your Python code using the following command.
Python Interview Questions | |
$ python -m pdb python -script.py |
Q20. What is polymorphism in Python?
Ans: The ability to assume different forms is known as polymorphism. Therefore, if the parent class has a method called ABC, for example, the child class may also have a method with the same name but with different parameters and variables.
Q21. What is encapsulation in Python?
Ans: Encapsulation in python binds the code and data together. It is used to encapsulate the process inside and only present the interface to the user.
Q22. How to delete a file in Python?
Ans: We can delete a file in Python using os.remove(), or os.unlink().
Q23. What is a namespace in Python?
Ans: A namespace in Python is used to give unique names to variables to avoid any confusion in naming.